75 Replies
• What's your exact discord.js
npm list discord.js
and node node -v
version?
• Post the full error stack trace, not just the top part!
• Show your code!
• Explain what exactly your issue is.
• Not a discord.js issue? Check out #useful-servers.this is in my interaction.js file
how would i get the value of the user chosen in the command?
kick.js
the 'user'you should use this instead of listening to
interactionCreate
for the confirmation buttonsah
else, you could store the member's id in a button's custom id
hm
so
The custom id must be a string
yeah
i figured
I suggest that you use a collector
ok
ty
wait
i dont get it
should i make a new file?
No; create the collector in your command file
ah
so
wait
what
The Collector must be created in the file of your command.
yes
Glad we're on the same page
ur lecturing a gebril
where in it?
like the beggining?
the collector relies on you to have access to the message containing buttons, first
k
what do i put where
you should have enough javascript and critical thinking skills to know which variables need to be defined before/after other variables
i mean
whats message
The return value of
interaction.reply(...)
once you resolve the promiseah
so
i get message after
async execute(interaction)
right?this is how it should be defined as
await interaction.reply(
{
embeds: [embed],
components: [row],
ephemeral: true
});
like that
Yes, that function returns an object with a
.awaitMessageComponent()
methodafter thats executed?
after it sends teh embeds?
Well you can't collect interactions on a message that hasn't been sent yet
fair
so awaitMessageComponent is returned to interacts.js?
I don't understand what you mean
like
nvm
so basically
i add
componentType: ComponentType.UserSelect
you're collecting buttons, so your component type should be ComponentType.Button
which also means interaction.values
won't existbut
im trying to get the user to kick
also why is tehre a 60 second deffered responce?
Then please show me where you create a
new UserSelectMenuBuilder()
not a menu
its a subcommand
the collector is for collection message component interactions
message components are components sent on messages. they are buttons and select menus.
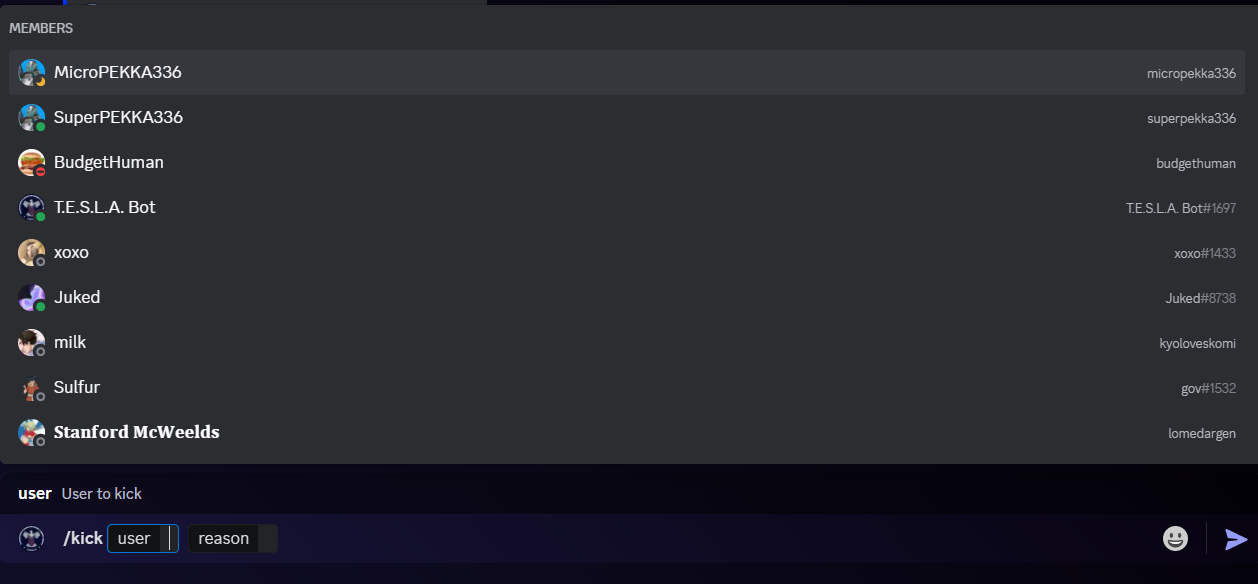
you don't need to collect which user was selected because that was handled by your slash command (chat input command), which is not a select menu
you are only collecting the button that tells you if the command user confirms the kick
correct
the
componentType
option when using collectors tells djs which type of component interactions to collect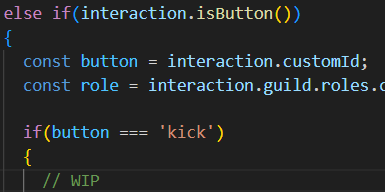
ComponentType.UserSelect
is the type for user slsect menuswait
no
ive done all this already
remind that all of this should be in your command file
right now
wait what
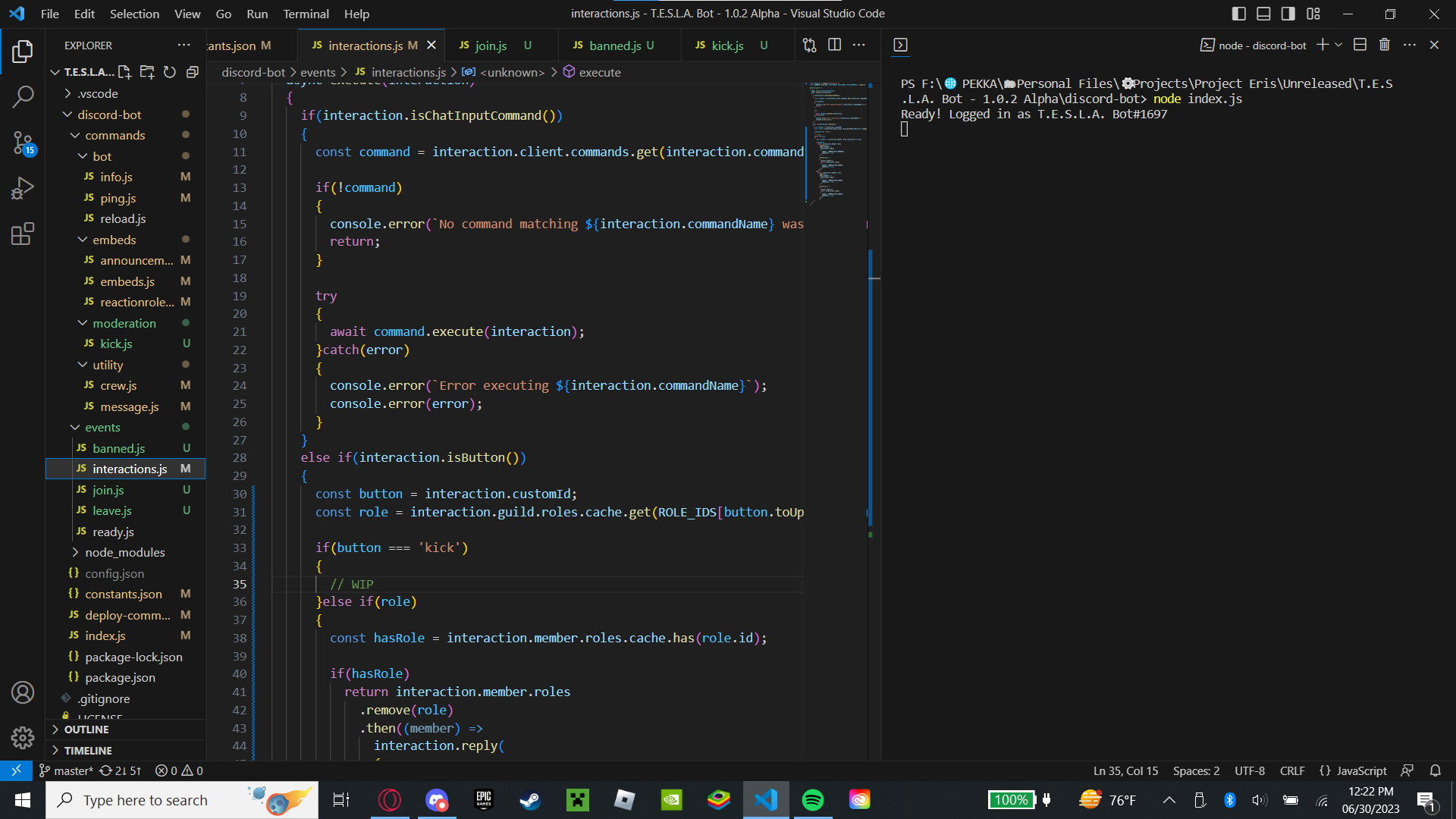
shouldnt i have a single file
taht handles ALL interactions?
*that
this file.
your command's file
yes
the confirmation buttons are entirely dependent on your command being used.
correct
Glad we're on the same page
likewise
ok new question:
How do I get the member selected in the subcommand to the interactions.js file?
the entire course of this thread has been me telling you that you don't need to go back-and-forth, and you can handle everything -- the subcommand and the confirmation buttons -- in the command file
ok
so
then i use this
so i dont need interactions.js at all?
i don't know, look at
interactions.js
and ask yourself if it's necessary for your botright now, yes
but i should move all the interactions to their respective commands?
so each command handles their own interactions?
oh a fellow Fortnite enjoyer
if you send a message component as a response to a command, and if that message component is meant to be used only temporarily (like a simple confirmation button), then I believe that it should be handled in the command's file
ah ok
bc i have reaction roels
in interactions.js
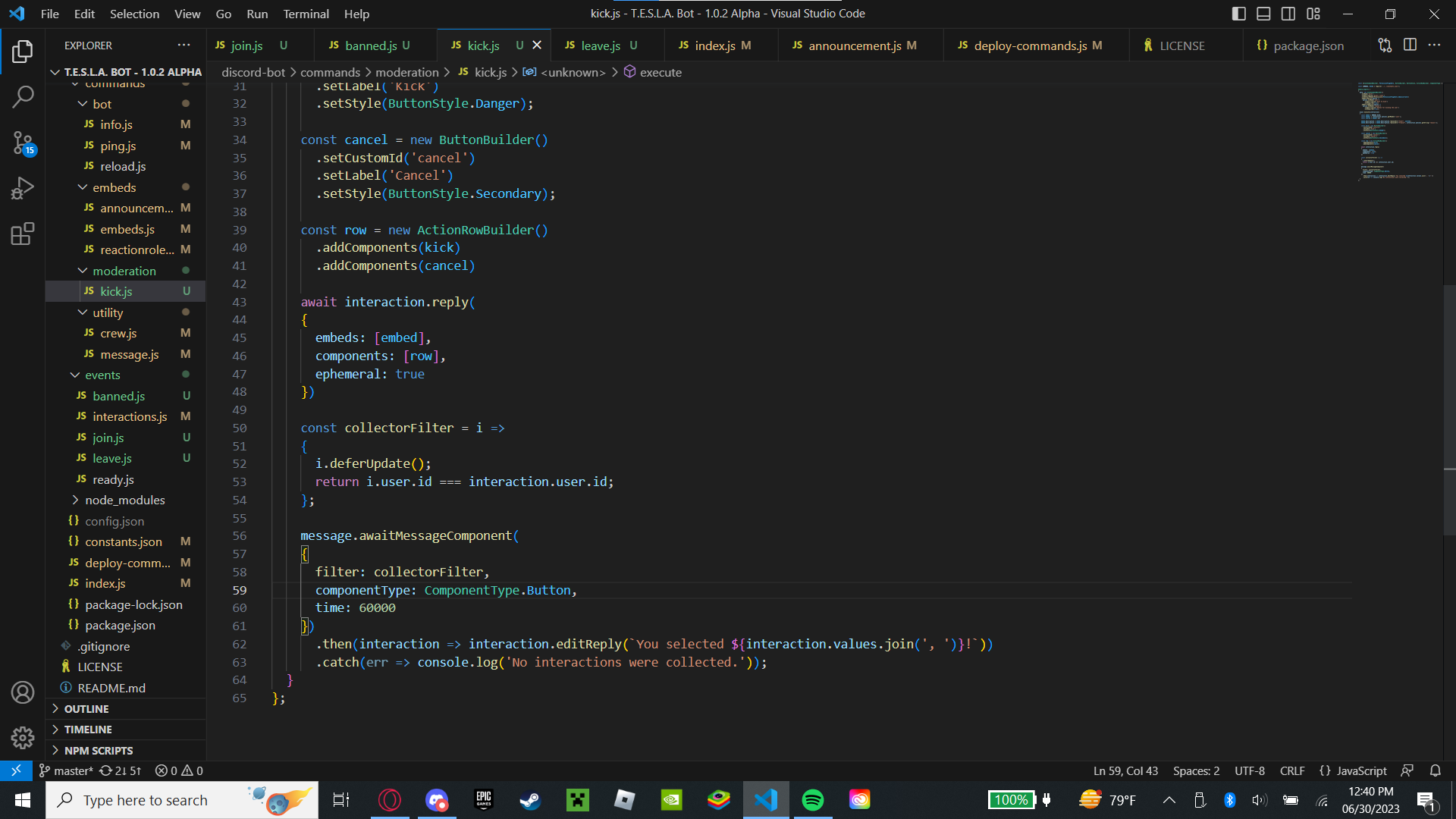
this correct?
Does it work
well
no
Then it's incorrect
well
where do i kick?
The world is your oyster. You can kick wherever you'd like.
....
"message isnt defined"
Don't worry, I told you how to define it
where
:3
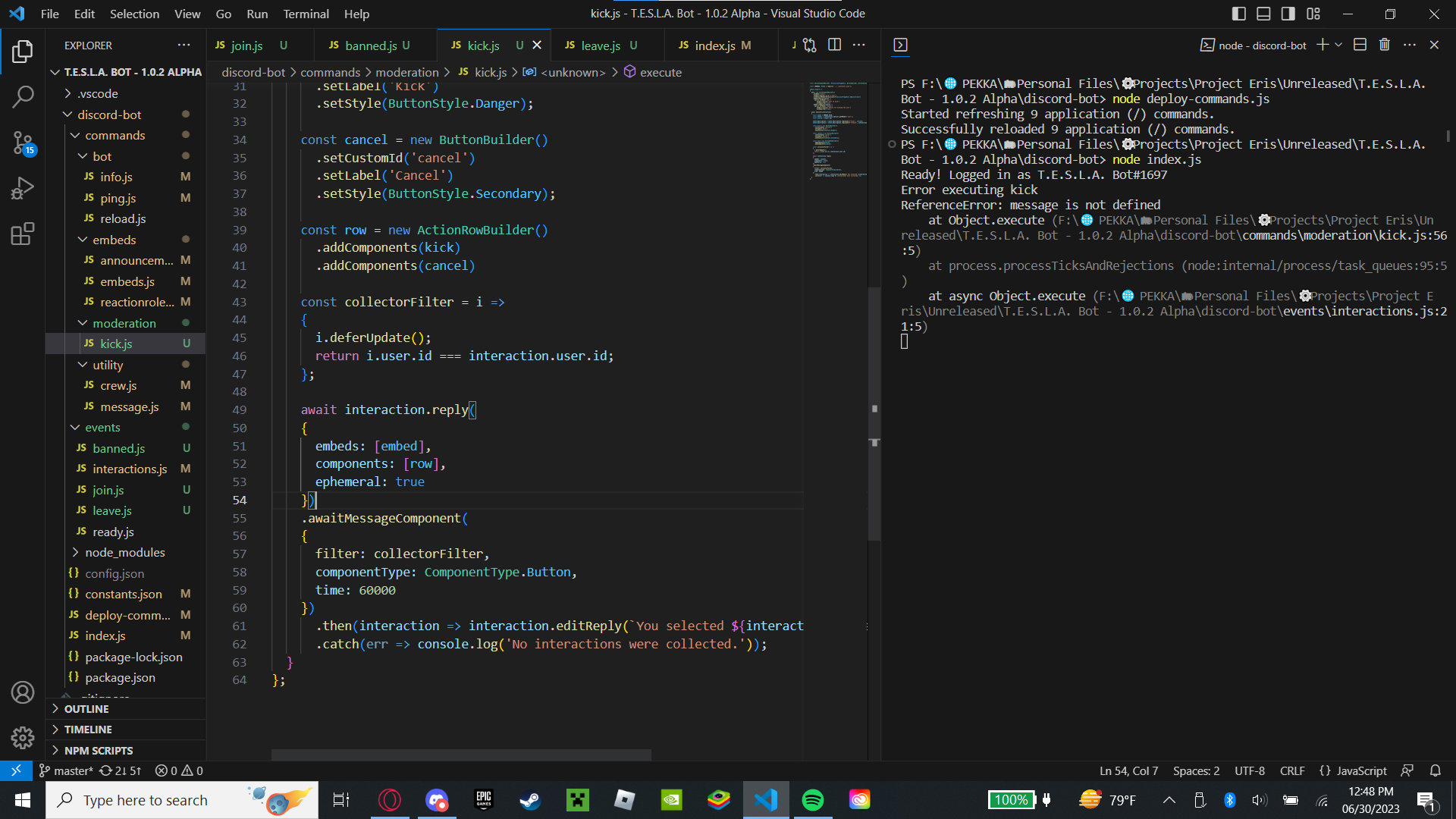
The current function chain doesn't resolve the promise that
interaction.reply()
returnshow would i
bc
yeah
how would i resolve it?
how do i fix this then?
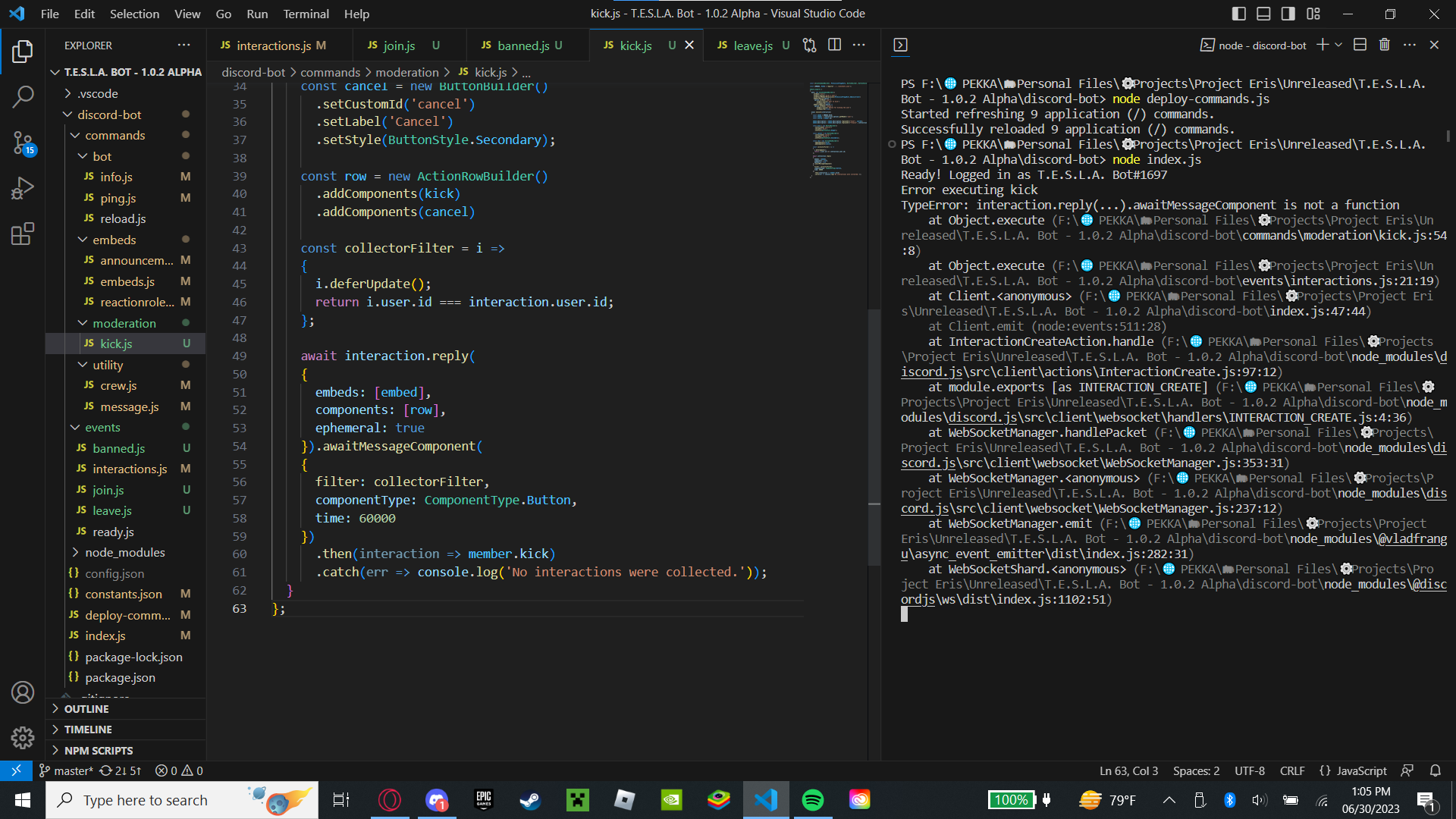
just follow the guide https://discordjs.guide/message-components/interactions.html#awaiting-components