Why is the zero-indexed item in my $array not recognised?
results:
$array does not contain 0
$array does not contain 1
$array does not contain 2
$array does contain 3
$array does not contain 4
$array does contain 5
$array does not contain 6
$array does contain 7
$array does not contain 8
$array does contain 9
18 Replies
in general, you shouldn't rely on type inference in your
if
statements
don't just if($value)
actually compare it to something (unless it is already a boolean) if($value) !== 0
and to answer your question, when you rely on type inference php basically..."converts" the result to a boolean type, and 0
is a falsy value, the array_search
function returns the index of the found item or false if it's not there
which means when that function returns 0
(the index) it takes it as false
is there an easy way to overcome this ?
like I showed
you just compare it to something
if (array_search($c, $array, true) !== false)
ahhhh gotcha, thank you so much
otherwise what your if says is basically
if(0)
which is translated to if(false)
funsies of type inferencei had been using just one entry in my original array, and it being the zero-index was continually being false, and i thought it was other aspects of my code that was wrong - been pulling my hair out for 2 days now lol
ah yes, when you have issues with
if
statements save the value to a variable instead of putting it inside the if
statement, and log it
so you can see what is actually being compared
I am very confident in JS I still don't, but I'm more of a strict type programming language guy, so that's why
it is good to learn how type inference works tho, that can cause a whole lot of bugs, so should give it a readi have made a note for future, thank you so much
👍
i am currently learning JS
oh?
well Js has the same issues so
also a good read
and I think they're very similar no, Hope?
in that regard at 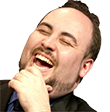
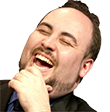
in many of my arrays i put a fake entry in the zero index and start counting from 1
oh
no need for that
can you?
like TS kind of types or python types where it is actually integrated
just...extra optional 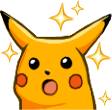
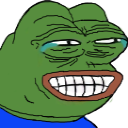
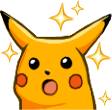
what does that do?
i don't use them either
you just added one point to php on my list of languages to use for a project 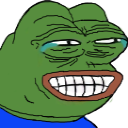
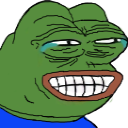
PHP mostly, but learning some JS for dynamic user interaction
eh probably not something you should worry about yet, altho it doesn't seem super restrictive
but still, focus on type inference is good to know
then you can get to strict types
thank you again, I will sleep tonight now 🙂
when i say tonight, of course i mean toDAY lol
another issue has arisen now lol
i am actually comparing one array with 3 others, and it is "finding" a positive result where there is none, even in an empty array