upload thing jest
how to i set up testing with jest on components that include uploadthing?
[2:40 PM]
seems like it keeps throwing this error Cannot find module '@uploadthing/react' from 'src/common/tests/components.test.tsx'
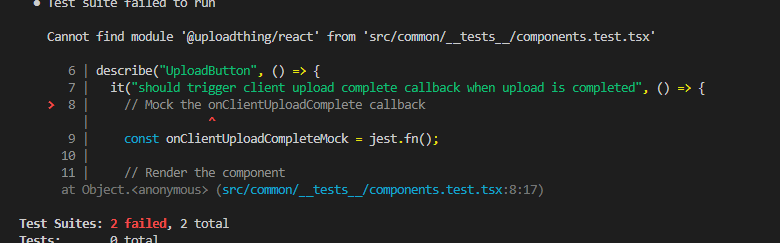
1 Reply
import React from "react";
import { render, fireEvent } from "@testing-library/react";
import { UploadButton } from "@uploadthing/react";
import { OurFileRouter } from "~/app/api/uploadthing/core";
describe("UploadButton", () => {
it("should trigger client upload complete callback when upload is completed", () => {
// Mock the onClientUploadComplete callback
const onClientUploadCompleteMock = jest.fn();
// Render the component
const { getByText } = render(
<UploadButton<OurFileRouter>
endpoint="imageUploader"
multiple={false}
onClientUploadComplete={onClientUploadCompleteMock}
onUploadError={jest.fn()} // Mock the onUploadError callback
/>
);
// Simulate an upload completion event
fireEvent.click(getByText("Upload"));
// Assert that the onClientUploadComplete callback has been called
expect(onClientUploadCompleteMock).toHaveBeenCalled();
});
// Add more test cases as needed
});
import React from "react";
import { render, fireEvent } from "@testing-library/react";
import { UploadButton } from "@uploadthing/react";
import { OurFileRouter } from "~/app/api/uploadthing/core";
describe("UploadButton", () => {
it("should trigger client upload complete callback when upload is completed", () => {
// Mock the onClientUploadComplete callback
const onClientUploadCompleteMock = jest.fn();
// Render the component
const { getByText } = render(
<UploadButton<OurFileRouter>
endpoint="imageUploader"
multiple={false}
onClientUploadComplete={onClientUploadCompleteMock}
onUploadError={jest.fn()} // Mock the onUploadError callback
/>
);
// Simulate an upload completion event
fireEvent.click(getByText("Upload"));
// Assert that the onClientUploadComplete callback has been called
expect(onClientUploadCompleteMock).toHaveBeenCalled();
});
// Add more test cases as needed
});