encyclopedia embed type thing
hey so i was trying to make a dex or enc for my bot's items and i keep getting this one error:
Uncaught DiscordAPIError DiscordAPIError[50035]: Invalid Form Body
embeds[0].description[BASE_TYPE_REQUIRED]: This field is required
at handleErrors (c:\Users\Moh\Desktop\clutter\Gacha-Bot\bot-20\Tater\node_modules@discordjs\rest\dist\index.js:640:13)
at processTicksAndRejections (internal/process/task_queues:95:5)
here is any relevant code:
27 Replies
⢠What's your exact discord.js
npm list discord.js
and node node -v
version?
⢠Post the full error stack trace, not just the top part!
⢠Show your code!
⢠Explain what exactly your issue is.
⢠Not a discord.js issue? Check out #useful-servers.
i believe the main cause of the errors is just me trying to change the colour of the embeds
its supposed to change colour if the user doesn't have the card to be red, and green if the user does have the card
i've console log'd my variables and the embeds are post as they should be (atleast it looks like it) so i have no idea where the error is coming from
the error originates from your embed description
You're sending an empty embed, log the embed
this is what i get when i log it, is it something with how im returning the embed?

Can you not view those objects? I'm trying to see what the embed has
i dont have a debugger to view the variables it says, is there an extension for it?
embeds
here is an array of objects like these:
{ embeds: [...], files: [...]}
from what your code shows meyeah im trying to make a collection of embeds that the user can go through is the goal
Then you're doing the wrong thing, here's basically what you're doing:
Try this,
.send({ embeds: embeds[page].embeds
alright
hmm now its showing the embed but the colour is always red, ill try messing with it a bit more, this is definitely a step forward!
You understand what I was saying though?
yeye, i was just accessing the properties wrong right?
Yes, also to fox that color thing, change the value you passed to image Embed.setColor in the if(color) statement
In your createEncEmbed function, do .setColor(color)
ah okay, that makes more sense lol
when im setting the value of colour, should it be as a string or does it not matter?
like in here
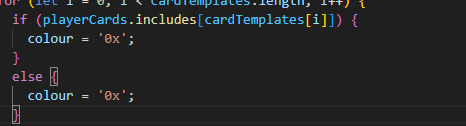
i heard javascript is really finnicky with these things
its mostly working now, but problem with colours still, and i was told the problem was somewhere around here
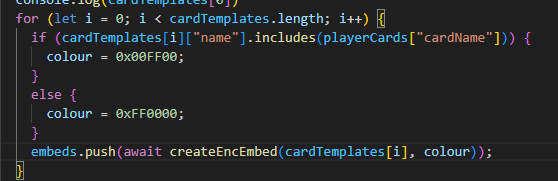
probably with how im using .includes
the if statement always evaluates to being false
nvm i just did it another way that seemd to work, im sure i could use .includes but i cant really figure it out lol
now just the last thing i have to do, allow the image to also pop up xd
oh wait i never declared it... that couldn't have been the problem could it? 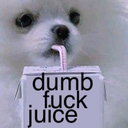
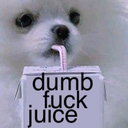
now i just use 2 for loops for it
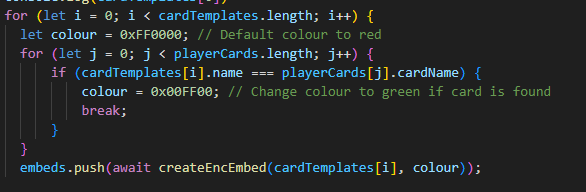
well i'd want it to change sometimes, i wouldnt want it to stay a constant
like in these two scenarios, green being the user that card, red being the user does not, ill look into .some though cuz its always good to learn some new code
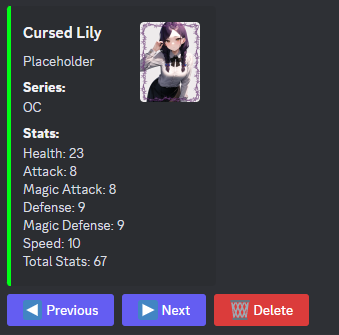
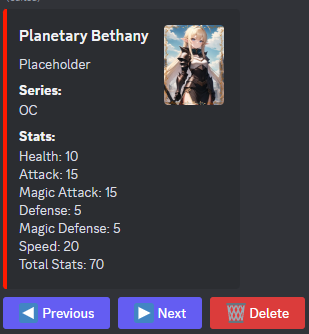
I said the error is here
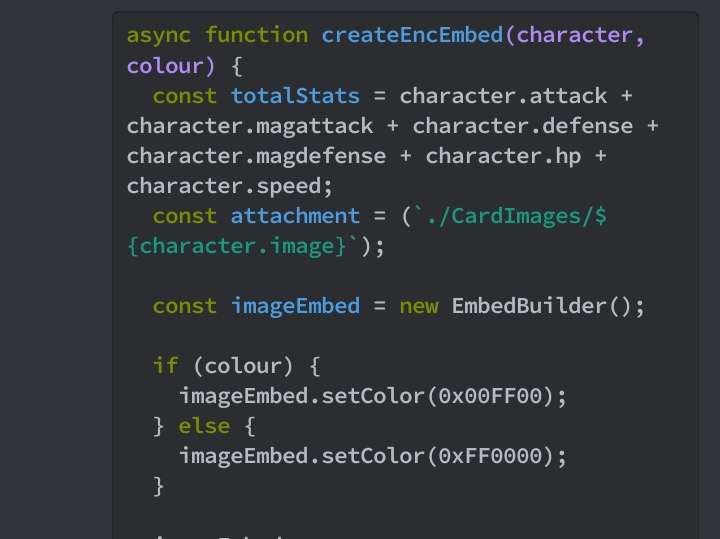
In your if(color), you don't set it to the color
yeah i noticed that, it still hadn't worked then, the problem was just with my .includes
ah ic, ill look into it if its more efficient then (doubt 2 for loops are more efficient lol)
thanks for the help guys ^^