React: passing in arrays as arguments to components
GH: https://github.com/nnall/Inventory.git
I have a <Filter> component which is part of a pop-out menu. It's essentially a <div> of 3 sets of checkboxes. (pic 1)
In that pic, above the return, you can see I also have 3 arrays, one for makes, years, and colors..
I want to use another component, <Checkbox/>..
Inside the main returned <div> of <Filter>, I want to invoke <Checkbox/> 3 times, in three places, and each time somehow pass in either the makeArray, yearArray, or colorArray in as its argument, and then have <Checkbox/> map over that array, and for each array item return an <input type = checkbox>, so returning however many inputs as there were items in the array, and insert them into the returned Filter html where<Checkbox/> component is..
I was really just hopping to have a component that quickly returns little pieces of html. for each array item.
Can I pass in these arrays as arguments into <Checkbox/>? If so how? Or do I need to take another approach?
What would I have to do to invoke each <Checkbox/> as a function with an argument, and then how would I receive that argument in the component itself?
GitHub
GitHub - nnall/Inventory
Contribute to nnall/Inventory development by creating an account on GitHub.
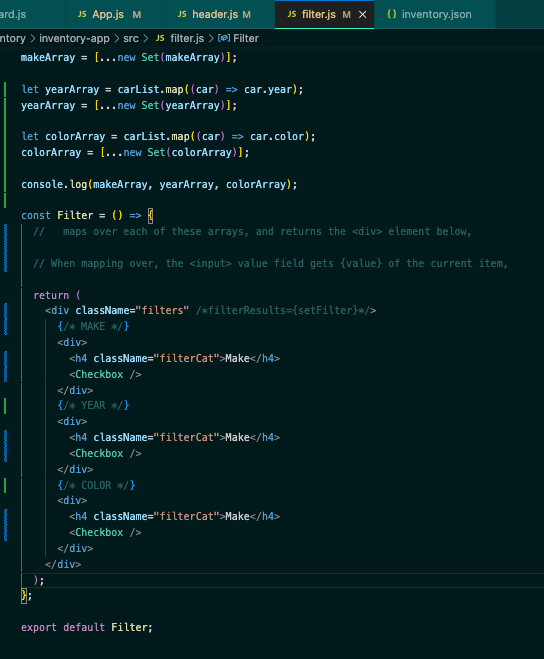
2 Replies
Hi! I encourage you to read the documentation to get a better understanding: https://react.dev/learn/passing-props-to-a-component
Basically you want to use
props
to achieve this. You've already used this pattern here: https://github.com/nnall/Inventory/blob/main/src/card.js. If you want to pass data to your components, you can pass attributes to your JSX element: <Card yourAttrName={attrValue} />
. You can read these attributes in your component like this:
There are no restrictions about the type of your props
. You can pass strings
, numbers
, objects
, etc.
One more thing. I saw that you're using React.createElement
, and that is not the recommended. You can simply do this:
Passing Props to a Component – React
The library for web and native user interfaces
There is nothing to be gotten from the items inside my arrays.. the array 'items' are just strings, not objects, so I'm not trying to get properties from them. In this Filter component, I just want to use .map() on each of these arrays, in order to output <input/> checkboxes, however many is in the array.
I would like to be able to set the for attribute inside the inputs as well, based on the array being mapped over, so that I'll know which field in my 'cars' inventory json (full of 'car' objects) to search on (ex year, make etc), when an input is triggered.
I want the inputs being created from mapping over colorArray for example, to be set to for = "color"
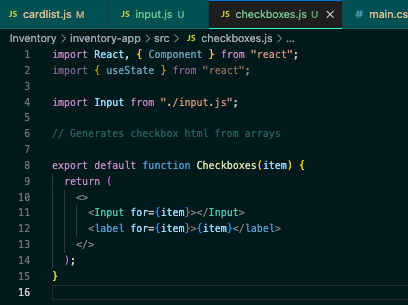
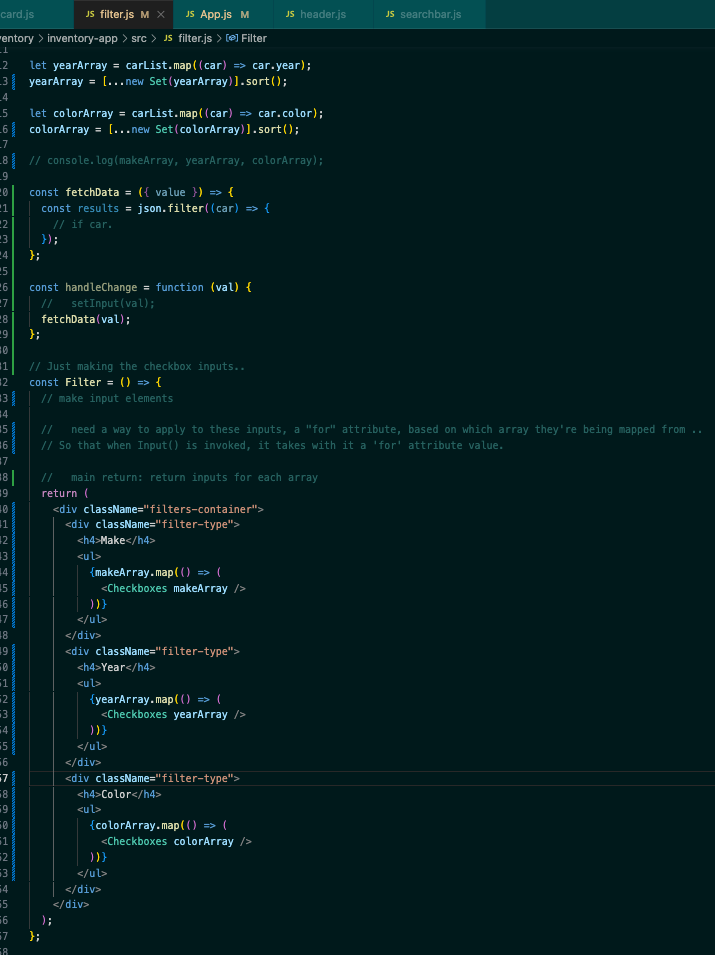