Clear input field after click in the button
Hiii everyone. I am beginner in React and I am trying to clear the input field everytime I add a new task or simply, everytime I click in the button "Add". But apparently, just putting setNewTask(''); in the addTask function did not work and I wanna know why...
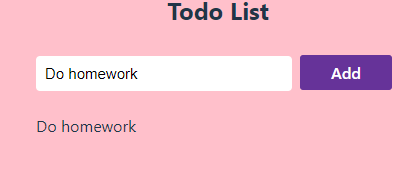
39 Replies
I think (and I am also relatively new to React so I'm sorry if I am wrong), that you need to tell the input that it's value is "newTask". i.e. you need to add value={newTask} to your input field.
it worked...But why?
I though it was not need the {newTask}, because I already have the onChange={handleInputValue} in the input
Because the input field needs to "know" that it's value is the value of newTask so, when you change the newTask value it will be updated.
The handleInputValue function is setting the newTask value by getting the value of the input field. But it is not setting anything in the input field, only retrieving.
For the input field to "know" that its value is supposed to be the same as the newTask value you need to tell it that. This is done by setting its value to the value of newTask, otherwise there is no relation between your input value and the newTask value.
it makes sense. Thanks so much π
@Chris I found out something that is bothering me in the code. When I add more than five tasks, the content inside of the container, is going up in a way, that I can't see the input filed anymore. Can u help me with his, please?
That is probably because you have kept the default styling from when you set up the react project which places the contents in the center of the page, both vertically and horizontally.
Therefore, as you add more items the contents gets βlongerβ (taller) and the centering pushes it up (and down) to keep it centered.
You need to look in the css files, probably the app.css, and adjust the position of your container. I think that you will find this defined in the .app class.
yeah, I made some changes in the app.css file. It looks like this:
margin-top: -270px
canβt be good π
it was aligning automatically the content in the center of the page without the align-items: center defined in the body. So, I found this solution to put the text where I wanted hahah
but I tested removing the margin-top to see if that was the problem. It wasn't
it's notthe problem, but having a negative margin top is not the solution either.
I don't know what to do
When you setup a new Rect app by default it actually includes 2 css files - index.css and app.css. index.css is where the general styles are defined and app.css is where the component specific files are defined.
You have changed the app.css but you also need to look in the index.css.
should I delete everything there?
not necessarily, it would be better to try to understand what is causing the issue so you can remember it for the next project.
Can you use the inspector to see how the elements are defined? This should help you work out which one is pushing the contents down.
let me see
the body
you are getting warmer π
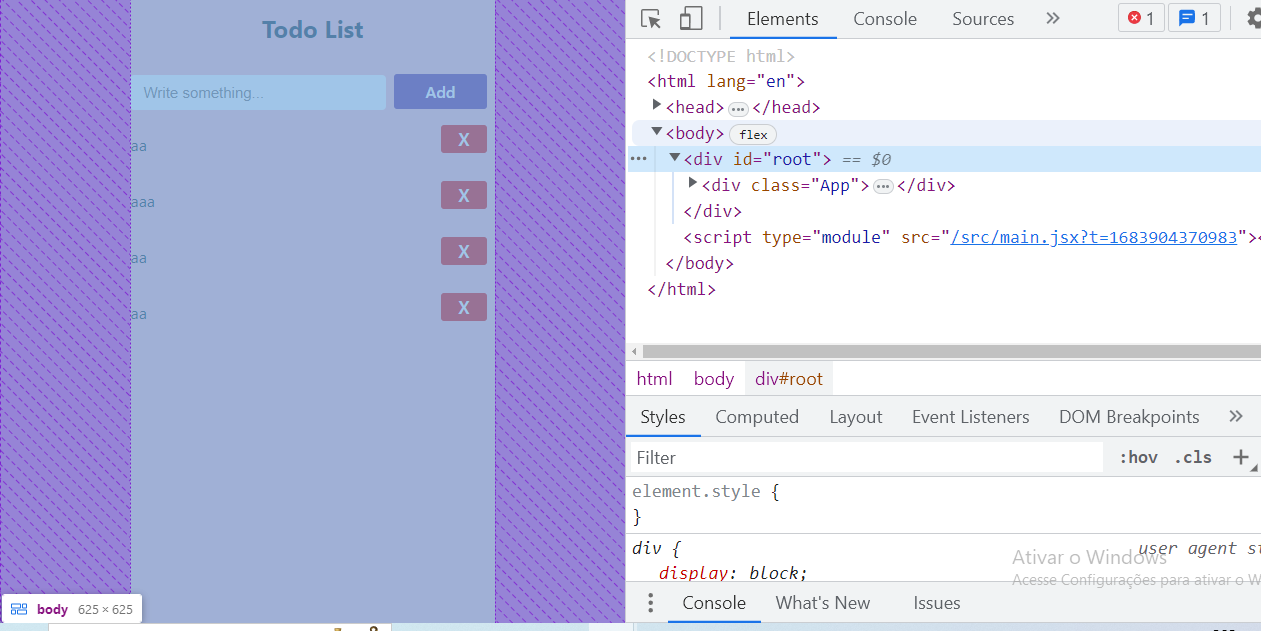
really? maybe is the root. I still don't understand the existence of the root π
the id root is point to the last task
the root is where you can define styles for the whole document - use carefully! It is usually used to define css custom properties such as colors that will then be used repeatadly throughout the code.
ok, get it now
actually, it is point out to nowhere in the page. It is strange
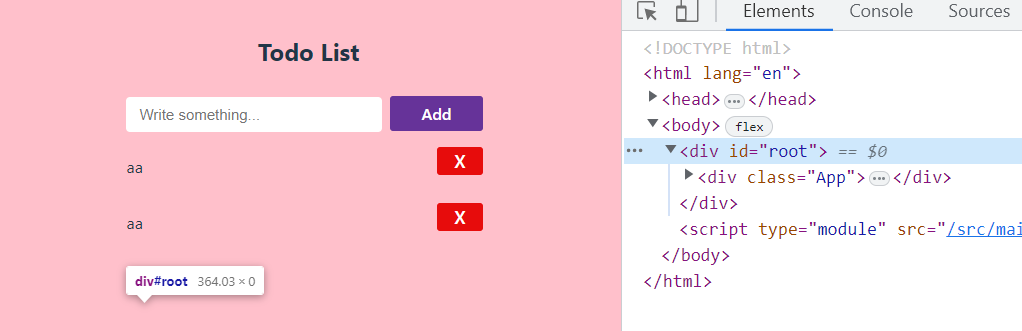
ah, one second... in react, rather annoyingly, they also define a class with the name of #root - this is not the same as :root
You need to look at the css styles in the inspector. Look at how each element is styled.
In your case I suspect that the issue is with the styling on the body tag
it is good to know it, I was confused about this
This is defined in the index.css and, whilst you have re-defined it in your app.css, you have not overwritten all of the values.
yeah, I think this as well
In index.css you will see that body has
place-items: center;
This is what is causing your issue.yes, it has
when I try to remove now, everthing dissapear in my page, just the background-color remained
Have you still got that negative margin-top on your h2?
That will pull things up out of view now
yes, I forget about it
easily done π
oh man, I can't thank u enough for the patience and help
In summary, when starting a new react project it is highly likely that it will be defined with some default css along with a logo and a demo counter. You need to remember to either delete or adjust these otherwise they can easily mess-up your code as you have seen.
I do tend to delete everything but I wanted to help you identify the issue first so you can better understand why it happens.
Right away, when I was writing my first react code yesterday, I was suprised by the fact that the button was predefined styled in a different way hahah. Now, everthing makes sense
Yes, I agree that it is rather confusing. I don't know if it is possible to install React without all the default code and assets but it would be good if it were.
yeah, I will have to find a way to configure this thing
The most confusing thing is the use of the id "root"
haha thanks
I will search this because I am curious
yeah, but it is need for mix hmtl and javascript all together, right?
By default react uses "root" as the id of the main (and only) div in the index.html file.
The components are loaded into this.
Now I may be wrong but this is nothing more than that, an id. You should be able to change this to whatever you like. However if you do, don't forget to also change it in the main.jsx file as this is where React is defining where to place the components with a simple document.getElementBy() selector :
ReactDOM.createRoot(document.getElementById('root') as HTMLElement).render(
for example something like "react-root" would be clearerthanks, I got it. How long are you learning React?
3 or 4 months now. I am complicating it further by forcing myself to use Typescript which I find more annoying than helpful but it is pretty much a requirement these days. I am also learning Astro and about to start on Svelte so it doesn't get my full attention .... there is so much to learn! π