How to `update() ` the same interaction multiple times?
So I have this simple slash command that sends 3 buttons:
hello
, hi
, and wassup
When a user click one of the buttons, the message should change into the chosen message, which works as intended.
However, it only work once. The next click will result in "Interaction failed". I tried duplicating the awaitMessageComponent()
function call but it still doesn't work.
Any idea?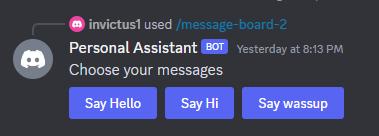
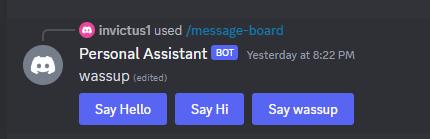
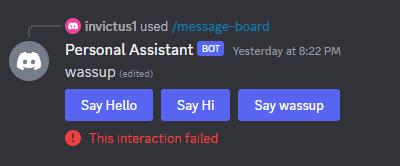
12 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!After calling .update() for the first time, you can use .editReply()
Should I make a new variable or can I just directly call
.editReply()
from userChoice
?You can call it on userChoice
Okay, let me try
Apparently, I ran into another problem. It looks like the function only run twice (First after the slash command is called, second after the user responds)? After that, my
console.log
no longer logs any things.
The next interaction only got intercepted by interactionCreate
listener, which is on a different file.
So the .editReply()
code doesn't even get called.You only await one button, so that makes sense
Use a collector if you want to collect multiple
Ohhh
What If I put the
awaitMessageComponent
on loop?No
Please dont
hehe it works though 😁
I mean, whatever floats your goat
Well, thanks anyway. I noticed that
awaitMessageComponent()
halts the function execution, saving the rest for the next interaction.
So, the loop is safe here, as it only runs when there is a new interaction.