tRPC Mutation
Hi, I don't quite understand how to pass the input when using with
useMutation
Server:
Client:
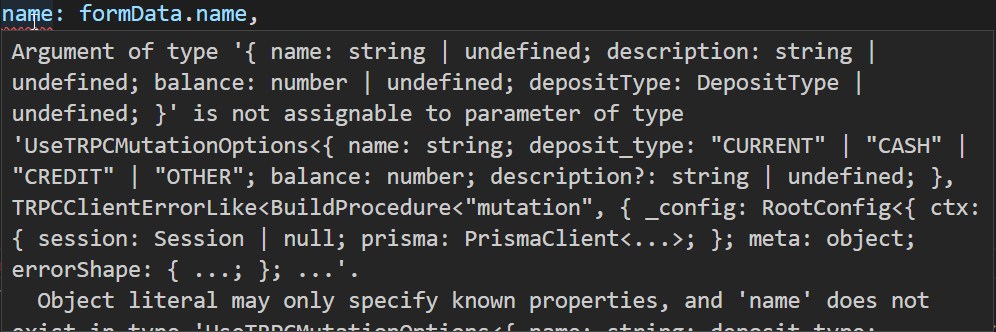
Solution:Jump to solution
api.deposit.create.useMutation is a hook so you cant use it like that
it expose a
mutate
function, so you can use it to actually send the data to the server, something like
```ts
const Deposit = () => {...5 Replies
Solution
api.deposit.create.useMutation is a hook so you cant use it like that
it expose a
mutate
function, so you can use it to actually send the data to the server, something like
Ahh, thanks
Thanks, @corn flour that really wasn't clear to me. I was reading two sets of documentation for React Query and TRPC couldn't join the dots until reading your answer. Perhaps the T3 docs should be updated with description of mutations as well.
The doc page for tRPC on ct3a does have a mutation example here
https://create.t3.gg/en/usage/trpc#inferring-errors
but perhaps having an actual section on mutation similar to query in that page might be a bit clearer for beginners?
i personally find the trpc doc for mutation pretty decently clear though; I didn't have trouble trying to figure that out: https://trpc.io/docs/reactjs/usemutation
useMutation() | tRPC
The hooks provided by @trpc/react-query are a thin wrapper around @tanstack/react-query. For in-depth information about options and usage patterns, refer to their docs on mutations.
also no calling hooks inside other functions 😄