For loop in JavaScript
Hello there,
I am creating a simple webpage for a Caesar Cipher.
I wrote the Cipher in Object-Oriented.
Something is wrong with a method though. Im not exactly sure but when I shift anything other than A, the encryption is wrong.
As you can see in the image.
B
plus 1
shouldnt be L
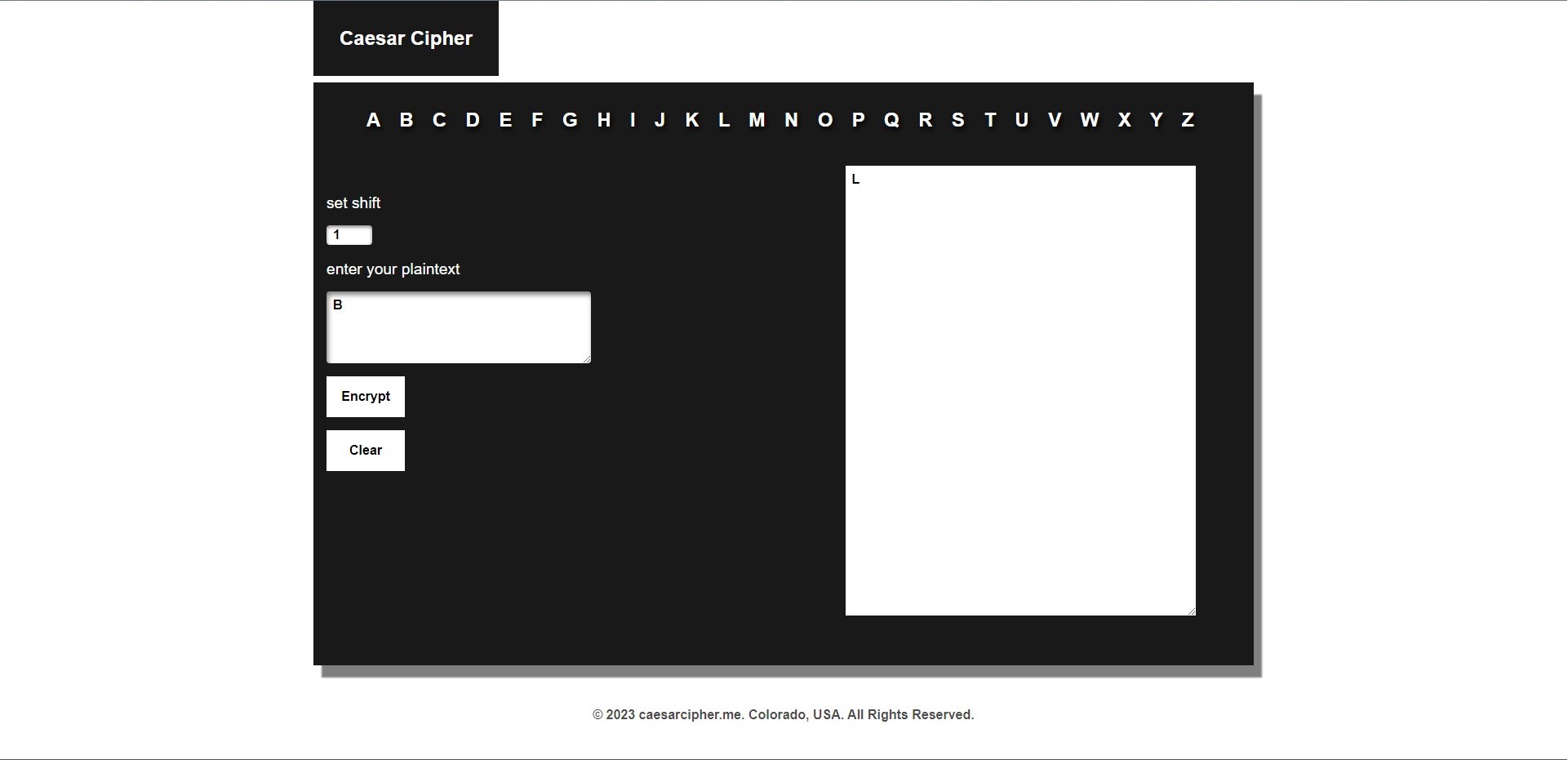
17 Replies
You're calling
this.plain_text
,this.alphabet
, and this.shift
without showing what those are so we can't really help much. Can you provide the whole class/function that you're using?Off the top of my head, I'm guessing the issue is with you setting the shift value. All inputs in HTML are read as strings by JS, so even if the HTML says the shift value is a number input with a value of 4 JS reads it as the string
"4"
. And that can cause problems if you're trying to add a string (as that will concatenate the values instead of adding them).ahh 🤔 i didnt get any errors tho
@cvanilla13eck how do you cast from string to int in js?
parseInt({str}, 10)
(10 being the radix; you'd use 2
for binary and 16
for hex)
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseIntExcellent!
it works
WOO!
😆
thanks so much
Just because I could, here's another way of doing the
encrypt
function by turning the string into an array and using the .map()
functionality to modify the letter, then using .join()
to make it into a string again:
This way you don't need to store the text length and don't need to do a for
loopahh i see
awesome
I'm not a fan of the old
for
loop, so I prefer to use forEach()
, .map()
, and .filter()
as it handles the minutia of index trackingyeah, im new to js
im comming from python
js has a shit ton of methods
If you prefer a more imperative-style, here's one using the
for...of
loop:
This snippet has more lines of code as you're manually doing what the .map()
method does under the hood, but it helps new programmers to see how it works
you have no idea 😜
@jj_roby_1993 added comments to the above code to help you understand what's happening@cvanilla13eck Yo for some reason when I try to take encrypted text and use the negative of the shift number, I get undefined as a result.
Shouldn't it still work and is essentially adding a negative number to the location (or subtracting) then modulo 26?
If you're trying to reverse it you can't use the
%
operator alone as it'll still be a negative number. After you do the %
operator you need to check if it's a negative number and if so, subtract that number from 26. That should get you the correct letterThat worked, thanks for that. I have an other issue if you dont mind...
I'm trying to set up media queries. I got it re-adjusted for a tablet, but when I do a phone size,
height: 100vh;
is not working properly.Please make a new post for a new question