Keeping getting the push ERROR
I keep getting this ERROR in the console everytime I call this function as you can see in the image. Why is this happening and how can I solve it? my intention with code was to create a new list of array everytime I change the const data and not to substitute the before alteration with a new one like the code is doing
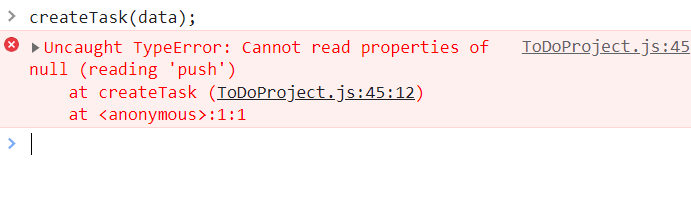
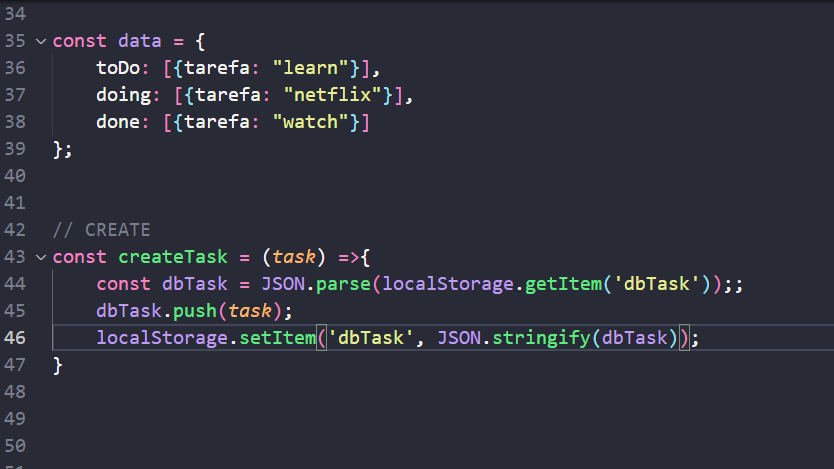
45 Replies
That’s because you’re trying to use
.push()
on an object. .push()
is an array method, no an object method.I must to convert using the Object.keys ? I try to use this method but it didn't work 😦
Also, for the future, please provide actual code and not screenshots. You can’t copy/paste from a screenshot
Sorry, I will send the code
Assuming
dbTask
follows the same setup as data
you need to get the correct task type first, then push the new team to the correct arrayCan you show me,pls?
dbTask.toDo.push(task)
will add the new task to the to-do array list
If I want to take all the three (Todo, doing and done) together?
What I would change:
The
category
parameter is added to dynamically choose which array to add to. I used bracket notation to select the key in the object to access
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Working_with_Objects#accessing_propertiesIf I want to modify in the data?
Like add task in the data variable
If you want to use the
data
variable then you should access that instead of local storageIt still get an error 😔
The error might be because you're trying to access something that doesn't exist. The first thing you do is grab the
dbTask
string from local storage, but if you didn't put anything there then it's not an object with arrays, it's an empty stringWhen I console log the dbTask, it shows that it is a null value
It may be because of this
My suggestions is to use the
data
variable you declared for all reads and only write to local storageCan you show me again? I only understand properly when I see an example
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Nullish_coalescing
https://developer.mozilla.org/en-US/docs/Glossary/IIFE
These are more advanced techniques, though. Not something you learn in JS 101
Thanks so much!!
Like I said in the other thread, to do lists are not the simple app most people think :p
Yeah, but I put in my head that I will make it anyway
In this way, I can learn more
It's a great learning experience, for sure
Another option that doesn't use an IIFE:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/assign
I think this second way is beter
Yeah, it's better 🙂
In JavaScript there are at least three different ways to do the same thing 🤣
How long did it takes to you to learn all this things?
That's the fun part 😅
I've been learning JS since 2018. So I'm going on 5 years
Be sure to subscribe to https://gomakethings.com/articles/
Daily Developer Tips
Hate the complexity of modern front‑end web development? I send out a short email each weekday on how to build a simpler, more resilient web. Join over 14k others.
If you're human, leave this blank Enter your email address to get Daily Developer Tips emails Get Daily Developer Tips ⏰ Last Chance! A new session of the Vanilla JS Academy sta...
Chris is an amazing teacher of all things vanilla JS!
MDN is a great resource if you know what you're looking for. MDN is more for reference, "how does Array.map() work again?" than it is for learning IMO
Thanks
But MDN does have a "Learn JS" section if you want to have a look
https://developer.mozilla.org/en-US/docs/Learn/JavaScript
it continues to show that ERROR message
I don't know what is happening
What's your code look like?
My whole code?
The code that's not working
I will send you. Just a second
And what error are you getting?
Cannot read properties of undefined (reading "push")
How are you creating your task? If you don't add the correct category it'll error out
I am creating in the data
Also, you don't need
const dbTask = JSON.parse(localStorage.getItem('dbTask'));
. You should have that in the const data
above:
Otherwise as you have it you're only creating the default object without applying the local storage items to it
No, I mean what does the function call look like to create the task?
if category
doesn't exactly equal toDo
, doing
, or done
then the function will error as nothing else is defined in data
(and undefined
doesn't have a .push()
method)
As an example, if you wanted to add "cut the grass" to your doing
list:
I am doing this way
In the "doing", I could use data.doing?
No, you can't
if
category
doesn't exactly equal toDo
, doing
, or done
then the function will error as nothing else is defined in data
(and undefined
doesn't have a .push()
method)
In the createTask
function data[category]
is what is telling the code where to push the task. When you pass "doing" to the function, it becomes data["doing"].push(...)
I get it
Thanks
I will give up this project for a while, because it so frustrating
So
createTask("cut the grass", "doing");
: