Message collector not working in thread
On discordjs 14.11.0. I cannot get a message collector to work in threads despite having no issue with other collectors such as for components. I've confirmed the thread is valid, as I can send messages to the thread using the same handle at the same point in the code. I have tried to log every event the collector triggers and I get a big load of nothing in the console, but it appears to instantiate correctly.
The bot has admin perms, all presence enabled, and is member of the thread.
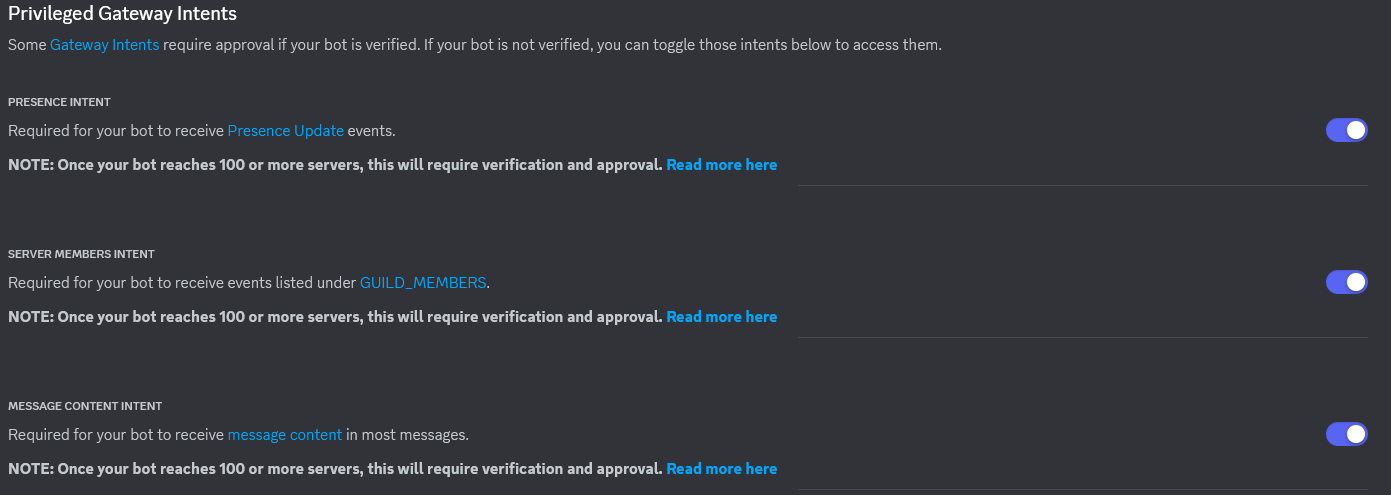
12 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Just updated to 14.12.1, no difference
do you have the messagecontent and GuildMessages intent?
also iirc createMessage.... returns a promise
createMessageCollector isn't async if that's what you mean
I will double check intents, I imagine the problem must lie there somewhere and it may have been misconfigured, as I don't believe there's anything wrong with the code
Is there a way to manage intents without re-inviting the bot to the server?
you dont have to reinvite it?
just add them
restart it and done
ah okay, preciate it 😄
I believe it already had all intents, is there anything else I need to configure besides what I showed in the attached image?
I gave the bot admin perms which I believe is the highest levels when I invited it
show your client constructor
ah
I see
that would be your issue
❤️ Is there a way to give it all intents without specifying them all?
no
that would also cause unecessary memory usage
understood, appreciate the help 👍