Error: ENOTDIR: not a directory, scandir 'C:\Users\k_der\developing\JS\bots\commands\ping.js'
Hi, so i'm making my first bot in JS. Now i want to deploy the slash commands inside the DIR
commands
. But it gives the error "Error: ENOTDIR: not a directory, scandir 'C:\Users\k_der\developing\JS\bots\commands\ping.js'" When i'm running deploy-commands.js
.
Who can help me?
Script i use:
22 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.discord.js@14.9.0
Node v18.16.0
Full stack trace:
You tried to call readdir on commands/ping.js, which is a file, not a directory
That part i get
But why does the official code do that? I got the
deploy-commands.js
from: https://discordjs.guide/creating-your-bot/command-deployment.html#global-commandsdiscord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
Or need to be a folder inside the commands dir?
The official guide doesn’t put commands into categories like that
Or ig it does 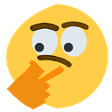
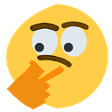
That's why i'm a lil bit confused
If you don’t want that, you can just reduce it to a single readdir
Remove the const commands and for (const folder of …) and define
const folder = "<the commands folder>"
I have also
const commandsPath = path.join(foldersPath, folder);
I think i can remove that also right?
Because i dont need to join aditional foldersDefine the commands folder path there instead
Remove the const folder
That should work
Hmm okey!
I'm now getting another error "missing access"
But i have enabled the "application.commands" scope and reinvited the Discord bot
Do you know what i can check more?
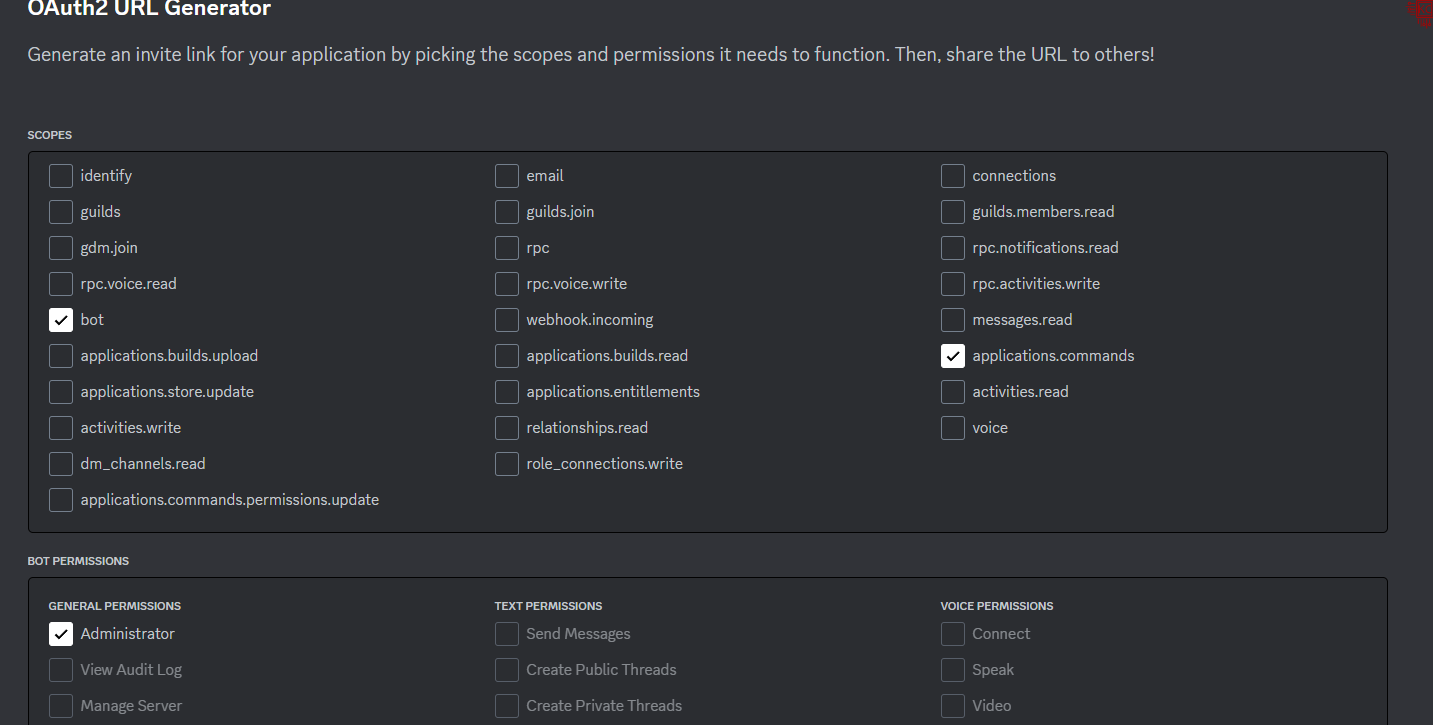
Use the url that was generated and authorize the bot to the guild
I did do that xd
Show bot in the integrations tab of the guild
I did kicked bot, copy this url in the browser

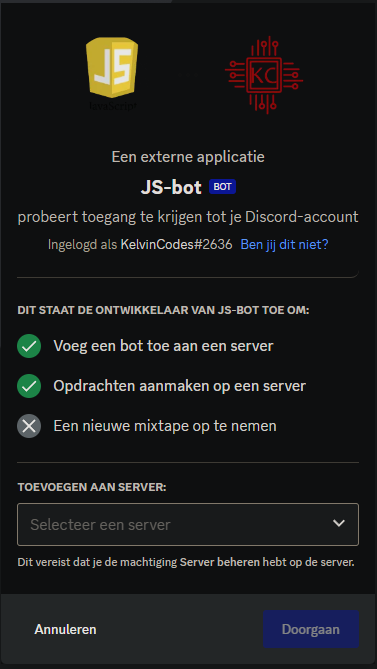
Is the guild id correct?
Good one, gonna check
It’s not common to have a guild id that starts w/ 00
Uhhm no 🥺 whoops
That was the issue!
Thank you so mutch! I did make that he goed trough every file tho, for ez use:
I got:
Started refreshing 3 application (/) commands.
Successfully reloaded 3 application (/) commands.
Jeeej
You're a hero for your help!