How can I fetch data in my component
Here is the link to stackoverflow.com displaying how I implemented it
https://stackoverflow.com/q/76112994/19684000
Stack Overflow
Rendering fetched data from supabase in Nextjs13 App Directory
I successfully fetched data from supabase but I am unable to render them in my component , I tried implemented a component to hold the fetched data but i was unable to as it arose to other errors t...
79 Replies
Im not familiar with supabase, but the general way of calling your endpoint, wait for a response, and set the result to a state variable in your component looks right. You should probably add am empty dependency array to your useEffect though. Apart from that a bit more information on what's going wrong in your example would help solving the cause.
Also you're not rendering the "profiles" state in your component yet
I didn't include rendering because no matter how I tried to render I got errors
Hey, are you using t3 stack? if so it's better to make it with prisma
seems like what you're trying to do is to fetch users data.
I suggest you to use following provider and use User hook:
then you can just use it as
but that's from supabse auth.user
if you want to fetch data of users profile you would have to set up supabse user management (check our sql editor and default templates)
and then fetch from public.profile (or profiles I don't remember)
check out how I'm using it, here's an t3 + supabse app I built like a week ago:
https://github.com/F-PTS/FriendlyMacros
maybe it would help you
GitHub
GitHub - F-PTS/FriendlyMacros
Contribute to F-PTS/FriendlyMacros development by creating an account on GitHub.
there's a bit of antipatterns in code you've put on stack overflow (e.g. useEffect without dependency array, using any types, etc.) I think FriendlyMacros repo would help you understand what good practices in react, next, prisma, trpc and supabase are
i am using supabase with nextjs without prisma
thanks bruh but this one i used nextjs 13.3.1 App Directory and Supabase
i included codes where i am performing rendering
i successfully fetch them but I am unable to render them
yeah same, i just used trpc and prisma as well
but shouldnt be any trouble
since its just a context provider
how come?
as i console log the data of a specific user i saw them but rendering it throws an error
i added Profile.tsx component in stackocerflow
you used new App Directory?
yes
what was the error?
let me screenshot it now
also, why are you using React.FC<ProfileProps> ? the default way of making functional component would be just fine I think
i shifted from prisma as i was only able to migrate model into the database but i was unable to perform user registration that's why i decided to shift to pure supabase as i always do when i am using vue 3
which one
Profile.tsx
What would the point of React.FC
and not
this is the error i am facing
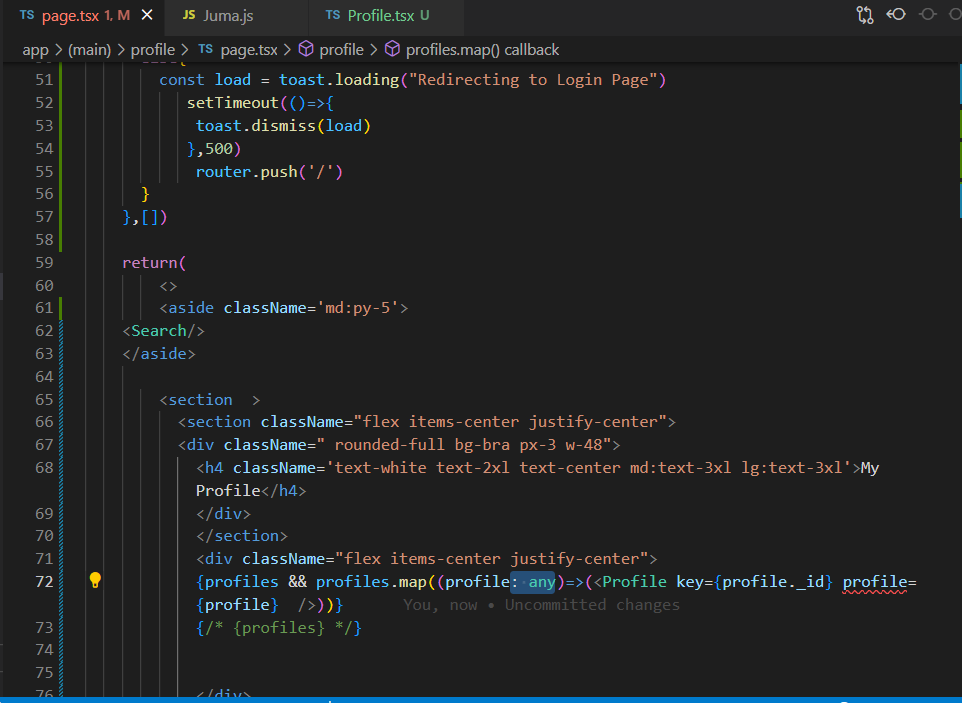
yeah great but let me see the error message
hover over it
oh yeah i think I know what are doing
this one throws some error
you specified in Profile.tsx that profile has to be type of ProfileProps. Meanwhile you are passing any into it
let me see an error message
this one
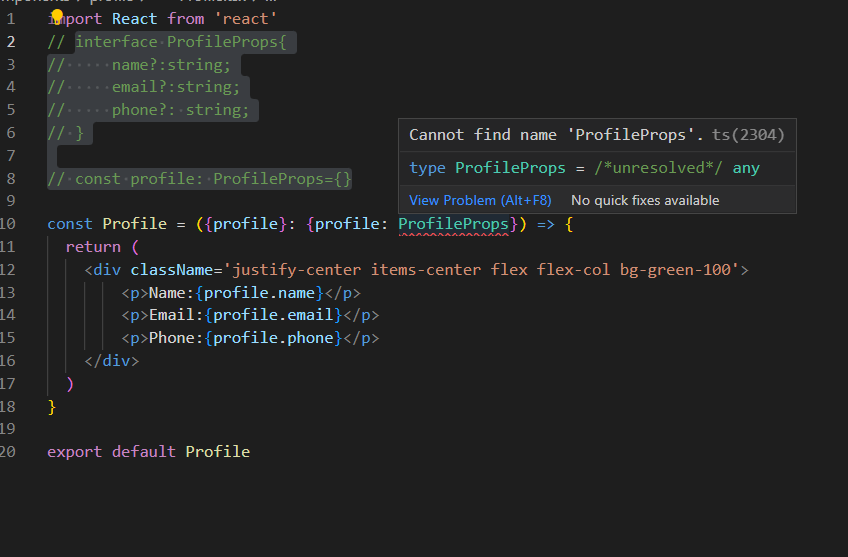
should i pass a string?
well no wonder it cannot find ProfileProps since you commented it out. Remove comments from the interface
and delete 8th line
done then should i try to see if the data can be displayed?
do you have a way of getting a type of your profile in your page.tsx?
thanks bruh they are retrieved successfully
because if you do, you should change :any type to the type of your response
but do not import the type from @prisma/client. You shouldn't mix backend and front end types (not a good practice)
i was able bruh thanks to you now i will be thinking only about implementing middleware
i am using pure nextjs and supabase bruh
yeah sure, also keep in mind that you should almost never (unless in app directory) use export default. Use export instead
oh right i brain farted lol
supabase could generate your types tho
i didn't generate types i just gave it a thought as supabase-js supports types what if i could utilize it without generating types using supabase cli
uhh I'm not sure which way would be the best to be honest since I don't know much about supabse-js
oh it's okay bruh but i am really thankful with your help as i was able to get through this nightmare
sure, no problem
any idea as how i can implement middleware bruh
the auth middleware?
of course
as i want to protect routes
You could do a HigherOrderComponent and use my AuthContext
then how can i attach it to my app directory
and then just do
on every page that user would have to be signed in to access the page
then inside the HOC you can use the useUser hook and redirect if there's no user or session (meaning thay user is logged off / user does not exist)
a little tip:
in app folder create (grantedAccess) folder, or (app), anything. doesnt matter but keep the naming accurate
and in that folder with () around the name add layout.tsx file. and there you can use withAuthContxt HigherOrderComponent
let me show you my app folder structure
inside (main) i created layout.tsx
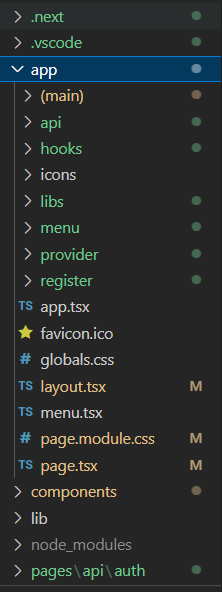
yeah and now just move every page you'd want to be accessed only by signed in users
you dont have to specify the name of (main) in routes, next automatically ignores it
my layout.tsx
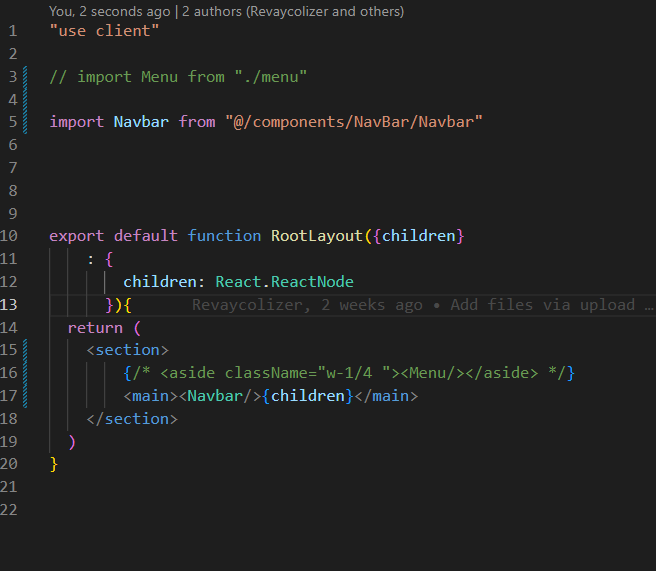
app
- (main)
-- page.tsx
is NOT /(main)/
it is simply /
(the default route)
is how i moved the files correctly?
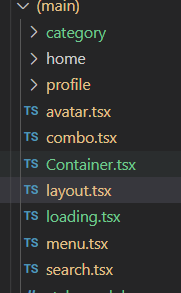
Make sure you didn't require to be signed in, in order to sign up or sign in
other than that it should be fine
should i use this one?
the components to sign in the user are not in (main) folder
yes
good
what about the component i included in the layout.tsx
yeah seems good, but make sure it renders as a column and not as a row
so navbar is on top
and not on the side
yes bruh it is on the top but wrapping it requires adding "use client" is it a good practice a middleware having "use client"?
did you create it in app folder?
in (main) folder
here menu.tsx
move it to src/utils
menu.tsx?
it's an server utility, not client
the higher order component
middleware???
yes, the middleware
what about the layout i just created?
as i get confused bruh
at the end of layout.tsx in (main) add:
is this the middleware or layout?
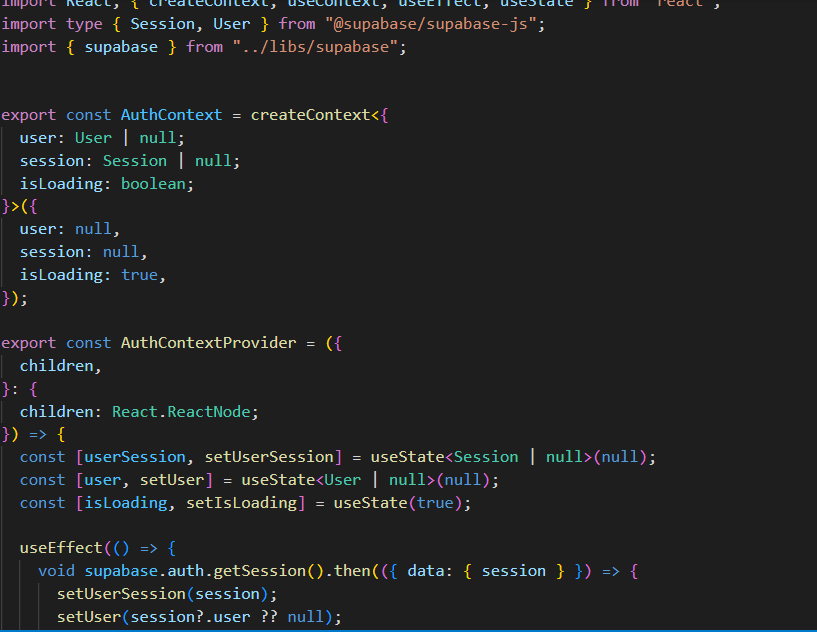
this is the middleware
oh my bad i replaced layout.tsx with it
lol
getting AuthContext.Provider error
solved as i first saved it as middleware.ts instead of middleware.tsx
good
now getting this error in layout.tsx
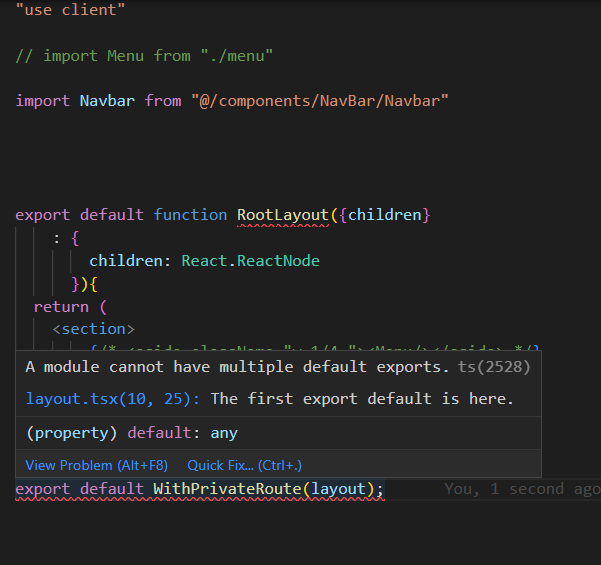
yeah so if you actually read it you could solve it
i was wondering as i should remove children
think
or am i making a big mistake?
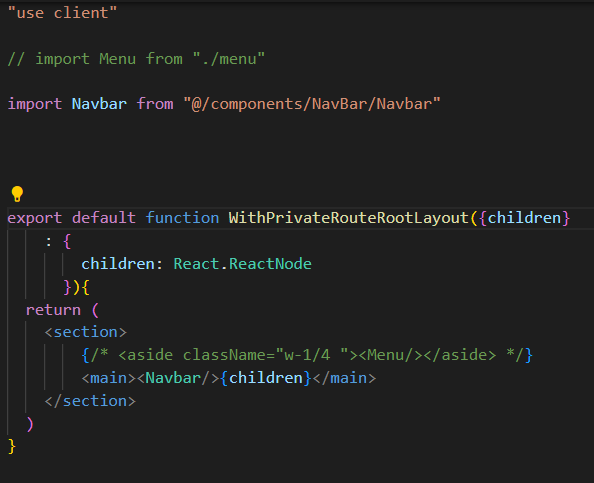
yes, you are
then should i remove RootLayout?
here
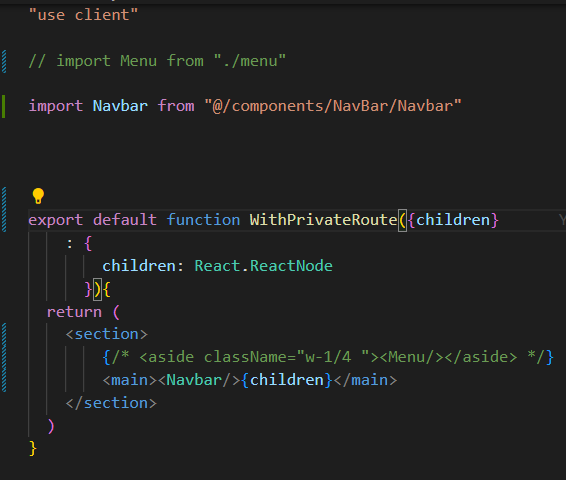
think
what are you trying to achieve
protecting pages rendered in this route
yeah and how would you do it
by using middleware to restrict unauthenticated user
and how do you use middelware?
look at the code of WithPrivateRoute
let me check it again
this PrivateRoute where do i include it
am i right?
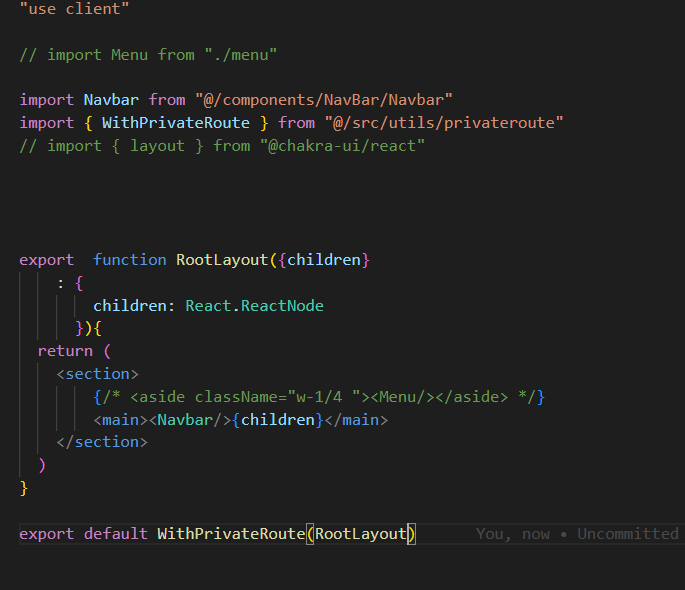
tried but getting only a spinner even if user is authenticated