How do i fetch data from a TRPC endpoint in getStaticProps and getServerSideProps?
currently trying to do it like this
throwing an error about how i cant use the useQuery hook in getServerSideProps which makes sense yeah? so how do i do that?
57 Replies
use the server-side helpers: https://trpc.io/docs/client/nextjs/server-side-helpers
Server-Side Helpers | tRPC
The server-side helpers provides you with a set of helper functions that you can use to prefetch queries on the server. This is useful for SSG, but also for SSR if you opt not to use ssr: true.
appreciate the help.
after implementing SSH, im getting this error
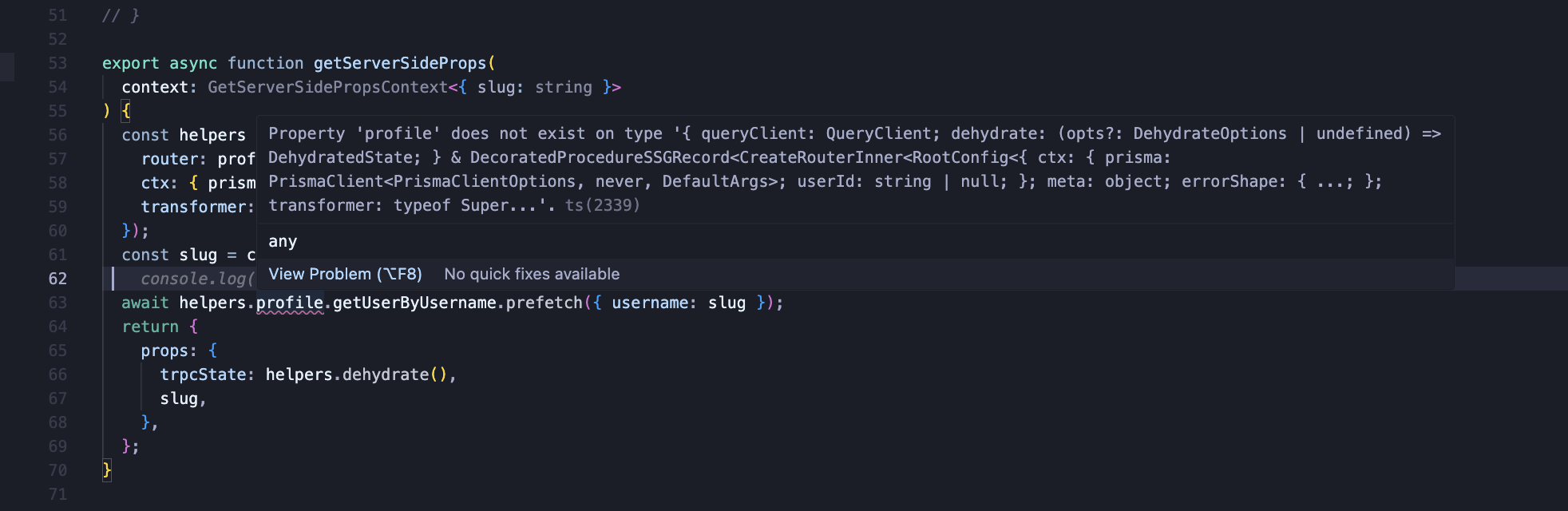
what are you passing to
router
my profile router
you probably wanna pass in your root router
yea idk what the behaviour is if you pass in a sub router
ohh from root
i thought i can pass it in directly
u might be able to and then remove the
profle.
part but idk if trpc will use the correct cache key in that caseworking now. appreciate your help
taking theos t3 course. do you have another t3 recommendation after this? trying to get very familiar with the stack
i haven't built anything with t3 haha ¯\_(ツ)_/¯
i just know a lot about the individual parts since we basically recrerated trpc in rust
oh wow. yet you know trpc so much hahaha
okay. just realised im talking to a senior
and i know way more than i should about prisma bc i made prisma client rust
nice. tried to pick up rust, was too difficult so i ended up learning golang
nooooo another person lost to go
@brendonovich Clerk is really stressing me out. Can you help with something please?
Idk much about Clerk but ask away
so here's whats happening basically.
following theos t3 tut, i had been using 1 github and 1 gmail account to sign into my app. tried to sign up as a new user now and it always defaults to my github account.
i go through all of the process of selecting the gmail account to sign in with, authorize it then it redirects me to my apps homepage with my github logged in.
jwt token is clearing from the browser so i know my initial signout is actually signing me out
lmfao just figured out whats happening
the new email im signing into is the email i used for the github account
so it's seeing them as 1 user
which is weird
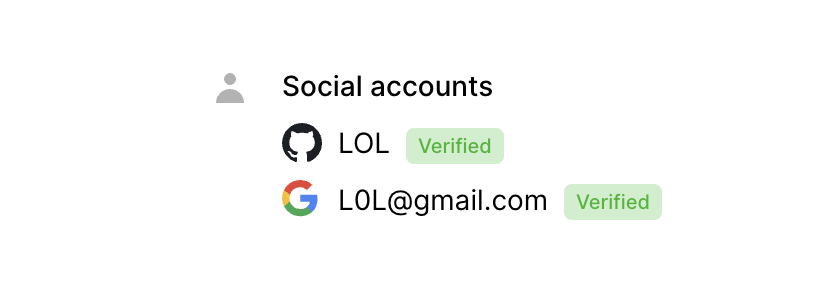
ah yea i thought that might be it haha
hello @brendonovich sorry for the occasional tags but kindly help out please.
following react query docs i'm finding it very difficult to understand or implement optimistic updates on my project
Basically when a mutation is triggered you manually update a query’s data, and if the query fails you roll it back
yes ser i know exactly thats whats happening, just dont know how from the docs.
i apologize if my questions are basic lol. i'm very junior
will post a screenshot of where i'm at now
Here's my code so far
Im confused on the following.
above is returning this error
Type 'unknown' must have a 'Symbol.iterator' method that returns an iterator.ts(2488)
this is also returning this error
'context' is possibly 'undefined'.ts(18048)
where is the context coming from?
i'd like to understand exactly what this is doing. especially how to implement the new post object because my post object should contain, createAt, title, and content and i should also be getting an author object from clerk so i'd get the profileImageUrl from it.
how do i set those to a temporary object in the newpost??
kindly help please
Type 'unknown' must have a 'Symbol.iterator' method that returns an iterator.ts(2488)
queryClient
won't know about the types of your tRPC router since it's just a React Query thing, it has no relation to tRPC. tRPC has useContext
that provides properly typed equivalents of each React Query function: https://trpc.io/docs/client/react/useContext#helpers. Would recommend using these instead of using queryClient.*
'context' is possibly 'undefined'.ts(18048)I'd just return early if
context
is undefined
how do i set those to a temporary object in the newpost??
newPost
contains all the data that's passed into the mutation, you can customise it as you wish. Idk what you'd do for the clerk stuff but yea u can chuck whatever in thereuseContext | tRPC
useContext is a hook that gives you access to helpers that let you manage the cached data of the queries you execute via @trpc/react-query. These helpers are actually thin wrappers around @tanstack/react-query's queryClient methods. If you want more in-depth information about options and usage patterns for useContext helpers than what we provide...
same error sir
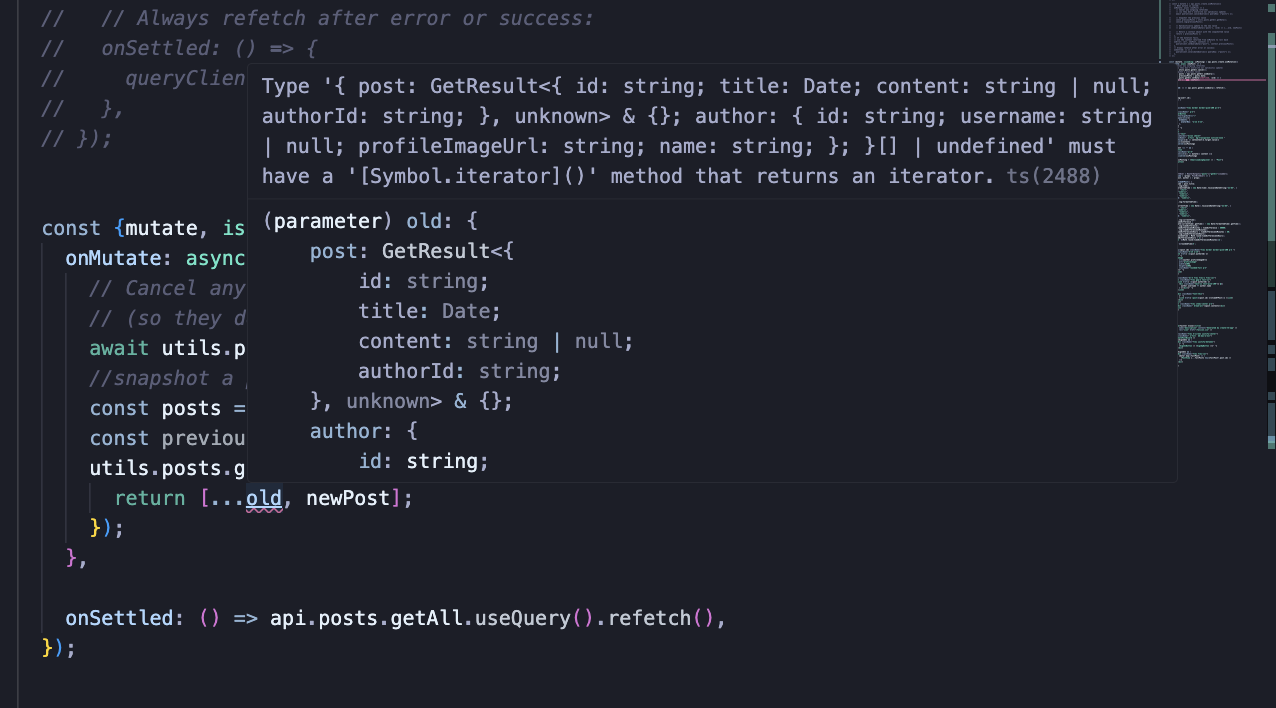
here's my code
import { api } from "~/utils/api";
const utils = api.useContext();
heres how i got both utils and api. can you explain their differences please?
Ah again just return early if old is undefined
const posts = api.posts.getAll.useQuery();
You can't just call it in the onMutate afaik?kindly explain @thatbarryguy
do you mean i should just do api.post.getAll.GetData()?
🤷 I just know you can't call a hook like that
whats the better way to call it? #noob
can you help with the problem im facing? @thatbarryguy
Barry is right in that you can’t use useQuery there - you’ve incorrectly translated the previous code you had
You should be using getData instead of useQuery since that’s the stated equivalent to getQueryData
Seems good
error
It’s hard to say since I don’t know where the error is occurring but it looks like one of your functions is returning the wrong value
this is where the error is coming from.
if you dont mind ser, i could provide the repo link.
({ …; } | { …; })[]
hints to me that the type of optimisticPost
doesn’t match the rest of the items in old
The array you’re returning could have 2 different types of elements
I see you have an OptimisticPost type, which probably isn’t a good idea since you need to use the element type of the array returned from that query
tRPC has utilities to extract return types from queries which you could use to give optimisticPost
a correct type based on the return type of the querythank you for your help so far ser
yes i did that and assigned it to optimisticPost
now i dont get any error in the console but it fetched every second so my UI glitches. i wonder if there's anything i did wrong here to cause that
i know i could just invalidate after every post but i dont wanna give up on learning optimistic updates.
onSettled will keep running the invalidate no?
no thats not it. problem persists
I’m on phone can’t clone
oh
i give up
you're defining components within components
don't do that
wut

haha
thanks for checking it out ser
fixed that
any feedback?
probably all the debugging, didnt realise
i suspect the components in components may have caused the refetching but i'm not sure
i only have loader there
i know you suggested i used trpc helper wrappers to implement it but will using react query queryClient directly work?
do i have to do it the trpc way?
it'll probably work but i'd think you'd be doing yourself a disservice not having the types available
i know the major problem right now is the fact that i'm not getting the right type for the instead of setting it to any.
here's someone that successfully implemented it.
so much
useCallback
🤦
not a knock on you, just saying hahaHere's the type he's using
oh it's not my code lol. just one i saw on github that's working
i was also wondering why he was using the callback hook a lot but what concerns me is his optimistic update query.
kindly take a look ser
is there a way to generate this type from prisma for my post query?
i mean it looks pretty normal
i don't see why you'd use a prisma type when u can use the types from your trpc router
you mean using
something like that right? i did that too
something like that right? i did that too
yea
are u having a problem with types or with the runtime logic?
this is my oldqueryData type
you know in optimistic updates you have to recreate what is happening in the object basically with this type this is my sample object to pass into the setData method but its throwing an error
what's the error?
and is it throwing an error or is typescript giving you an error?
hello sir. how do i pass data into a trpc useQuery wrapper? i'm trying to pass in refetchOnWindowFocus to my useQuery
nevermind ser. got it
noticed in trpc we pass a lot of undefined in our functions, why?