Next-Auth Session in Server Function
Trying out the new app directory with an example project and ran into issues with accessing the user session within a server function via
getServerSession
Calling this code from an action
handler within a form yields the following error Method expects to have requestAsyncStorage, none available
.
Is there anyone who has successfully gotten this to work?21 Replies
are u doing this in the api folder?
This is a server function in a file
actions.ts
co-located along pages.tsx
well when im doing something like this i usually write this code in the api folder and send the form data from any component using axios. Ive done it many time in the app dir. Ive not really gone deep into using the "use server" , and server functions
is this on the edge runtime?
or locally/lambda
this is locally
no edge runtime
Okay hold the fuck up. If I call a server function from a server component I can access
getServerSession
. As follows
But If i call the function from a form action
, it errors out.
Shouldn't both be called on the server side thus have the same access to the cookies????
maybe nextauth is not ready for app directory?
Its actually not a NextAuth issue I found out. The error was w/ accessing the browsers storage so I tried to access cookies just for fun
It gave the exact same error
well you can't access any browser storage with code that runs on the server (without passing it in as an argument)
not browser my bad. Same story though. https://nextjs.org/docs/app/building-your-application/data-fetching/server-actions#action
Data Fetching: Server Actions
Use Server Actions to mutate data in your Next.js application.
This snippet when called from a form action within a client component will not have access to cookies; which seems wrong?
Its called on the client, but the action itself is a server action. Thus it shouldn't matter if a server or client component calls it so long as its done in the appropriate manner
check the request in the dev tools of your browser, see if the cookie is sent
yep in both cases the cookies are sent. Yet errors out when the server function is called client side via action
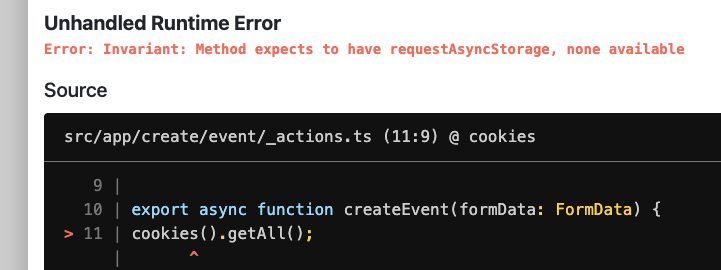
I'm not the first to discover it apparently https://github.com/vercel/next.js/issues/49235
GitHub
Importing server action from 'use client' component results in erro...
Verify canary release I verified that the issue exists in the latest Next.js canary release Provide environment information Operating System: Platform: linux Arch: x64 Version: #202204271406~165547...
oh you're using an action in a client component, i don't know if you're allowed to do that
According to their docs it should be 100% allowed.
Data Fetching: Server Actions
Use Server Actions to mutate data in your Next.js application.
action as in
action={...}
you're definitely allowed to call them as function
but i don't know if you're allowed to have them on a <form>
tag's action
attribute
ok looks like you canwell the temporary work around is to just call it straight from a server component I guess. Im on 13.4.1 so I'll keep my eye out for a fix I suppose.
have u found the solution?
Hey Guys, I found this thread through search and thought I'd chime in: There is currently no official fix for this but there is a workaround for the time being:
Wrap your client component in a server component. Import the server function in a server component and pass it as a prop to the client component and call it there. That works but is more boilerplate than I would like.
Like this:
Now using cookies or headers from
next/headers
works fine.