Why the infered type of this function is `string | undefined`?
Why the infered type of this function is
string | undefined
? How TypeScript is unable to see that the returned value can never be undefined
?
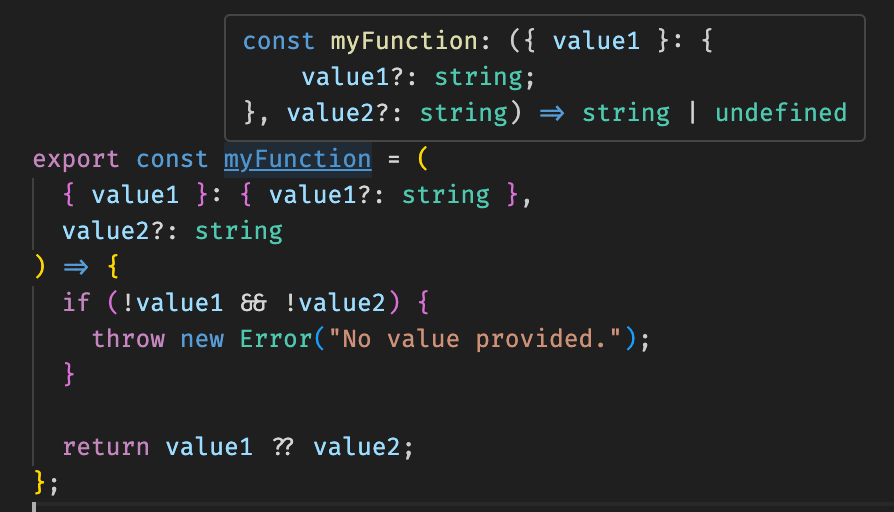
18 Replies
Even a simple version returns
string | undefined
:
this should be
then it'd be fine
Nope, you're changing the logic, I only want to throw if neither are defined (= both undefined). That's why TS should know at least one of them is defined and therefore the result is never
undefined
.hmmm
I have to add
as string
and I hate that 😢Its one of those cases where you cant get it to be inferred. The way it infers it technically is correct but I think as string is the only way.
how about
It was not exactly my use case, I am calling another function that takes a
string
as parameter. It works but it means I have to repeat the function call twice. Not ideal but maybe better than as string
, I don't know. I guess that's the 2 solutions. ¯\_(ツ)_/¯or you just use this as a separate function and then do something like this
yeah...
Not the best, but yeah
Yeah I think the
as string
is the most readable 😅Yup
My post purpose was mainly to understand why TypeScript doesn't understand
Because to the type system after the first if check, both are typed as string | undefined.
and it cant pickup on the fact that if value1 is undefined, value2 has to be defined.
Yeah, because it cant know which of them is not undefined.
but why if I only have one condition (
value !== undefined
), it manages, but not 2?because then it's logical what changed