Javascript nested loops for beginners
Hello folks!
Why does this code print 1 2, and not the names that are similar in the arrays?
Comes from codecademy's JS beginner course
8 Replies
take a look at the function definition on MDN:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/push
It says under Return value:
The new length property of the object upon which the method was called.if you want to log out the follower's name, either outside the loops run
console.log(mutualFollowers);
or inside the loop where you have it now log out bobsFollowers[i]
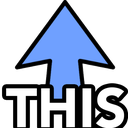
push()
and unshift()
return the length of the array, not the contentsOk, then that makes sense, but why 1 and 2? Is that the indeces of the bobs when tinas match?
it's the length of mutualFollowers after the push operation
when it runs into Kim, it pushes Kim to the mutualfollowers array and returns 1 for the new length
then it runs into Nim, and pushes that to mutualFollowers, and logs out 2 for the number of items in mutualFollowers after pushing Kim and Nim
Ah! Now I see, so i mixed up index number of each content, and the content number it self.
I was expecting the index number of bobs to appear.
that's simply not what push returns
Got it! Thanks! 👏
np!