Setting up vanilla trpc client
So I have a button and when that button is clicked I want it to do some trpc mutation.
After doing some searching and seeing this response in a different post here https://discord.com/channels/966627436387266600/1126264396285481151/1126264611008696320 I concluded that the best way to do this was to setup a vanilla trpc client following the linked guide(https://trpc.io/docs/client/vanilla/setup)
But after following that guide I get a typescript error when I build and when I try to click the button in the dev environment I get a runtime error. So what am I doing wrong?
repo(dev branch is where stuff is): [linked repo that isn't in the state it was when I asked this]
probably relevant files:
src/pages/wip.tsx
, src/server/api/routers/itemlist.ts
and src/server/api/root.ts
Solution:Jump to solution
alright I solved this myself and yeah, I marked it as noob for a reason
so yeah(
~
is src dir not unix home dir) ~/utils/api.ts
creates the react query version of things and that version has the correct object to pass into createTRPCProxyClient
so I just copy pasted it into that resulting in this
```ts
export const vanilla_api = createTRPCProxyClient<AppRouter>({...12 Replies
typescript error:
runtime error:
reason I tagged this react query btw is because this may be an XY problem and there's actually some way using that to do what I want
Solution
alright I solved this myself and yeah, I marked it as noob for a reason
so yeah(
~
is src dir not unix home dir) ~/utils/api.ts
creates the react query version of things and that version has the correct object to pass into createTRPCProxyClient
so I just copy pasted it into that resulting in this
which seems to work for what I want/need to doi think youre misinterpeting whats being said in the linked post
like it works but you don't need the vanilla client for this
what you can do is
yeah it seems that way, it also seems that I didn't know how to use react query
it seems I can't mark your response as a solution due to marking my own thing as a solution which is annoying but oh well
thanks
@Rhys as reece feature request
Thanks for the ping
@pagwin are you wanting the ability to mark multiple responses as the solution or change the answer?
personally both seem reasonable, but the ability to change the "correct" answer seems more important
Yeah I’d agree with that, I’ve mean meaning to add support for changing the answer I’ll hopefully get to that by the end of this week
but if theres two good answers then being able to surface both to google feels cool also. definitely less important though
tbh my random complaint was not intended as a feature request, I agree with that cje said though
Well your getting features now
enjoy
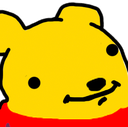
*you're
I always use it's even when I should use its so I shouldn't be throwing stones