Problem saving data 2 times in 1 request
When I send a request 1 time to an index.html file that says hello world, the data KV is recorded 2 times, how can I solve this problem?
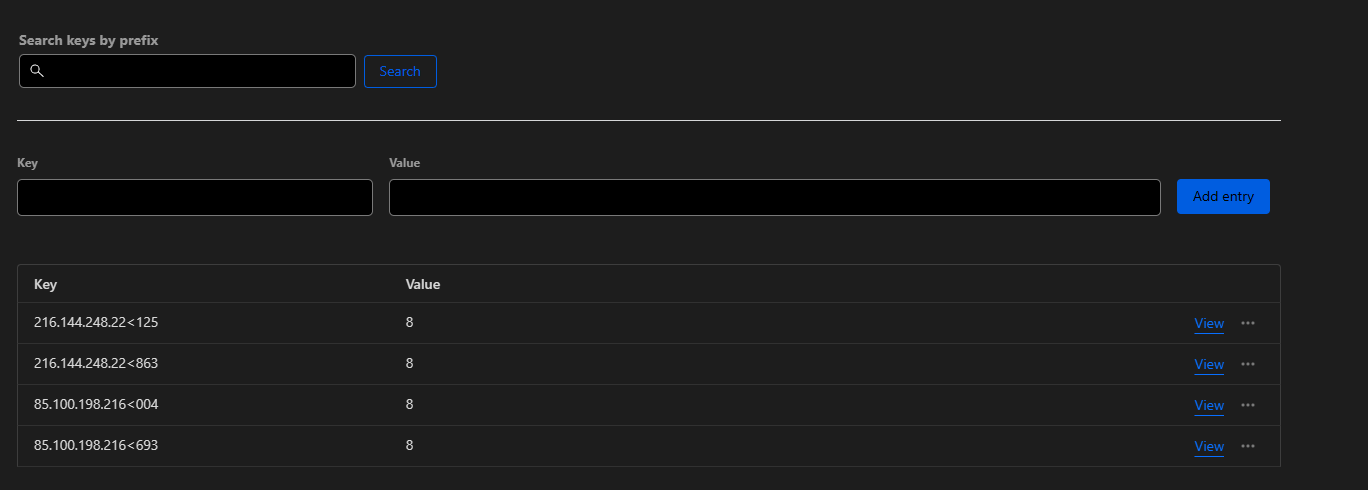
10 Replies
it's probably because your browser is doing at least 2 requests, even if the page has no other resources, it's getting the raw html & favicon.ico (automatic)
The worker is ran for every request to every resource, unless you made the route just
/
and not /*
(and not using a custom domain)Thank you for your answer @chaika.me 🙏
if I do not use /* it will not work in other url paths how can I prevent this
well just to exclude favicon requests, you could do something like
(rewritten to module format, don't use service worker format, it's ugly and slower)
if you only want one log per user per session, you could send back set a cookie and check it
I just want the ip address to be recorded as many requests as the user sends.
well your browser automatically making a request to favicon.ico is a "request the user made"
I understand you... I do not want to record the request from other automatically running services such as png, ico, php that the website runs
For example, In xenforo forum software, many files are running in one request
It is really a pity that when a user sends a request, it is saved in the KV storage many times
one last question
do not want to include favicon.ico and style.css
is this usage correct
looks ok to me
hmm being in a proxy position is a bit special, you could try something somewhat primitive like checking for the returned content-type being text/html, or maybe the referrer headers.
thank you I'll try that mate ❤️