How do I set the default useState value of a trpc call?
EDIT: Sorry apparently I can't rename the title. I meant to say: 'How do I set a default useState value to something that came from a trpc call'
Hi folks, so I need some help with my component
It's a simple team switcher. I need help understanding a few things. I have two main trpc endpoints:
getAllForLoggedUser
and switchActiveWorkspace
.
one thing: whenever I am reloading this component fresh new, I am not being able to set the default value of the useState call. The default value is not being set. How can I make it so it sets the default value that came from the trpc call
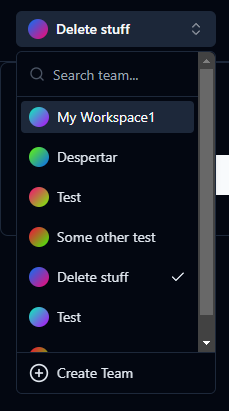
7 Replies
Here's the full code if needed
put the useState above the queries
onSuccess of the query, update the state
@Neto But this way,
workspace
(where the useState is invoked) will be used before it's being defined.
OOh I see what you meanif the state is derived from the query
you can make a "blank" state and update when the query finishes
This fixed it!! Thank you so much ❤️
while this does work its probably not ideal: https://tkdodo.eu/blog/breaking-react-querys-api-on-purpose
Breaking React Query's API on purpose
Why good API design matters, even if it means breaking existing APIs in the face of resistance.
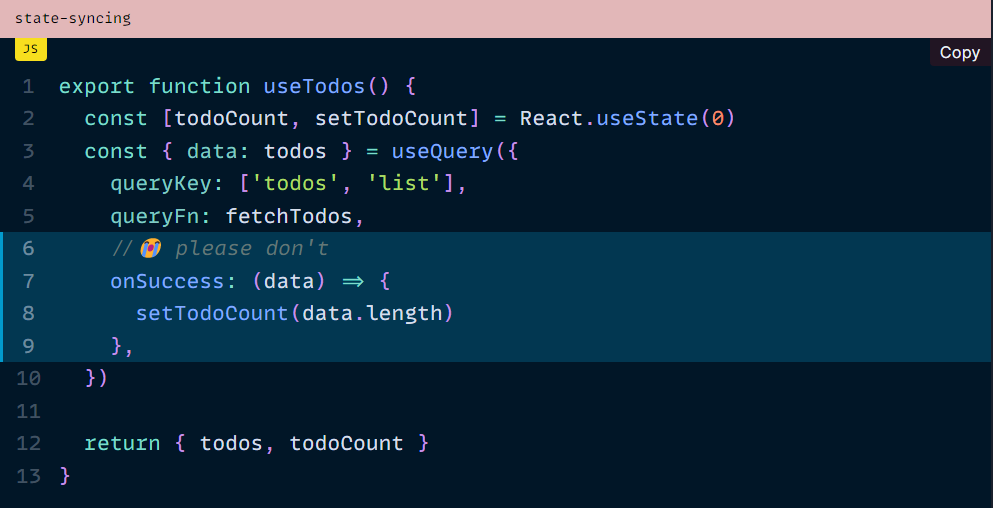