Reply failing on button interaction, getting no errors
Just not sure what I did wrong here.
Looks fine according to the discord API guide as far as I can tell.
When I hit the yes button, I get an error in discord that says "This interaction failed."
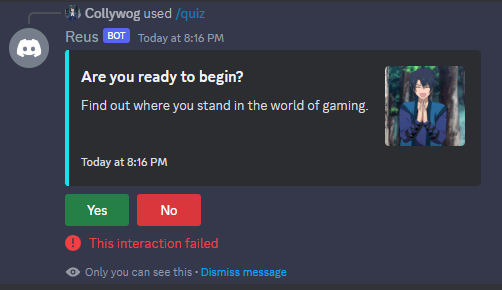
13 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.How are you acknowledging the button interaction that you get from clicking "Yes"?
that would be in a response
const response = interaction.reply()
That's how you acknowledge the command interaction, but you still need to acknowledge the button interaction
confirmation
You're doing it correctly for when the custom id is "answer2"
but you're not for "answer1"
oh so I would need another confirmation.update before the interaction.reply?
so I'm still confused, sorry!
I tried a few different things and this is where I'm currently at, but I'm still getting a failure. Am I doing the update wrong?
await confirmation.update({}) didn't work either.
confirmation.update({}) should've worked
Did it throw an error? And if it didn't, did "We're in the loop." get logged?
it threw the same error but We're in the loop. didn't get logged
That makes it sound like
questions
is an empty array, so I would recommend logging it to make sure it's populated
Also, you can only update()
an interaction once; you can't update it multiple times with each questionoh. is there a way I could update it for each question? I want it all to be in the same ephemeral to not cloud the chat
If you could update it multiple times, think about it:
It would update with the first question, then immediately it would replace itself with the second question
How do you want to handle showing each of them?
For example, you could make it so a prompt has to occur after the first question, then it shows the second question
Or, you could send multiple embeds in one message, each containing one question's data
You have lots of choices but you need to make sure you know what you're trying to end up with
I was hoping to have each question update the embed with editReply, that way it all happens on one messgae
is that possible?
is there not a way to nest waiting for a user's input inside an interaction?
If you're waiting for a user's embed between loops, you can use
awaitMessages()
or awaitModalSubmit()
to collect that input after updating/editing each embed
And yes, you can use update()
on the first loop (when i === 0
) and editReply()
on every other loop (when i > 0
) and it should work finethank you so much! I really appreciate the advice!