Noob question: How to pass a string to a child component?
I used prisma to get two strings from a database, now I want to give those two strings to a client component. This is because client components cant use prisma.
I'm new to react, how can I pass these strings to the client component? I'm thinking something like this: and then the main page.tsx would have like: How should I do this? This has been taking an embarrassing amount of time trying to figure out.
I'm new to react, how can I pass these strings to the client component? I'm thinking something like this: and then the main page.tsx would have like: How should I do this? This has been taking an embarrassing amount of time trying to figure out.
12 Replies
A client component can't be async either.
Ok so if it isn’t async, how do I pass in those values to a client component within a server component
did u try just passing first
I don't know how to do that. What did I do wrong in my example?
i updated it
Looks like you're using nextjs from your imports.
If you're doing server side rendering you'll need to use "getServerSideProps" to run your prisma call on the server, which then gets passed to the component.
https://nextjs.org/docs/pages/building-your-application/rendering/server-side-rendering
Alternatively (but doesn't look like your use case), if the inputs are known (think blog posts in a markdown directory, or database), you can use server side generation (SSG) in which case your HTML for each route would be built during the build process and served to the client when that route is requested.
https://nextjs.org/docs/pages/building-your-application/rendering/static-site-generation
He’s not using the pages router
So it sounds like the react server component needs to run the query and pass the data as a prop to the client component.
and this error in the server component:
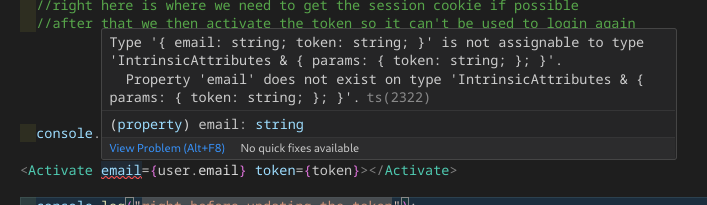
Prisma is doing the DB query on th server component, and the i want to give the output of that db query to the client component
but i don't know the correct syntax for passing it in as props and using them
this is the client component:
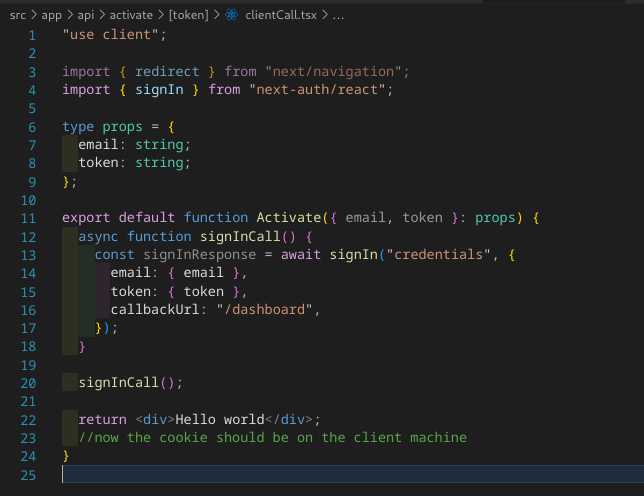
idk if thats how you pass in props to a client component from a server component