Problem with AuditLogs (MessageDelete)
I have some problem. If some other person deletes my message, and then I delete my messages, it will write that he deleted my messages. How to fix it?
10 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!because you're fetching the latest log and deleting your own message doesn't create a log. you could create a work around by checking if the log was created in the past 5 seconds
Can you please suggest how to do this? I don't understand
you could check if your
auditLogEntry
createdTimestamp was in the last 5 seconds and if it isn't return the author of the message but this could have some flaws like for example if a bot deletes a message it could confuse that with the author deleting their own messageIt works the first few times, but then it doesn't detect
up
the code is fetching the latest audit log entry for any message deletions that have occurred after the creation of the message you're checking, regardless of who deleted it so if someone else deletes your message before you delete it yourself, the audit log entry for the other person's deletion will be returned instead of the entry for your own deletion
you need to modify the code to filter out any audit log entries that do not correspond to your own deletion of the message
you can do this is to add an additional check that verifies that the audit log entry was generated by your own deletion of the message
i added an additional check that verifies that the
executor
of the audit log entry (i.e., the user who performed the action that generated the entry) is the same as the author
of the message
this ensures that the audit log entry corresponds to your own deletion of the message, rather than someone else's deletion.? an entry isnt made in the audit log when a user deletes their own message
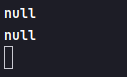
up