EmbedBuilder troubles
I've beeb trying to make an inventory sort of embed for a couple hours now and im completely stuck.
i'm sure theres a way to do this but I can't figure it out, .addFields in the embed just doesnt seem to work when theres a for loop and It's just what i need so i'm not too sure what to do
here is my code:
i've also sent an image with how my folder structure looks like
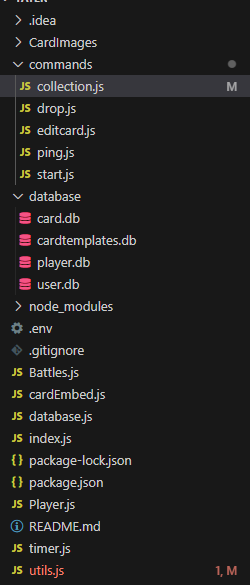
6 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.and just incase its needed, heres my collection.js code
help would be really appreciated, i haven't been this stuck on coding this as much as now
what version are you on?
iirc in v14 .addFields is an array consisting of
{name: "", value: ""}
objectsI'm preeetty sure I'm v14, I thought I tried that and it didn't work but ill try again, thought I'm really unsure if I'll even syntax it properly
Yeah I tried that and wouldn't work, I've been working on smthn a lil differently which also didn't work but I'll post my code in a bit
im using these functions like a recursive type of thing
and im getting output
but im only getting the first card in my database thats assigned to my userid
the maxCard's i have just simply doesnt work and im not sure why at all
the code i have in collections.js hasnt changed
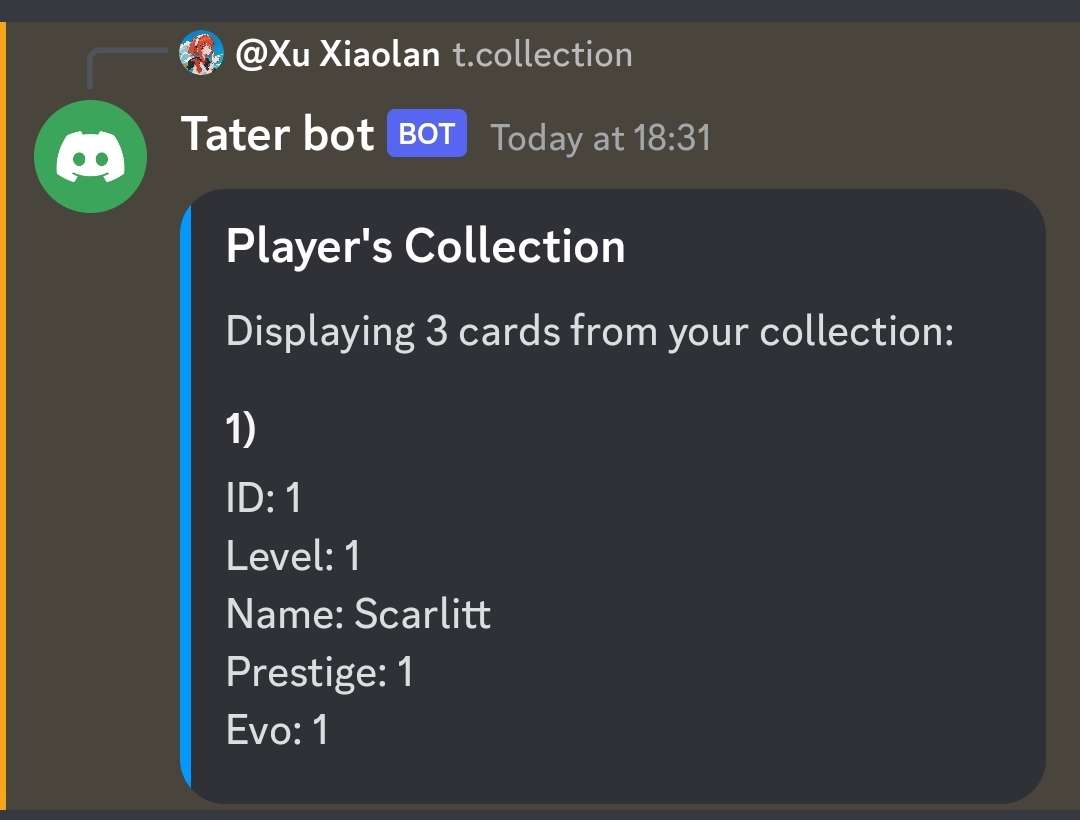
Oh that could be it, cardIds should be a list of the ids a player owns
Then if it's that, then getListCardId isn't working
I'll need to check that
Ig ill read over this and see if it's doing as it should
Oh goddammit
I put LIMIT 1
That was indeed the problem, thanks guys
I'm so stupid lok
Lol