Middleware forward headers to other functions
Hello, I am building a next application and I have a middleware where I add the href in a header. Then I access it in some server components. It's working correctly on Vercel and locally but on Cloudflare, the header is not forwarded to the server components.
Do I need to add something else?
Or do you have another solution? I want to access some query params in a nextjs layout but from what I know it's not directly possible right now.
Thanks.
Example:
10 Replies
Currently trying something like this but I have a 500 when I deploy
Found a solution to my problem
I'm not sure I understand what you are trying to do. Have you tried using the same way that they demonstrate setting request headers in the docs? https://nextjs.org/docs/app/building-your-application/routing/middleware#setting-headers
Indeed and the header is not forwarded to the other functions when I deploy with @cloudflare/next-on-pages. Otherwise, locally it's works perfectly
We have tests that set request headers following the recommended way in the docs and it works fine. I have actually just tested it.
You can see it here: https://aacf0d23.nop-middleware-demo.pages.dev/api/hello?setHeader
None of the code snippets you sent are following the format in the docs. Specifically this part:
I maybe don't understand something. But I tried again and it doesn't work the same way in local and when I deploy. If you check here https://d0ba92e2.altissia-apps.pages.dev/login?interfaceLg=fr_FR I have middleware that set the header x-interface-lg and I try to access that header in the layout just after passing the middleware to set the good interface language but like this, the header is not forwarded
When you say local, I would assume you mean
next dev
? If so, that might have differences to a deployment. If you build with next-on-pages, you can try it locally with Wrangler in an environment that will be the same as when deployed to Cloudflare Pages.
Could you share the current code snippet that you are trying to use in the middleware?I didn't test it but I did a little reproduction here https://github.com/anymaniax/cloudflare-middleware-forward. I don't know how to run wrangler dev locally when I tried to do npx wrangler dev ./.vercel/output/static/_worker.js/index.js --compatibility-flags nodejs_compat I have an error
The command for locally running it is
npx wrangler pages dev .vercel/output/static --compatibility-flag=nodejs_compat
You need to change line 26 like I have in this screenshot, as it says in the Next.js docs and like I wrote above.
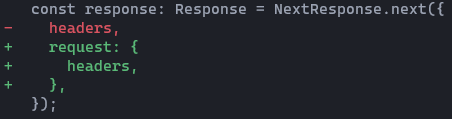

I feel stupid right now
Thanks for your time 😄
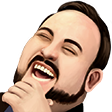