Receiving an axios.post() request in app.js
This is my axios.post() sending my form's input.value to the server
In my app.js, when I set up the route to receive this axios.post() request, I destructure
HOWEVER, when I have
req.body
into a name
variable, and then attempt to console.log(name)
.
In the console though, I get undefined.HOWEVER, when I have
app.use(express.json());
in my app.js
, I do see the 'name' value consoled.
Whether or not this app.use(express.json());
is included in my app.js though, I will see this name
reflected in the "payload" tab and the res.status(201).send()
/res.status(401).send()
logic result in the devtools network "preview" tab.
character cut-off: My question will be in the first comment11 Replies
That sounds right, you need to parse the incoming request using a middleware like express.json
1. Why do I need
app.use(express.json());
in order to see the consoled name
? When I'd used the browser-submitted form (method = "POST"), I didn't need app.use(express.json());
in order to console my destructured name
.
2. I also notice that with the browser-based POST request, the app.post() actually sent me to the path /login
URL upon submit, and the page displayed what I specified in my .send().. meaning like the form disappeared and the .send() string appeared. Where as with my axios.POST response.. the URL doesn't change to reflect the specified path /api/people
.. The only evidence I have that the .POST request was successful is if I go into my dev tools Network, and look in the Preview tab.. which shows the .send() string message I'd created.When you say browser-based request what do you mean?
nvm, okay, yea, I sent it out with axios.post() as a json, therefore in app.js, it needs to be un-json-ified with express.json(), that makes sense
That's right 🙂
There are other formats that you can specify like plain text or raw for raw bytes, each with its own middleware to parse it in express
can we leave a pin in this.. i need to rnun right now, but i wanna finish this
Sure no problem
So browser request, I just mean a regular form submission to a server, with a method = "POST", from the html page.
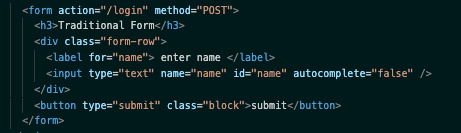
but with axios.post(), say if I'm sending a form input value.. I would need to send that as .json regardless right?
.. and then in my app.js, I'd destructure whatever I wanted out of the json, using express.json().
But I couldn't, for instance, send the information from axios.post() as a string, right?.. It has to be sent as a .json?
Yes, you can send the data in form of string. It's just that JSON is so common that it's the default used which is why you need to parse it on the express application.
I haven't used axios in a while so I'm not entirely sure but I think the option you need to change is
responseType
https://axios-http.com/docs/req_config
The only evidence I have that the .POST request was successful is if I go into my dev tools Network, and look in the Preview tabThis is correct. When you submit a form the browser page will navigate to that route specified, unless you use the event method
preventDefault
. When you make use of axios (the native options are XMLHTTPRequest, which Axios uses under the hood, and the Fetch API) you are making a network request, but one that is asynchronous i.e., it doesn't interact the browser's navigation.at this point you've made several posts regarding axios and I'm inclined to suggest you learn to do all this without axios for 2 reasons:
1) the native
fetch
API can do everything axios does so it is unnecessary to install a package that does the exact same thing
2) it's generally best to learn how libraries/frameworks work under the hood if you have a good understanding of the technologies they are using you will have a better understanding of why they fail or why they do things a certain way
app.use(express.json())
is a middleware, which means it puts itself in between the request made to your server and your code, in this case this middleware intercepts the requests and parses them to json so it is functionally the same as getting the body of your request and doing JSON.parse()
the only advantage here is that it does it automatically which is WHY when you don't have it the request body is not parsed is a simple string so destructuring doesn't work because it is not an object at that point
when you send a POST request you don't send json, you can't send json, instead, what one does (and axios is doing this for you) is convert your javascript object into a string, send that string to the server and the server then takes that string and converts it back into an object
This is a POST request using the fetch API, you can see our body isn't json, instead we stringify
our object so it is sent as a string