getById from current user
Hi, its my first time using tRCP and I would like to ask how I can get something by id from the current user.
23 Replies
your where is wrong
you also need to add userId: ctx.session.user.id
currently he gets the deposit from a specific id, if you want to get from a specific id and userID ( in this case the one logged in ) you need to add ctx.session.user.id
but you actually dont even need to use the current user, if the id is unique, you just query it, dont need to specify a user, since it already belongs to that user only
OK, but how would I do it with
ctx.session.user.id
?
Because this is giving me an error:
do you have userID on your schema?
on the deposit model
yes it works for the getAll
but as I said,
getAll
works fine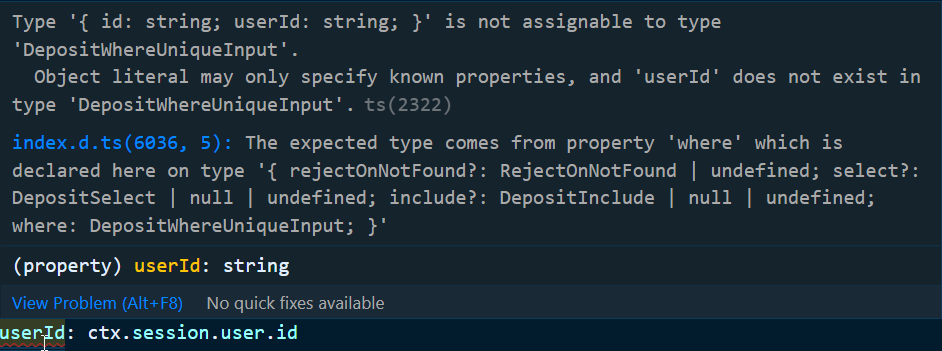
try this, where: {
AND: [
{ id: input.id },
{ userId: ctx.session.user.id },
],
},
now AND is the problem:
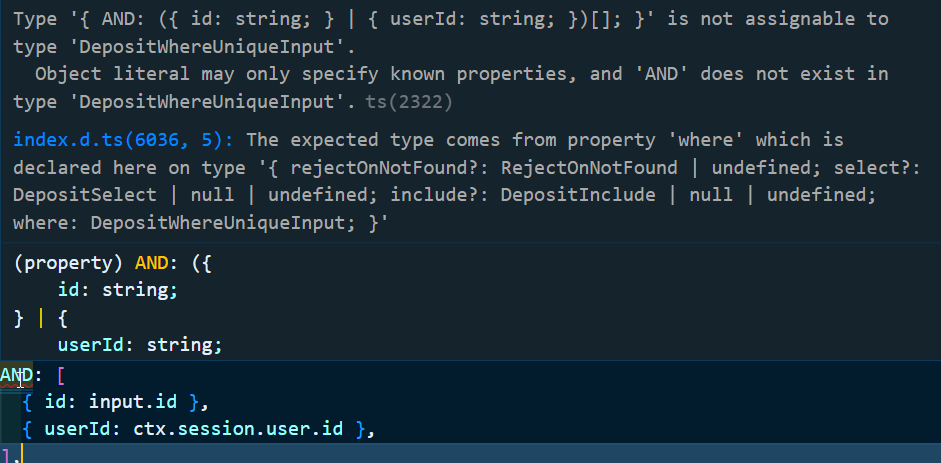
ohhh wait i get it now
its the findunnique
it only accepts 1 search paramter
so it will never let you use more than 1
ohh, but this btw. is also not working:
maybe because it is not nnique
yeah, bc there is more than 1 deposit
and findUnique purpose it to find only 1
since there are multiple deposits with the same userID it will not work
do you think this would do?
but if you use the deposit id it will work everytime
it is not giving me an error
you can try
but i dont think it will
i suggest this simply doing this:
getById: protectedProcedure
.input(z.object({ id: z.string() }))
.query(async ({ ctx, input }) => {
const deposit = await ctx.prisma.deposit.findUnique({
where: {
id: input.id,
},
});
return deposit;
}),
it will always get the one specifiedYea, but theoretical if you have the ID you can get the deposit from a another user right?
no, bc you are using the deposits ID that ID is unique to that deposit
and that deposit is associated to 1 user only
alright, thanks for your help @Diogovski
you're welcome
OK, I checked it and it do is like I thaght:
server:
client:
I'm logged in as user 1 but the deposit.id is of a deposit from another user.
I think I will just go with this, if you have any better ideas please tell me:
but thats bc you let the other user know the deposit id from a different user
which you shouldnt do
yes ofc, but it feels a but sloppy,
just because I know the id of a privat massage of yours I don't think I could request it.
---------------------- CLOSE ----------------------
https://discord.com/channels/966627436387266600/1093273688557768924/1093273688557768924