Can't read default_member_permissions from SlashCommandBuilder?
I'm building a script that documents slash command attributes for a wiki page. I have a wrapper class that holds command attributes that aren't directly used by the SlashCommandBuilder (eg cooldown, execute function).
Strangely, the
default_member_permissions
is readable inside the class, but not outside the wrapper.
For a more concrete exmaple, here's the wrapper constructor:
In the console.log, this shows default_member_permissions: '1024',
.
However, this differs from when it gets logged outside the wrapper class.
This ends up logging default_member_permissions: undefined,
. This seems especially problematic since my command uploader reads the default member permission in the same way, which would likely mean that attribute is getting stripped before getting uploaded. Am I missing something about how exporting might affect SlashCommandBuilders?
Bonus: any recommendations on converting from a permission bit field back to a human readable list of permissions?7 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.Can you show everything that's getting logged?
<SlashCommandBuilder>.toJSON().default_member_permissions
should be what you're looking for, but you may not be directly assigning every property to whatever's being logged
Bonus: <PermissionsBitField>.toArray()
returns strings like ViewChannel
Looks like the only difference is the
default_member_permissions
(omitted some looping over other commands).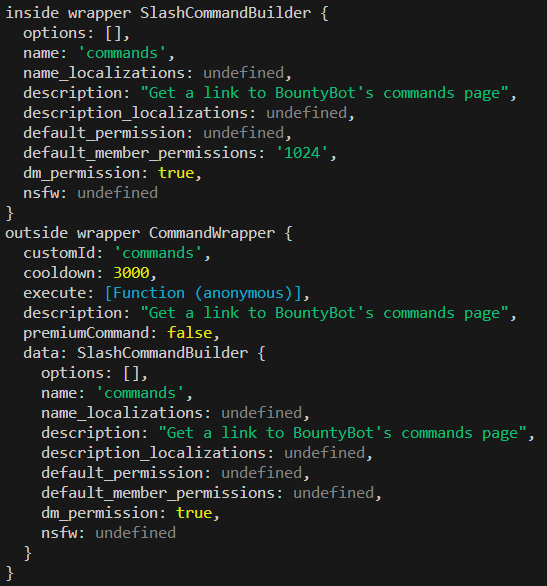
I'm guessing it has something to do with your constructor for
CommandWrapper
. It looks like you're passing in each property from the builder to the CommandWrapper class, so something might be getting lost in translation thereSeems plausible to me, but I'm stumped on what's going wrong specifically. Here's the
CommandWrapper
in its entirety:
Notably, I have it hardcoded to .setDefaultMemberPermissions(PermissionFlagsBits.ViewChannel)
to reduce complexity for debugging, but normally it's just putting defaultMemberPermission
straight in there without any modifications.The last line in the constructor is
this.data.setDefaultMemberPermissions()
. That's probably why the property is present in the log, but empty: you explicitly set it to undefined
facepalms thanks!