How to get Next-Auth session to include custom properties
I'm working on an app which uses the Next-Auth credentials provider - I'm running into a few related problems when it comes to adding some of my data into the session object and token.
I want to add in the username, role, id and profile image of a user to the session and token, and after adding an interface for session, JWT and user in my types definition I have managed to get the token including such fields, but session only has the original default properties in the session object - perhaps related to this, when I useSession and log it on a client-side component I see all my session object as it should be, but reloading the page then gets rid of it (I'm guessing due to the session issue).
Below is my callbacks and types definition for next-auth - I've look around a lot triyng to figure this out and keep running into more problems, so any help figuring this out would be greatly appreciated!
7 Replies
It looks like you're conditionally returning back your session. From what I have seen the session needs to be returned regardless and you alter the session if the token exist. This is the repo from where I got the next auth working.
https://github.com/shadcn/taxonomy/blob/main/lib/auth.ts
GitHub
taxonomy/auth.ts at main ยท shadcn/taxonomy
An open source application built using the new router, server components and everything new in Next.js 13. - taxonomy/auth.ts at main ยท shadcn/taxonomy
I tried that repo and I still can't seem to get it to work - I have done a bit of logging and it's confusing me a bit now in terms of what I am getting in response, using that repo I don't seem to be able to get any of my extended data to show up, other than in some logging, but it doesn't get returned at all.
This is what the above returns
Ok, this is what was first outputted when I signed in with Github. I added a console.log for the user because for some reason it was finding a user even though there wasn't any in the DB. So, I switched the Prisma query to findUnique and it didn't find a user thats why you see the value of null. I also added some default values to properties like role, username because I don't have those values in my DB.

I guess the session callback gets created when you use getServerSession function or some other way I'm using it within a server component. Anyways, it seems the JWT callback gets called again, but the user is undefined because UserTokenCheck does not get logged but the NoDbUserToken does. And again, the user is null. The last part is a console.log of the session in a server component.
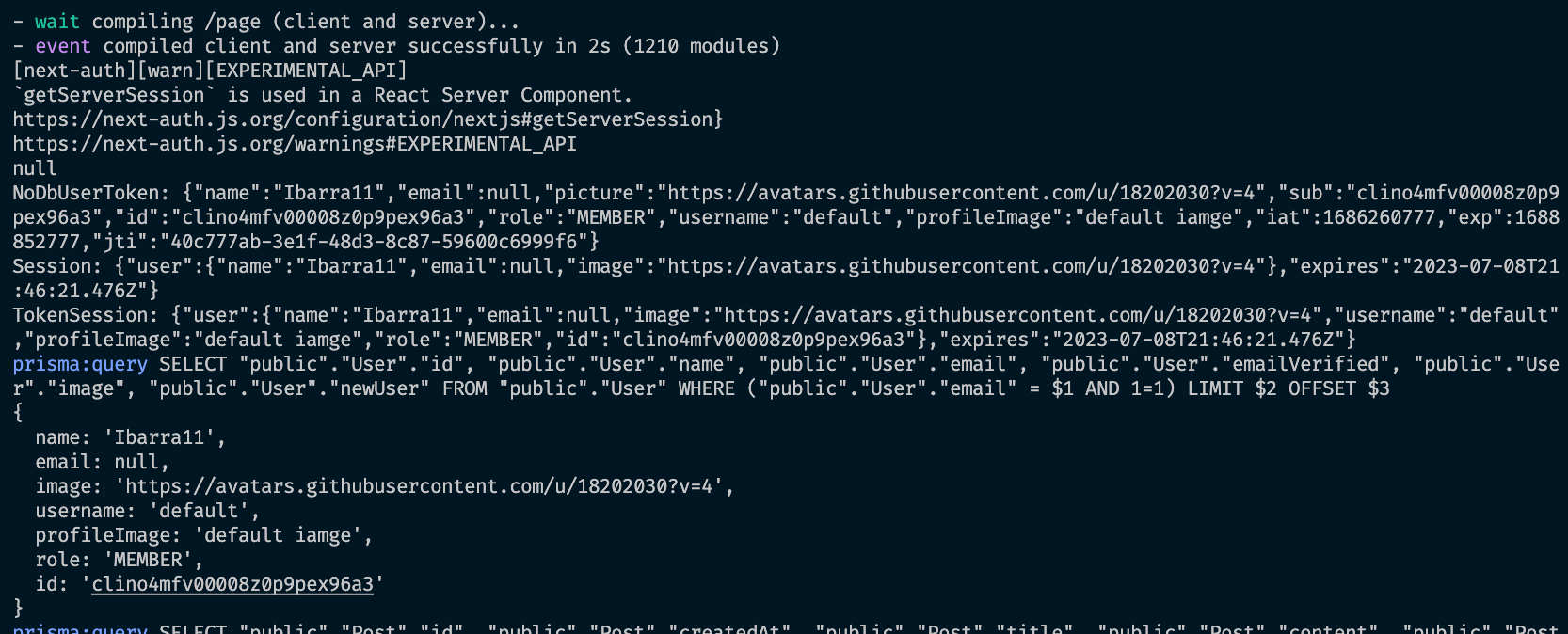
This is my server component and the function I use to get the session.
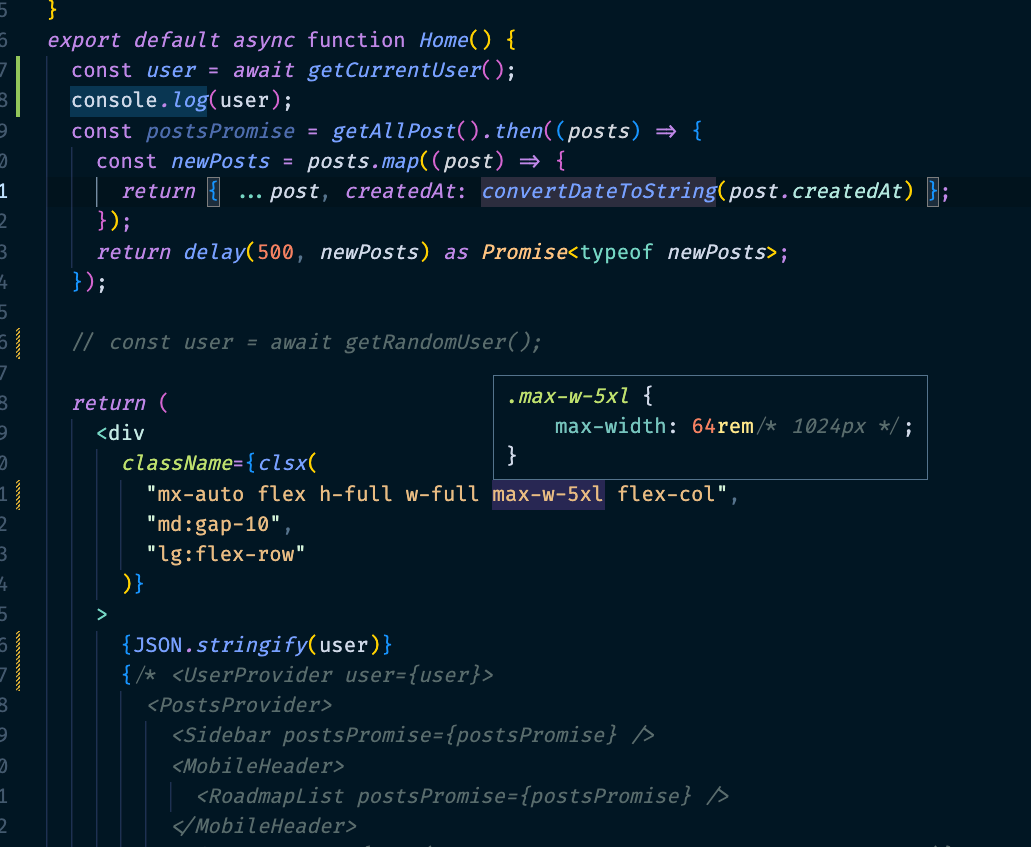
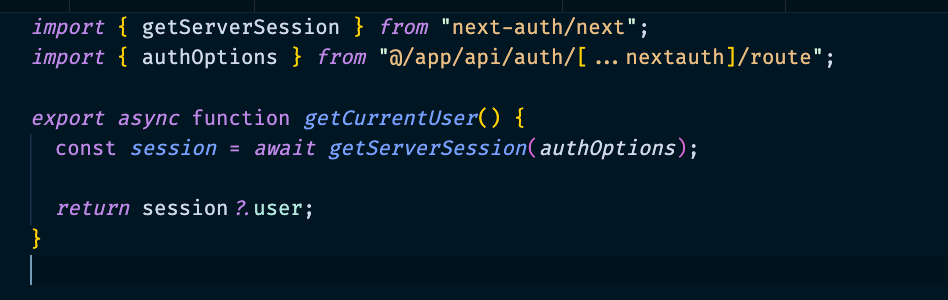
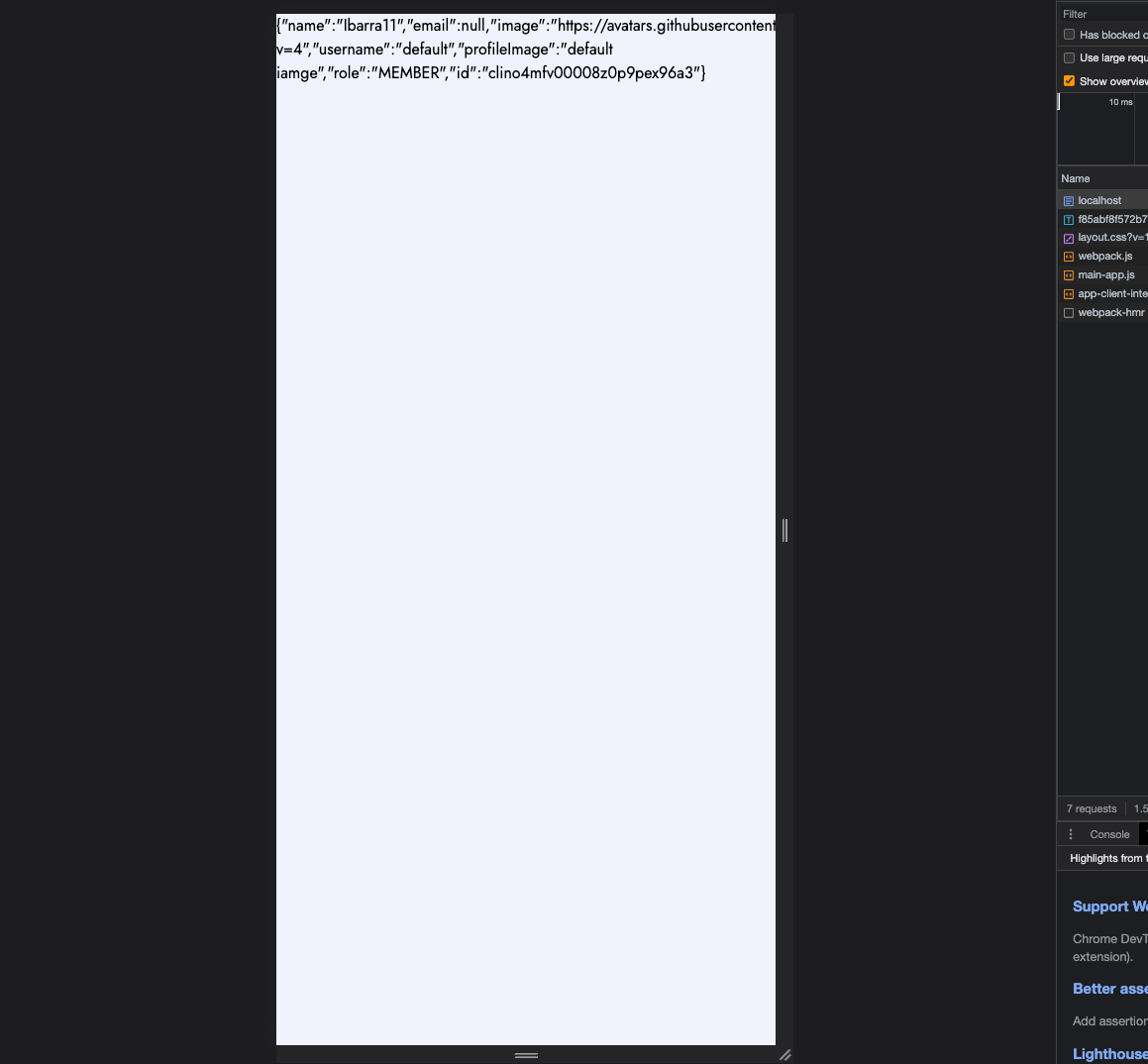
Lastly, this is next auth api setup
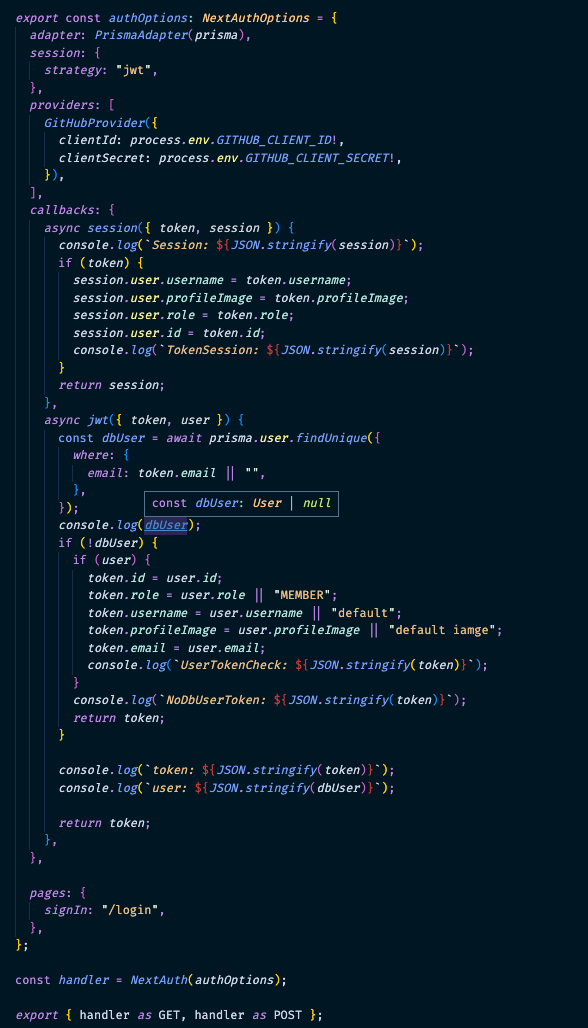
Thank you! This works, and I am getting the correct data back now! ๐ ๐