How to add Many to Many relation fields in `z.object` and how to write procedure for it ?
I have created these two models
I have already created categories.
I want to write a procedure to create a product but I don't know how to add categories.
How to define categories in
z.object
do I have to add array of category_id
or something else?
Will the product will be automatically added to the category table?
having same question for other fields too i.e. skus, images...15 Replies
something like this
Prisma
Relation queries (Concepts)
Prisma Client provides convenient queries for working with relations, such as a fluent API, nested writes (transactions), nested reads and relation filters.
Thanks,
I am trying this, I will ask if have any issue
it is giving this type error
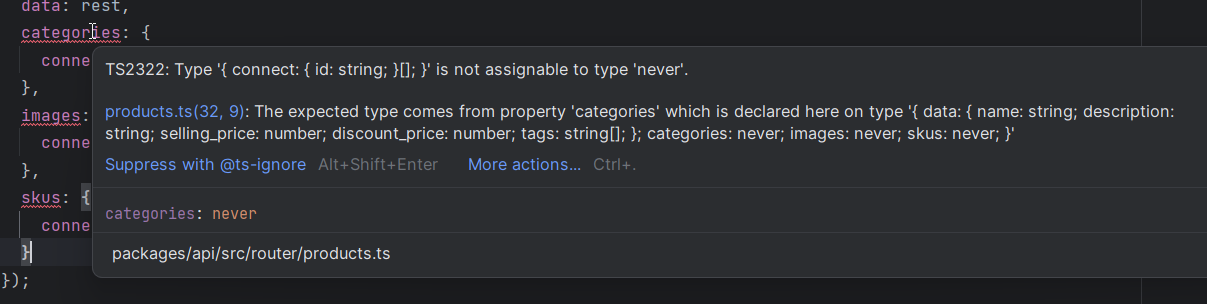
what should I do ?
you should generate the new prisma types
I ran the command to genrate prisma types
turbo db:generate
Do I have to define any types here ?
typescript does not recognize the type
you should look into it tho
here's also it's showing this type error
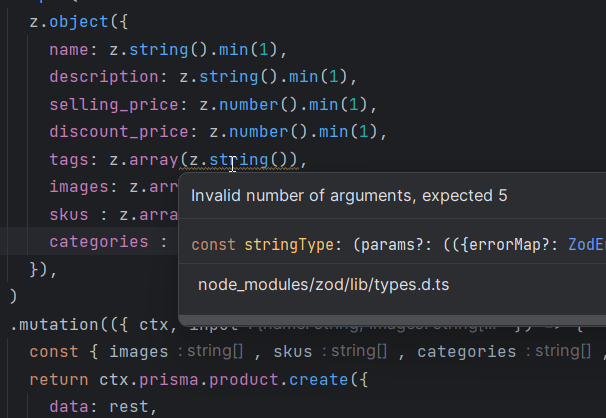
think I should
// @ts-ignore
and move on 👀yeah
turbo and prisma are weird
this feels too much illegal ðŸ˜
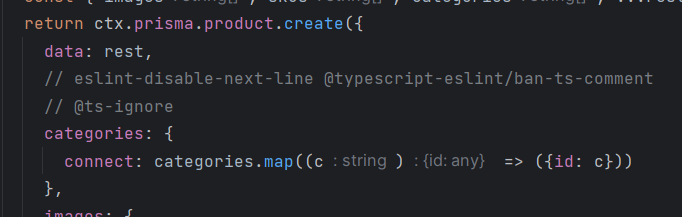
there was a syntax error
the fields should be inside
data
😅
Hi guys I have an almost identical situation and I'm also confused - when you say you have already created categories, do you mean that you created a categories table manually in Prisma Studio so that you can connect newly-created products to these existing categories? Can you explain what you mean by "I already created the categories"?