disabling an interaction menu
im just trying to disable an interaction after input from a player so that the messages can be properly deleted
27 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.
this code currently doesnt end the interaction menu and doesnt delete it either
you run .setDisabled but never update your selectmenu
select.setDisabled(true);
row.addComponents(select);
await interaction.message.edit({components: [row]});
something like this?
assuming your row is a new actianrowbuilder it should work
im getting unknown message error
specifically on line 307

the one with interaction.message.edit
because you never reply, only in your collector
sorry im a bit dum, wdym by that?
you use message.reply, but never interaction.reply
oh, what should i do then?
resolve the promise that it returns and edit that
also, response.delete isnt a property but a method
and i dont see response being defined anywhere
nvm
const response = await message.reply({
embeds: [embed],
components: [row],
ephemeral: true
it was defined
oh
lol
you need to edit that
and what is message? An interaction or a regular message?
an interaction, cuz its supposed to pop up an ephemeral select menu for the user to pick an option, then disable the menu and delete it for them
use response.edit
now im getting an error about invalid form body, component custom id cannot be duplicated, that means im using the same custom id as another row actionrowbuilder right?
create a new actionrowbuilder
or response.components[0].data.disabled = true
is one method more efficient than the other or does it not matter too much?
It depends on how you prefer but you have to wrap it in a new actionrow
this just almost works
the only problem i've been having a lot is that, when i use the select menu again, its like it runs the code twice in a row
so when it runs smthn like await response.delete(); it just gives me unknown message error cuz theres no message to delete
on the first iteration it works fine, afterwards it breaks like that
why are you setting your selectmenu to disabled if you delete the message anyways?
cuz theres a button to open the selectMenu again, that they can press after a turn has passed, so they can open the selectmenu again after the turn ends for both players
the first turn goes fine without any errors, but after that if user 1 or 2 uses the selectMenu more than once, i get the blue errors about unknown message, and if both players have used selectmenu more than once, i get the red unkown message error
its really weird i cant wrap my head around it
this is how it looks like, the other buttons work, switch opens up the switch menu, which is a select menu for cards i put in an array, all works just fine except all the unknown message errors im getting, they feel really random too, it never happens on the two player's first switch, but afterwards i always get this error
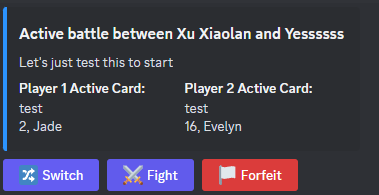

i figured it out, i must've worded my question wrong sorry, i wanted the collector to end so i didnt realise that there was a collector.stop()
thanks for dealing with this huge mess lol