Memoize function in typescript
I want to memoize a pure function in ts that's just a static export without class. Typescript-memoize seems to need a class for the decorator to work. Do I have to wrap my functions into a class and possibly use a singleton or is there a simpler way to pull off memoization?
14 Replies
something like this?
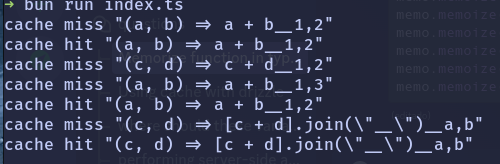
yeah I think the part I'm uncertain about is it being a class and where to save the instance and map in my t3 app but I think I'll go with something like that thanks!
you can create a context
the map would be at the context root
no need to "use a class for that"
wait. im just confused. why would you need to memoize a pure function?
heavy calculations, such as some pi digit or factorial
(I think) the goal is to not execute the function again and again, so the result is always the same since the input is the same
Oh so memorizing the results?
Not the function itself?
Yes
I mean, that's what I think. instead of calling the function it'll check for the memoized data and return the output instead
Ok. That makes more sense. Never mind
:)
for context: it's a pathfinding algorithm on a gameboard and I wouldn't want it to run on every render, so atm my approach is to save the result until a player makes another move
just trying to find the most elegant approach for that
also, my phone error corrected memoize to memorize in the title -.-
So I ended up just throwing this into a .ts file. Is it bad practice to just use globals for this kinda stuff or is it really that simple? Seems to work fine for me... @nyx (Rustular DevRel)
i mean, if it works
its fine
yeah lgtm
thanks for the advice