Thats rather simple You split up the
Thats rather simple. You split up the file in chunks on the client and send it one by one
1 Reply
Part 2:
That's a code snippet from one project. Everyone: Please judge me 😁
Upload flow:
- Iterate over the queue of to-be-uploaded files (there could be multiple)
- Figure out how many chunks need to be uploaded
- Request an upload session from our backend
- Iterate over the chunk count and upload the chunk (and create checksums in this case for good measure)
What this code misses: Any form of error handling / retry logic when a chunk upload fails for some reason. To say it in Cloudflares terms: It will be added
.
Hope this can help you, @w8r .
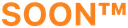