How to get rid of CORS errors
I keep getting cors error when I tried to connect my front and back end applications. It works on localhost but when I deployed both of them, it just fails. I did some googling and they said I should add this to my app.js
Which I did but it still doesn't work
Frontend - Nextjs,
deploy - vercel
Backend - Nodejs/express
deploy - railway
Frontend code executing the function
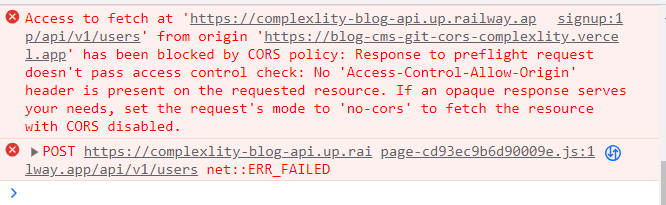
5 Replies
Update:
I tried to use render to host the api and till the same thing. I noticed it does not even show the request on the node logs.
I pinged
/api/v1/sessions
(IMAGE 1) but it still doesn't show on the logs (IMAGE 2) could it be the browser?
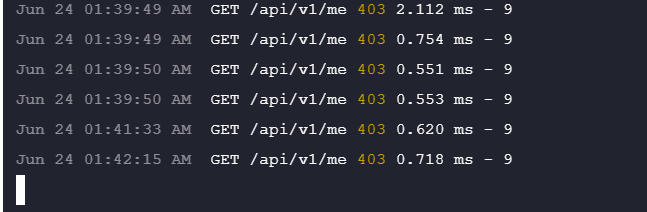
try setting origin: "*"
with a *
to allow all origins... just to see if it works
Okay 👌 . Will do
I just remember I tried that. It didn’t work because I have credentials:true. Apparently, to send credentials, you have to put some allowed domains and cannot used *
you can change your middleware so that if you hit the api endpoint it will append the headers
Oh wow. Does that work if you use a different backend server. I use node/express in this case and not the nextjs api route