Pressing button results in TypeError: Cannot read properties of undefined (reading 'execute')
I will send the code for my interactionCreator.js, bot.js and deleteAll.js files below
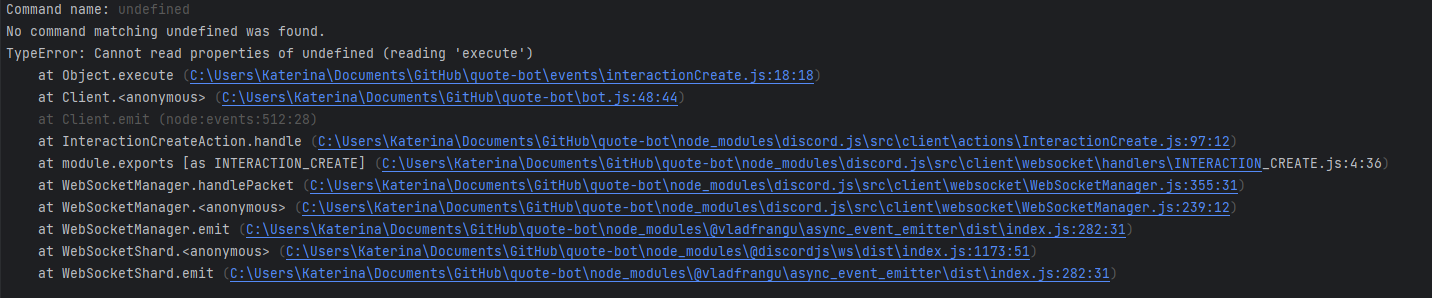
2 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!deleteAll.js
interactionCreate.js
bot.js
I have 3 other commands that all get registered and work correctly, however they do not have buttons or confirmation so I am confused
when I add this, it breaks all my other commands, so I had to remove it
I also have context menu commands,
one sec I will add it and show you
okay
module.exports = {
name: 'deleteall',
data: new SlashCommandBuilder()
.setName('deleteall')
.setDescription('Delete all quotes'),
async execute(interaction) {
if (!interaction.member.permissionsIn(interaction.channel).has(PermissionsBitField.Flags.Administrator)) {
return interaction.reply('You do not have permission to use this command');
}
const confirm = new ButtonBuilder()
.setCustomId('confirm')
.setLabel('Confirm deletion')
.setStyle(ButtonStyle.Danger);
const cancel = new ButtonBuilder()
.setCustomId('cancel')
.setLabel('Cancel')
.setStyle(ButtonStyle.Secondary);
const row = new ActionRowBuilder()
.addComponents(cancel, confirm);
const response = await interaction.reply({
content: 'Are you sure you want to delete all quotes?',
components: [row],
});
const collectorFilter = i => i.user.id === interaction.user.id;
try {
const confirmation = await response.awaitMessageComponent({ filter: collectorFilter, time: 20000 });
if (confirmation.customId === 'confirm') {
await interaction.editReply({ content: 'confirm', components: [] });
await confirmation.update({ content: 'Confirmed deletion', components: [] });
}
else if (confirmation.customId === 'cancel') {
await interaction.editReply({ content: 'Cancelled deletion', components: [] });
await confirmation.update({ content: 'Cancelled deletion', components: [] });
}
}
catch (error) {
await interaction.editReply({ content: 'Confirmation times out. Deletion aborted.', components: [] });
}
},
};
module.exports = {
name: 'deleteall',
data: new SlashCommandBuilder()
.setName('deleteall')
.setDescription('Delete all quotes'),
async execute(interaction) {
if (!interaction.member.permissionsIn(interaction.channel).has(PermissionsBitField.Flags.Administrator)) {
return interaction.reply('You do not have permission to use this command');
}
const confirm = new ButtonBuilder()
.setCustomId('confirm')
.setLabel('Confirm deletion')
.setStyle(ButtonStyle.Danger);
const cancel = new ButtonBuilder()
.setCustomId('cancel')
.setLabel('Cancel')
.setStyle(ButtonStyle.Secondary);
const row = new ActionRowBuilder()
.addComponents(cancel, confirm);
const response = await interaction.reply({
content: 'Are you sure you want to delete all quotes?',
components: [row],
});
const collectorFilter = i => i.user.id === interaction.user.id;
try {
const confirmation = await response.awaitMessageComponent({ filter: collectorFilter, time: 20000 });
if (confirmation.customId === 'confirm') {
await interaction.editReply({ content: 'confirm', components: [] });
await confirmation.update({ content: 'Confirmed deletion', components: [] });
}
else if (confirmation.customId === 'cancel') {
await interaction.editReply({ content: 'Cancelled deletion', components: [] });
await confirmation.update({ content: 'Cancelled deletion', components: [] });
}
}
catch (error) {
await interaction.editReply({ content: 'Confirmation times out. Deletion aborted.', components: [] });
}
},
};
const { Events } = require('discord.js');
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
console.log('Interaction received:', interaction);
console.log('Command name:', interaction.commandName);
const command = interaction.client.commands.get(interaction.commandName);
// If the command doesn't exist
if (!command) {
console.error(`No command matching ${interaction.commandName} was found.`);
}
try {
// Executes command
await command.execute(interaction);
}
catch (error) {
console.error(error);
if (interaction.replied || interaction.deferred) {
await interaction.followUp({ content: 'There was an error while executing this command!', ephemeral: true });
}
else {
await interaction.reply({ content: 'There was an error while executing this command!', ephemeral: true });
}
}
},
};
const { Events } = require('discord.js');
module.exports = {
name: Events.InteractionCreate,
async execute(interaction) {
console.log('Interaction received:', interaction);
console.log('Command name:', interaction.commandName);
const command = interaction.client.commands.get(interaction.commandName);
// If the command doesn't exist
if (!command) {
console.error(`No command matching ${interaction.commandName} was found.`);
}
try {
// Executes command
await command.execute(interaction);
}
catch (error) {
console.error(error);
if (interaction.replied || interaction.deferred) {
await interaction.followUp({ content: 'There was an error while executing this command!', ephemeral: true });
}
else {
await interaction.reply({ content: 'There was an error while executing this command!', ephemeral: true });
}
}
},
};
// Discord.js
const { Client, Collection, GatewayIntentBits } = require('discord.js');
const fs = require('fs');
const path = require('path');
const dbFunc = require('./database/dbFunc');
// Config
// Token
require('dotenv').config();
const token = process.env.DISCORD_TOKEN;
// New Client instance
const client = new Client({ intents: [GatewayIntentBits.Guilds] });
// Commands
client.commands = new Collection();
const foldersPath = path.join(__dirname, 'commands');
const commandFolders = fs.readdirSync(foldersPath);
// Gets all command files from command folder
// Returns error if command file is incomplete
for (const folder of commandFolders) {
const commandsPath = path.join(foldersPath, folder);
const commandFiles = fs.readdirSync(commandsPath).filter(file => file.endsWith('.js'));
for (const file of commandFiles) {
const filePath = path.join(commandsPath, file);
const command = require(filePath);
if ('data' in command && 'execute' in command) {
client.commands.set(command.data.name, command);
}
else {
console.log(`[WARNING] The command at ${filePath} is missing a required "data" or "execute" property.`);
}
}
}
// Read event files
const eventsPath = path.join(__dirname, 'events');
const eventFiles = fs.readdirSync(eventsPath).filter(file => file.endsWith('.js'));
for (const file of eventFiles) {
const filePath = path.join(eventsPath, file);
const event = require(filePath);
if (event.once) {
client.once(event.name, (...args) => event.execute(...args));
}
else {
client.on(event.name, (...args) => event.execute(...args));
}
}
dbFunc.connectDatabase().then(() => {
// Log in w/ client token
client.login(token);
}).catch((error) => {
console.error('Error: ', error);
process.exit(1);
});
process.on('beforeExit', () => {
dbFunc.dbClient.close().then(() => console.log('Shutting down...'));
});
// Discord.js
const { Client, Collection, GatewayIntentBits } = require('discord.js');
const fs = require('fs');
const path = require('path');
const dbFunc = require('./database/dbFunc');
// Config
// Token
require('dotenv').config();
const token = process.env.DISCORD_TOKEN;
// New Client instance
const client = new Client({ intents: [GatewayIntentBits.Guilds] });
// Commands
client.commands = new Collection();
const foldersPath = path.join(__dirname, 'commands');
const commandFolders = fs.readdirSync(foldersPath);
// Gets all command files from command folder
// Returns error if command file is incomplete
for (const folder of commandFolders) {
const commandsPath = path.join(foldersPath, folder);
const commandFiles = fs.readdirSync(commandsPath).filter(file => file.endsWith('.js'));
for (const file of commandFiles) {
const filePath = path.join(commandsPath, file);
const command = require(filePath);
if ('data' in command && 'execute' in command) {
client.commands.set(command.data.name, command);
}
else {
console.log(`[WARNING] The command at ${filePath} is missing a required "data" or "execute" property.`);
}
}
}
// Read event files
const eventsPath = path.join(__dirname, 'events');
const eventFiles = fs.readdirSync(eventsPath).filter(file => file.endsWith('.js'));
for (const file of eventFiles) {
const filePath = path.join(eventsPath, file);
const event = require(filePath);
if (event.once) {
client.once(event.name, (...args) => event.execute(...args));
}
else {
client.on(event.name, (...args) => event.execute(...args));
}
}
dbFunc.connectDatabase().then(() => {
// Log in w/ client token
client.login(token);
}).catch((error) => {
console.error('Error: ', error);
process.exit(1);
});
process.on('beforeExit', () => {
dbFunc.dbClient.close().then(() => console.log('Shutting down...'));
});