Send setter to `props.children`
So I think this is silly, but at the same time I don't find a way out of it, so I am here asking for help.
I am trying to make a
Modal
that can accept any children
and that children will receive the setOpen
fn to open/close the modal independently.
Let's show some code:
As you can see my attempt is to generalize the Dialog
component from Shadcn/ui but I really cannot wrap my head around on how to pass setOpen
down to SpecificForm
and also make TypeScript happy.
Thanks for the help.Solution:Jump to solution
My preferred way though is to hoist the state of the modal outside of the JSX and store it in a hook.
```tsx
const useModal = () => {
const [open, setOpen] = useState(false);
...
7 Replies
One way is to make children a render prop:
Solution
My preferred way though is to hoist the state of the modal outside of the JSX and store it in a hook.
If you want even more of an abstraction, check out how we do dialogs & forms in Spacedrive:
https://github.com/spacedriveapp/spacedrive/blob/f1239d166ab5e96d5c2352d355bfe6d1f022eb59/interface/app/%24libraryId/settings/node/libraries/CreateDialog.tsx
Thanks for the quick answer, actually I thought of lifting state since in a different project (at work) I already use Zustand to handle the state of different modals, but just between you and me I don't like it much 😅
Anyway, maybe I am just confused now, but lifting this state up means that I'll have to handle the state for each modal but I believe this will be the best approach anyway.
Thanks for the support @brendonovich
what a quality 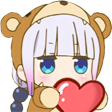
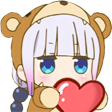
gonna keep writing essays here until Spacedrive has enough engineering blog posts that I can just link as replies
BASICALLY
I've never touched render props so its cool to see a specific use case for them