buttons not working
Hi! I have a code that should give a sample math test - it gives you a linear equation and two answer choices (one of them is correct) The code is pasted below:
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, SlashCommandBuilder } = require('discord.js');
module.exports = {
data: new SlashCommandBuilder()
.setName('mathtest')
.setDescription('Gives you a math test'),
async execute(interaction) {
var a = Math.floor(Math.random()10)+1;
var b = Math.floor(Math.random()10)+1;
var c = Math.floor(Math.random()(20-(a+b)))+a+b;
var d = Math.floor(Math.random())+1;
var aone;
var atwo;
var sol;
if(d === 1){
aone = (c - b)/a;
atwo = Math.floor(Math.random()10)+1;
sol = aone;
}
if(d === 2){
aone = Math.floor(Math.random()*10)+1;
atwo = (c-b)/a;
sol = atwo;
}
const answerone = new ButtonBuilder()
.setCustomId('answerone')
.setLabel(
const response = await interaction.reply({ content:
const collectorFilter = i => i.user.id === interaction.user.id; try { const answer = await response.awaitMessageComponent({ filter: collectorFilter, time: 60_000 });
if (answer.Label ===
}, };
${aone}
)
.setStyle(ButtonStyle.Primary);
const answertwo = new ButtonBuilder()
.setCustomId('answertwo')
.setLabel(${atwo}
)
.setStyle(ButtonStyle.Primary);
const row = new ActionRowBuilder()
.addComponents(answerone, answertwo);
const response = await interaction.reply({ content:
Solve the math problem, ${a}x + ${b} = ${c}
,
components: [row],
});
const collectorFilter = i => i.user.id === interaction.user.id; try { const answer = await response.awaitMessageComponent({ filter: collectorFilter, time: 60_000 });
if (answer.Label ===
${sol}
) {
await answer.update({ content: Sorry, incorrect
, components: [] });
} else if (answer.Label !== ${sol}
) {
await answer.update({ content: 'You got it correct!', components: [] });
}
} catch (e) {
await response.editReply({ content: 'Nobody answered!', components: [] });
}
}, };
56 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
oh ok - i meant the label property but i think i can change it's custom id to the respective answer too
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
hm - it's still not working - here's the code:
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, SlashCommandBuilder } = require('discord.js');
module.exports = {
data: new SlashCommandBuilder()
.setName('mathtest')
.setDescription('Gives you a math test'),
async execute(interaction) {
var a = Math.floor(Math.random()10)+1;
var b = Math.floor(Math.random()10)+1;
var c = Math.floor(Math.random()(20-(a+b)))+a+b;
var d = Math.floor(Math.random())+1;
var aone;
var atwo;
var sol;
if(d === 1){
aone = (c - b)/a;
atwo = Math.floor(Math.random()10)+1;
sol = aone;
}
if(d === 2){
aone = Math.floor(Math.random()*10)+1;
atwo = (c-b)/a;
sol = atwo;
}
const answerone = new ButtonBuilder()
.setCustomId(
const response = await interaction.reply({ content:
const collectorFilter = i => i.user.id === interaction.user.id; try { const answer = await response.awaitMessageComponent({ filter: collectorFilter, time: 60_000 });
if (answer.customId ===
}, };
${aone}
)
.setLabel(${aone}
)
.setStyle(ButtonStyle.Primary);
const answertwo = new ButtonBuilder()
.setCustomId(${atwo}
)
.setLabel(${atwo}
)
.setStyle(ButtonStyle.Primary);
const row = new ActionRowBuilder()
.addComponents(${aone}
, ${atwo}
);
const response = await interaction.reply({ content:
Solve the math problem, ${a}x + ${b} = ${c}
,
components: [row],
});
const collectorFilter = i => i.user.id === interaction.user.id; try { const answer = await response.awaitMessageComponent({ filter: collectorFilter, time: 60_000 });
if (answer.customId ===
${sol}
) {
await answer.update({ content: Sorry, incorrect
, components: [] });
} else if (answer.customId !== ${sol}
) {
await answer.update({ content: 'You got it correct!', components: [] });
}
} catch (e) {
await response.editReply({ content: 'Nobody answered!', components: [] });
}
}, };
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
it's currently saying "application did not respond"
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
i think its bc the code just isn't working
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
alright it's executing but it says that it's not working
how do i do it without passing template literals?
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
because i want to have two answer buttons and, generate two numbers, one being the answer (sol) and the other being a random number - then, by random (purpose of d variable), choose which button gets correct ans and which gets wrong ans
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
If you aren't getting any errors, try to place
console.log
checkpoints throughout your code to find out where execution stops.
• Once you do, log relevant values and if-conditions
• More sophisticated debugging methods are breakpoints and runtime inspections: learn moreright now it's saying "application did not respond" but idk why because all my other commands execute fine
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
yea i'll comment out parts of my code to see
it was working a while ago tho
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
im pretty sure there are errors but right now it's not executing so its prob taking too long
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
yea i played with the template literals
it doesnt say anything there tho
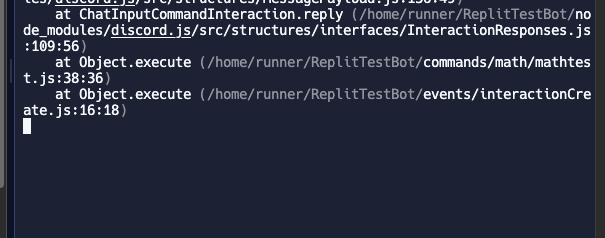
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
i think everything above that in the console is from previous stuff
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
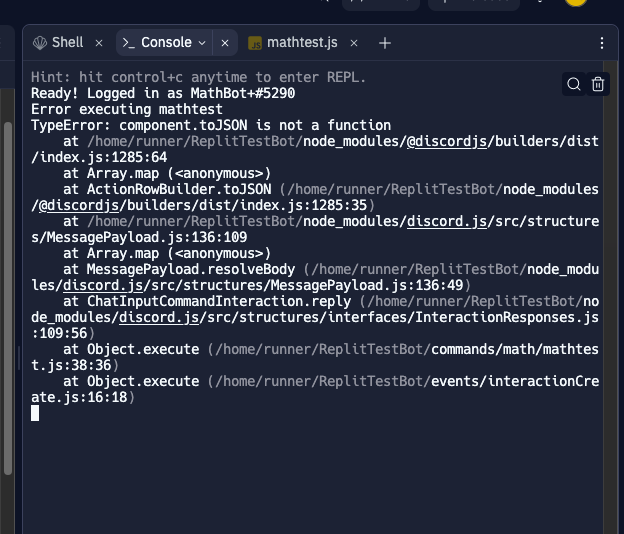
i never use .toJSON tho so idk what that's about
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
yea but now my ids are ${aone} and ${atwo}?
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
oh
but i never define answerone or answertwo anywhere
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
ohh so
those things should put the button names, or the button ids
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
oh ok
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
oh ok yea now it's responding
my current code is still in the debug console.log mode i'll see if the actual code works
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
regarding the template literals is there any other ones that should be removed?
its kinda messy to put those template literals as customIds
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
hm but the sol thing isn't working
sol should be equal to whichever is (c - b)/a and then i have the if(answer.customId === sol) or else if (answer.customID !== sol)
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
yea so either aone or atwo is (c - b)/a and whichever is would be equal to sol so if(answer.customId === sol) should be true only if answer.customId = (c-b)/a
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
well its kinda confusing with the template literals but yea aone and atwo should also be float numbers and then sol would be a float number but then idk if custom Ids can be numbers
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
oh ok yea idk but should i still do the
${}
thing?Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
but it's not currently working
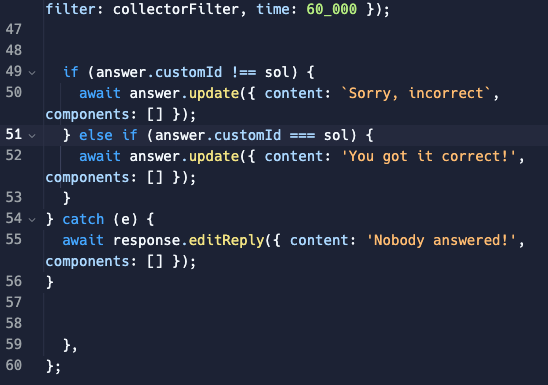
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
ok
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View
ok i think its working now
thanks
Unknown User•14mo ago
Message Not Public
Sign In & Join Server To View