many many errors
the code:
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, SlashCommandBuilder, EmbedBuilder } = require('discord.js');
const { ActivityType } = require('discord.js');
module.exports = {
cooldown: 120,
data: new SlashCommandBuilder()
.setName('status')
.setDescription('Set the bot\'s status')
.addStringOption(option =>
option.setName('input')
.setDescription('The status to set')
.setMaxLength(128)
.setRequired(true)),
category: 'dev',
async execute(interaction) {
const status = interaction.options.getString('input');
const cancel = new ButtonBuilder()
.setCustomId('Cancel')
.setLabel('Cancel')
.setStyle(ButtonStyle.Secondary);
const playing = new ButtonBuilder()
.setCustomId('Playing')
.setLabel('Playing')
.setStyle(ButtonStyle.Primary);
const watching = new ButtonBuilder()
.setCustomId('Watching')
.setLabel('Watching')
.setStyle(ButtonStyle.Primary);
const listening = new ButtonBuilder()
.setCustomId('Listening')
.setLabel('Listening')
.setStyle(ButtonStyle.Primary);
const reset = new ButtonBuilder()
.setCustomId('Reset')
.setLabel('Reset')
.setStyle(ButtonStyle.Danger);
const row = new ActionRowBuilder()
.addComponents(cancel, playing, watching, listening, reset);
const content = `\`/status\` from ${interaction.user}`;
const type = interaction.customId;
const preType = new EmbedBuilder()
.setColor(0x2b2d31)
.setTitle('_No status type, moo?_ 🐄')
.setDescription(`**Status:** ${status}\n\nPlease pick a type for this status!`);
const selectType = EmbedBuilder.from(preType)
.setTitle('_Successful moo!_ 🐄')
.setDescription(`**Status:** ${status}\n**Type:** ${type}\n\nI have set the status!`)
.setFooter({ text: 'To set a different status, use /status again.' });
const resetType = EmbedBuilder.from(preType)
.setDescription('**Status:** reset\n\nI have reset the status!')
.setFooter({ text: 'To set another status, use /status again.' });
const response = await interaction.reply({
embeds: [preType], content: `${content}`, fetchReply: true,
components: [row],
});
const collectorFilter = i => i.user.id === interaction.user.id;
const selection = await response.awaitMessageComponent({ filter: collectorFilter });
if (selection.customId === 'Cancel') {
await response.suppressEmbeds();
await interaction.editReply({ content: '`/status` type selection cancelled.', components: [] });
}
else if (selection.customId === 'Reset') {
await interaction.client.user.setActivity(null);
await interaction.editReply({ embeds: [resetType], content: `${content}`, components: [] });
}
else if (selection.customId === 'Playing') {
await interaction.client.user.setActivity(`${status}`, { type: ActivityType.Playing });
await interaction.editReply({ embeds: [selectType], content: `${content}`, components: [] });
}
else if (selection.customId === 'Watching') {
await interaction.client.user.setActivity(`${status}`, { type: ActivityType.Watching });
await interaction.editReply({ embeds: [selectType], content: `${content}`, components: [] });
}
else if (selection.customId === 'Listening') {
await interaction.client.user.setActivity(`${status}`, { type: ActivityType.Listening });
await interaction.editReply({ embeds: [selectType], content: `${content}`, components: [] });
}
},
};
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, SlashCommandBuilder, EmbedBuilder } = require('discord.js');
const { ActivityType } = require('discord.js');
module.exports = {
cooldown: 120,
data: new SlashCommandBuilder()
.setName('status')
.setDescription('Set the bot\'s status')
.addStringOption(option =>
option.setName('input')
.setDescription('The status to set')
.setMaxLength(128)
.setRequired(true)),
category: 'dev',
async execute(interaction) {
const status = interaction.options.getString('input');
const cancel = new ButtonBuilder()
.setCustomId('Cancel')
.setLabel('Cancel')
.setStyle(ButtonStyle.Secondary);
const playing = new ButtonBuilder()
.setCustomId('Playing')
.setLabel('Playing')
.setStyle(ButtonStyle.Primary);
const watching = new ButtonBuilder()
.setCustomId('Watching')
.setLabel('Watching')
.setStyle(ButtonStyle.Primary);
const listening = new ButtonBuilder()
.setCustomId('Listening')
.setLabel('Listening')
.setStyle(ButtonStyle.Primary);
const reset = new ButtonBuilder()
.setCustomId('Reset')
.setLabel('Reset')
.setStyle(ButtonStyle.Danger);
const row = new ActionRowBuilder()
.addComponents(cancel, playing, watching, listening, reset);
const content = `\`/status\` from ${interaction.user}`;
const type = interaction.customId;
const preType = new EmbedBuilder()
.setColor(0x2b2d31)
.setTitle('_No status type, moo?_ 🐄')
.setDescription(`**Status:** ${status}\n\nPlease pick a type for this status!`);
const selectType = EmbedBuilder.from(preType)
.setTitle('_Successful moo!_ 🐄')
.setDescription(`**Status:** ${status}\n**Type:** ${type}\n\nI have set the status!`)
.setFooter({ text: 'To set a different status, use /status again.' });
const resetType = EmbedBuilder.from(preType)
.setDescription('**Status:** reset\n\nI have reset the status!')
.setFooter({ text: 'To set another status, use /status again.' });
const response = await interaction.reply({
embeds: [preType], content: `${content}`, fetchReply: true,
components: [row],
});
const collectorFilter = i => i.user.id === interaction.user.id;
const selection = await response.awaitMessageComponent({ filter: collectorFilter });
if (selection.customId === 'Cancel') {
await response.suppressEmbeds();
await interaction.editReply({ content: '`/status` type selection cancelled.', components: [] });
}
else if (selection.customId === 'Reset') {
await interaction.client.user.setActivity(null);
await interaction.editReply({ embeds: [resetType], content: `${content}`, components: [] });
}
else if (selection.customId === 'Playing') {
await interaction.client.user.setActivity(`${status}`, { type: ActivityType.Playing });
await interaction.editReply({ embeds: [selectType], content: `${content}`, components: [] });
}
else if (selection.customId === 'Watching') {
await interaction.client.user.setActivity(`${status}`, { type: ActivityType.Watching });
await interaction.editReply({ embeds: [selectType], content: `${content}`, components: [] });
}
else if (selection.customId === 'Listening') {
await interaction.client.user.setActivity(`${status}`, { type: ActivityType.Listening });
await interaction.editReply({ embeds: [selectType], content: `${content}`, components: [] });
}
},
};
5 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!the error(s):
DiscordAPIError[40060]: Interaction has already been acknowledged.
at handleErrors (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:640:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:736:23)
at async REST.request (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:1387:22)
at async ChatInputCommandInteraction.reply (C:\NodeJS\npm\.moo-client\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:111:5)
at async Client.<anonymous> (C:\NodeJS\npm\.moo-client\index.js:73:3) {
requestBody: { files: [], json: { type: 4, data: [Object] } },
rawError: {
message: 'Interaction has already been acknowledged.',
code: 40060
},
code: 40060,
status: 400,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1134734765275226173/aW50ZXJhY3Rpb246MTEzNDczNDc2NTI3NTIyNjE3MzphQ0l4VzZVYnllS1pGdEdybmdVWDkzOWs4UUNNNmk2ZEVvS0hyT3R6Q2FraFhuUE05SXFlNUxKSndKNzFEN0IyVjZtUVI2YVptTHBPTWtPejNkTVNTQ1hHeDFjR09TRXZXUG9VYWJWYVBPVFF0YjZlbkhtSHhveXdDaGNkWlVYMg/callback'
}
node:events:492
throw er; // Unhandled 'error' event
^
DiscordAPIError[40060]: Interaction has already been acknowledged.
at handleErrors (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:640:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:736:23)
at async REST.request (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:1387:22)
at async ChatInputCommandInteraction.reply (C:\NodeJS\npm\.moo-client\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:111:5)
at async Client.<anonymous> (C:\NodeJS\npm\.moo-client\index.js:81:4)
Emitted 'error' event on Client instance at:
at emitUnhandledRejectionOrErr (node:events:395:10)
at process.processTicksAndRejections (node:internal/process/task_queues:84:21) {
requestBody: {
files: [],
json: {
type: 4,
data: {
content: 'There was an error while executing this command!',
tts: false,
nonce: undefined,
embeds: undefined,
components: undefined,
username: undefined,
avatar_url: undefined,
allowed_mentions: undefined,
flags: 64,
message_reference: undefined,
attachments: undefined,
sticker_ids: undefined,
thread_name: undefined
}
}
},
rawError: {
message: 'Interaction has already been acknowledged.',
code: 40060
},
code: 40060,
status: 400,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1134734765275226173/aW50ZXJhY3Rpb246MTEzNDczNDc2NTI3NTIyNjE3MzphQ0l4VzZVYnllS1pGdEdybmdVWDkzOWs4UUNNNmk2ZEVvS0hyT3R6Q2FraFhuUE05SXFlNUxKSndKNzFEN0IyVjZtUVI2YVptTHBPTWtPejNkTVNTQ1hHeDFjR09TRXZXUG9VYWJWYVBPVFF0YjZlbkhtSHhveXdDaGNkWlVYMg/callback'
}
Node.js v18.17.0
[nodemon] app crashed - waiting for file changes before starting...
DiscordAPIError[40060]: Interaction has already been acknowledged.
at handleErrors (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:640:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:736:23)
at async REST.request (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:1387:22)
at async ChatInputCommandInteraction.reply (C:\NodeJS\npm\.moo-client\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:111:5)
at async Client.<anonymous> (C:\NodeJS\npm\.moo-client\index.js:73:3) {
requestBody: { files: [], json: { type: 4, data: [Object] } },
rawError: {
message: 'Interaction has already been acknowledged.',
code: 40060
},
code: 40060,
status: 400,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1134734765275226173/aW50ZXJhY3Rpb246MTEzNDczNDc2NTI3NTIyNjE3MzphQ0l4VzZVYnllS1pGdEdybmdVWDkzOWs4UUNNNmk2ZEVvS0hyT3R6Q2FraFhuUE05SXFlNUxKSndKNzFEN0IyVjZtUVI2YVptTHBPTWtPejNkTVNTQ1hHeDFjR09TRXZXUG9VYWJWYVBPVFF0YjZlbkhtSHhveXdDaGNkWlVYMg/callback'
}
node:events:492
throw er; // Unhandled 'error' event
^
DiscordAPIError[40060]: Interaction has already been acknowledged.
at handleErrors (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:640:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:736:23)
at async REST.request (C:\NodeJS\npm\.moo-client\node_modules\@discordjs\rest\dist\index.js:1387:22)
at async ChatInputCommandInteraction.reply (C:\NodeJS\npm\.moo-client\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:111:5)
at async Client.<anonymous> (C:\NodeJS\npm\.moo-client\index.js:81:4)
Emitted 'error' event on Client instance at:
at emitUnhandledRejectionOrErr (node:events:395:10)
at process.processTicksAndRejections (node:internal/process/task_queues:84:21) {
requestBody: {
files: [],
json: {
type: 4,
data: {
content: 'There was an error while executing this command!',
tts: false,
nonce: undefined,
embeds: undefined,
components: undefined,
username: undefined,
avatar_url: undefined,
allowed_mentions: undefined,
flags: 64,
message_reference: undefined,
attachments: undefined,
sticker_ids: undefined,
thread_name: undefined
}
}
},
rawError: {
message: 'Interaction has already been acknowledged.',
code: 40060
},
code: 40060,
status: 400,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1134734765275226173/aW50ZXJhY3Rpb246MTEzNDczNDc2NTI3NTIyNjE3MzphQ0l4VzZVYnllS1pGdEdybmdVWDkzOWs4UUNNNmk2ZEVvS0hyT3R6Q2FraFhuUE05SXFlNUxKSndKNzFEN0IyVjZtUVI2YVptTHBPTWtPejNkTVNTQ1hHeDFjR09TRXZXUG9VYWJWYVBPVFF0YjZlbkhtSHhveXdDaGNkWlVYMg/callback'
}
Node.js v18.17.0
[nodemon] app crashed - waiting for file changes before starting...
it works except for when it doesn't and when it messes up it shows this old message that i used to have it set as from #how to make this concept work?
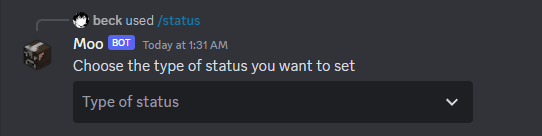
when i reset the bot, it shows what it's supposed to & functions as supposed to, except for the type which i messed up on (and i ran into this issue cuz i could not get it to show the custom ID properly in the first place, idk if that's the only issue anymore)
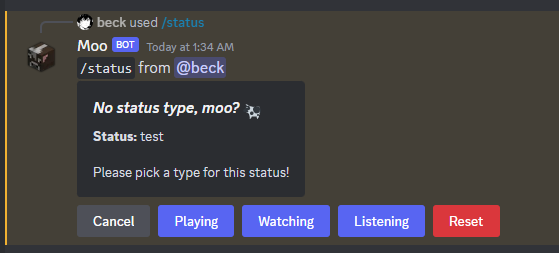
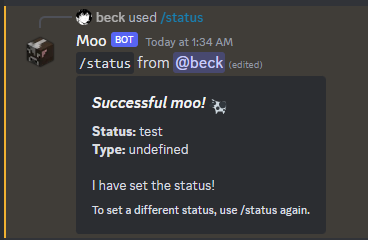
idk atp
it keeps giving me shit about how the interaction has already been acknowledged, method isn't allowed etc, shows the old message layout upon error even though it's not there anymore, i keep changing stuff but i can't figure it out. it's probably silly to put this much time into it but like it is possible right? with the buttons? 😭 i don't even know
thank you i was so distressed