How to display register/signin errors on the client from a server action?
I'm using a server action on a register page to conditionally create db entries for a new user, and send out a verification email.
My question is: How can I display an error on the register form like: "that username is already taken," based on the internal logic of the server action.
Attached are my form and the server action respectively
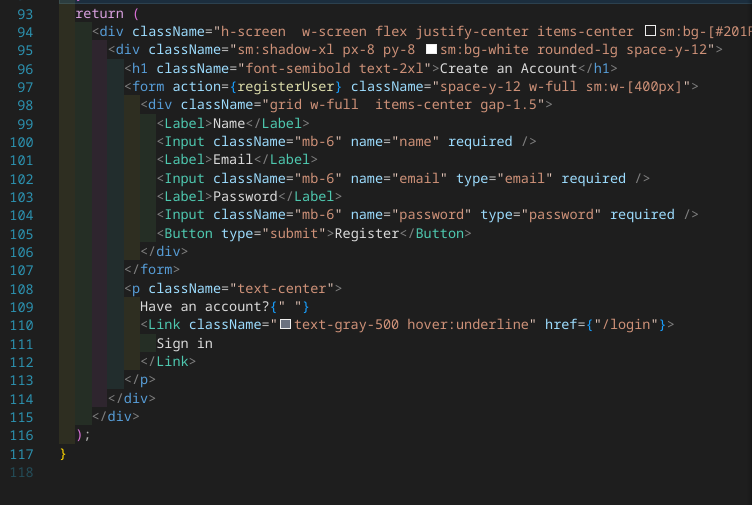
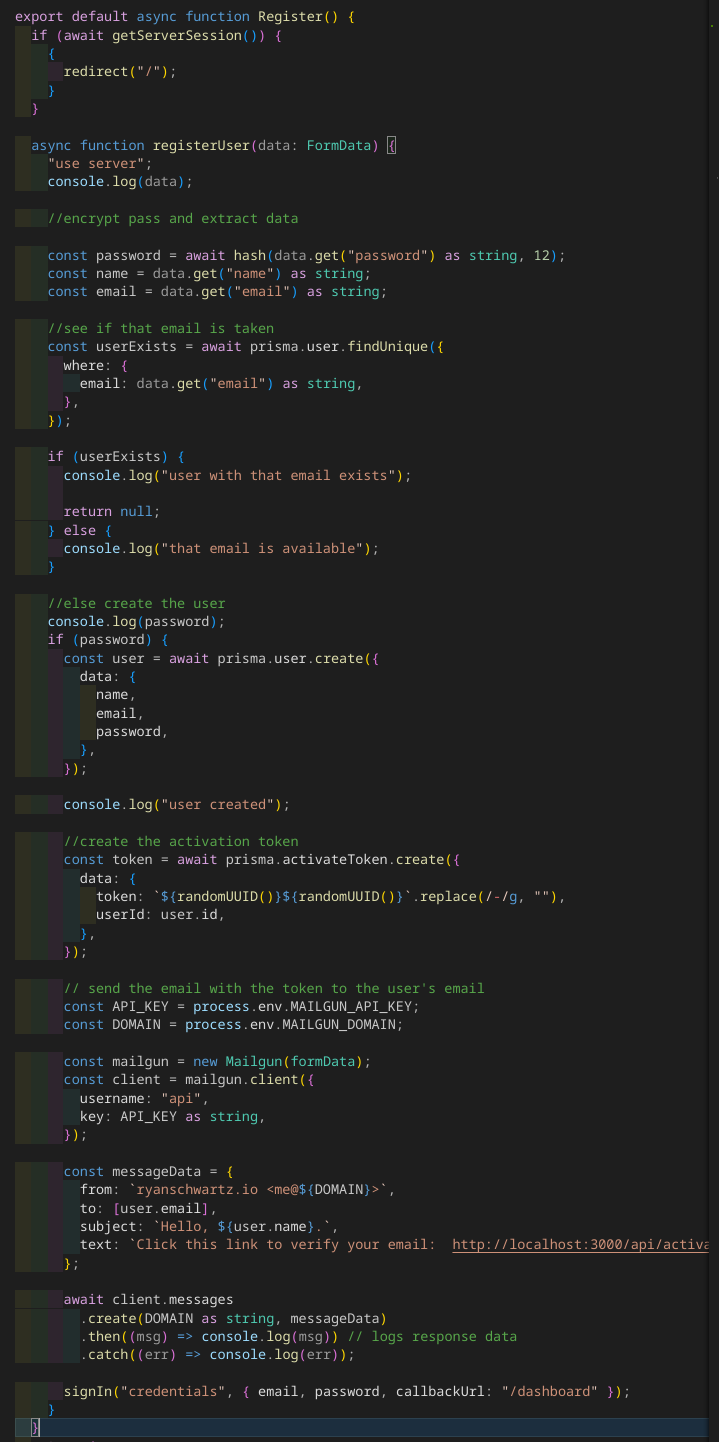
17 Replies
On your backend you need to return errors, and then on your frontend you could create a variable called "error" (or errors, if you wanna display multiple of them).
And you can just conditionally check if that variable has an assigned value and then display an error component
I’m more wondering how to do this with server actions. I’ve only done that with API routes that return NextResponses
Exactly how I said, just use an error variable and change that
Also remember that you should handle errors both on the backend and frontend
How?
just like return a JSON object?
Yes
And a status code that is different from 200
If you don't know what status code to use, check out https://http.cat
Funnily enough there's also https://http.dog
How do you intercept the response on the client? this is my current code:
so registerUser() is the server action, and inside the server action, I return a 200.
How do I "catch" the response?
so to speak
Such that I can render a “registration failed” error
In the page
I'm at work so I can't really help you now, I'll take a look once I'm done @machina0
:)
No worries at all
Hey @machina0
I wrote a small example on how you can get errors from your server actions
(HomePage)
(action.ts)
thank you! @gigantino
No problem :)
is this how you do it, or do would you personally still use an api route with a client fetch to do most stuff
i'm trying to understand what the best circumstances are to use server actions
I wouldn't always use them personally, and I've yet to experiment enough (they are still experimental, so I haven't had a chance as of right now)
If I were you I'd go with API routes
I usually just prefer to fetch data on the server to pre-render it, and then if I need to make other requests I just do them with API routes.
I honestly though love Svelte's approach of fetching data, and I think that the React and Next team should take inspiration from them
I'm curious about svelte. I'll have to mess around with it someday
You have to, it feels like black magic
I don't get how it does what it does