Using Buttons Properly
Hello š
So I am creating a staff application Modal, I haven't actually gotten to the modal part of things yet tho ahaha
You can find my current file structure attached in an image
This is the staffapp.js file
```
const { Events, Interaction } = require("discord.js");
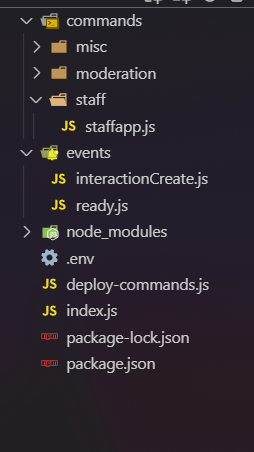
3 Replies
⢠What's your exact discord.js
npm list discord.js
and node node -v
version?
⢠Post the full error stack trace, not just the top part!
⢠Show your code!
⢠Explain what exactly your issue is.
⢠Not a discord.js issue? Check out #useful-servers.
interactionCreate.js
I was wondering how I would add buttons into this
I was thinking something like this inside the interactionCreate.js file.
But how would I actually access the button calls inside a different buttons directory? Help please
discord.js@14.11.0 and node v18.12.1
Im trying to find this in the documentation but im honestly failing to find where its stated
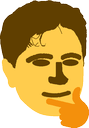
this was semi-crossposted to #djs-help-v14 but not directly answered
not sure what you intend to do after receiving the answer you were given https://discord.com/channels/222078108977594368/824411059443204127/1120858869841797200
but just to specifically answer your question here, you'd need a similar setup to the guide's command handling
just as
<Client>.commands
is a Collection you would have to define and populate, you would also have to define <Client>.buttons
and populate it yourself by iterating and reading your buttons' files