Help with autocomplete and JSON.
This code searches within 3 JSONS (This will change at the end) it searches the keys "text" (name) and "reference" (Page in book.)
As it is I need to type the exact word for the search, I would like to use the autocomplete feature but it needs to search within the jsons. The search system I did only searches the exact words. They could help me with that. ?
const fs = require("fs");
const { SlashCommandBuilder, EmbedBuilder } = require("discord.js");
function filtrarDadosComPalavraChave(objetos, palavraChave, resultados) {
objetos.forEach((objeto) => {
if (objeto.children) {
filtrarDadosComPalavraChave(objeto.children, palavraChave, resultados);
} else {
// Verifique se as propriedades 'text' e 'reference' existem antes de acessá-las
if (
objeto.text &&
objeto.reference &&
(objeto.text.includes(palavraChave) ||
objeto.reference.includes(palavraChave))
) {
resultados.push(objeto);
}
}
});
}
function carregarDados() {
const dadosPadrao = require("../JSONS/rules.json");
const dadosMas = require("../JSONS/rules_ma.json");
const dadosMagic = require("../JSONS/rules_m.json");
const dadosCombinados = [
...dadosPadrao.rows,
...dadosMas.rows,
...dadosMagic.rows,
];
return dadosCombinados;
}
module.exports = {
data: new SlashCommandBuilder()
.setName("rules")
.setDescription("Verificar página relacionada com a regra.")
.addStringOption((option) =>
option
.setName("rule")
.setDescription("A palavra-chave para pesquisa.")
.setRequired(true)
),
async execute(interaction) {
const palavraChave = interaction.options.getString("rule");
const dados = carregarDados();
const resultados = [];
filtrarDadosComPalavraChave(dados, palavraChave, resultados);
if (resultados.length === 0) {
const embed = new EmbedBuilder()
const fs = require("fs");
const { SlashCommandBuilder, EmbedBuilder } = require("discord.js");
function filtrarDadosComPalavraChave(objetos, palavraChave, resultados) {
objetos.forEach((objeto) => {
if (objeto.children) {
filtrarDadosComPalavraChave(objeto.children, palavraChave, resultados);
} else {
// Verifique se as propriedades 'text' e 'reference' existem antes de acessá-las
if (
objeto.text &&
objeto.reference &&
(objeto.text.includes(palavraChave) ||
objeto.reference.includes(palavraChave))
) {
resultados.push(objeto);
}
}
});
}
function carregarDados() {
const dadosPadrao = require("../JSONS/rules.json");
const dadosMas = require("../JSONS/rules_ma.json");
const dadosMagic = require("../JSONS/rules_m.json");
const dadosCombinados = [
...dadosPadrao.rows,
...dadosMas.rows,
...dadosMagic.rows,
];
return dadosCombinados;
}
module.exports = {
data: new SlashCommandBuilder()
.setName("rules")
.setDescription("Verificar página relacionada com a regra.")
.addStringOption((option) =>
option
.setName("rule")
.setDescription("A palavra-chave para pesquisa.")
.setRequired(true)
),
async execute(interaction) {
const palavraChave = interaction.options.getString("rule");
const dados = carregarDados();
const resultados = [];
filtrarDadosComPalavraChave(dados, palavraChave, resultados);
if (resultados.length === 0) {
const embed = new EmbedBuilder()
11 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
they are nested
I tryied it more simple
and nothing!
let me try it
{
"type": "note_list",
"version": 4,
"rows": [
{
"id": "1f05daa9-67cc-4d3f-a0b9-96185664f7e8",
"type": "note_container",
"open": true,
"children": [
{
"id": "c07164b3-b73b-4428-945b-300078bd5255",
"type": "note",
"text": "When to Roll",
"reference": "BX343"
},
{
"id": "eeabb12c-4329-474c-8b2d-eadba1ca0ad7",
"type": "note",
"text": "Base Skill vs Effective Skill",
"reference": "BX344"
},
{
"id": "346b3eae-5212-4e37-b861-55209aef86ba",
"type": "note",
"text": "Modifiers",
"reference": "BX344"
},
{
"id": "33bbd880-7778-4860-bcb1-05f752ee984b",
"type": "note",
"text": "Sidebar: Default Rolls",
"reference": "BX344"
},
....
{
"type": "note_list",
"version": 4,
"rows": [
{
"id": "1f05daa9-67cc-4d3f-a0b9-96185664f7e8",
"type": "note_container",
"open": true,
"children": [
{
"id": "c07164b3-b73b-4428-945b-300078bd5255",
"type": "note",
"text": "When to Roll",
"reference": "BX343"
},
{
"id": "eeabb12c-4329-474c-8b2d-eadba1ca0ad7",
"type": "note",
"text": "Base Skill vs Effective Skill",
"reference": "BX344"
},
{
"id": "346b3eae-5212-4e37-b861-55209aef86ba",
"type": "note",
"text": "Modifiers",
"reference": "BX344"
},
{
"id": "33bbd880-7778-4860-bcb1-05f752ee984b",
"type": "note",
"text": "Sidebar: Default Rolls",
"reference": "BX344"
},
....
const { SlashCommandBuilder } = require("discord.js");
const fs = require("fs");
function extractTextFromNotes(notes, result = []) {
notes.forEach((note) => {
if (note.type === "note") {
result.push(note.text);
} else if (note.children) {
extractTextFromNotes(note.children, result);
}
});
}
module.exports = {
data: new SlashCommandBuilder()
.setName("autocomplete")
.setDescription("autocomplete")
.addStringOption((option) =>
option
.setName("query")
.setDescription("Input a query")
.setRequired(true)
.setAutocomplete(true)
),
async autocomplete(interaction) {
const value = interaction.options.getString("query").toLowerCase();
// Carrega o conteúdo do arquivo JSON
const jsonData = fs.readFileSync("../JSONS/rules.json", "utf8");
const jsonObject = JSON.parse(jsonData);
const choices = [];
extractTextFromNotes(jsonObject.rows, choices);
const filtered = choices
.filter((choice) => choice.toLowerCase().includes(value))
.slice(0, 25);
if (!interaction.replied) return;
await interaction.reply(
filtered.map((choice) => ({ name: choice, value: choice }))
);
},
async execute(interaction) {
const query = interaction.options.getString("query");
await interaction.reply({
content: `You Selected ${query}`,
ephemeral: true,
});
},
};
const { SlashCommandBuilder } = require("discord.js");
const fs = require("fs");
function extractTextFromNotes(notes, result = []) {
notes.forEach((note) => {
if (note.type === "note") {
result.push(note.text);
} else if (note.children) {
extractTextFromNotes(note.children, result);
}
});
}
module.exports = {
data: new SlashCommandBuilder()
.setName("autocomplete")
.setDescription("autocomplete")
.addStringOption((option) =>
option
.setName("query")
.setDescription("Input a query")
.setRequired(true)
.setAutocomplete(true)
),
async autocomplete(interaction) {
const value = interaction.options.getString("query").toLowerCase();
// Carrega o conteúdo do arquivo JSON
const jsonData = fs.readFileSync("../JSONS/rules.json", "utf8");
const jsonObject = JSON.parse(jsonData);
const choices = [];
extractTextFromNotes(jsonObject.rows, choices);
const filtered = choices
.filter((choice) => choice.toLowerCase().includes(value))
.slice(0, 25);
if (!interaction.replied) return;
await interaction.reply(
filtered.map((choice) => ({ name: choice, value: choice }))
);
},
async execute(interaction) {
const query = interaction.options.getString("query");
await interaction.reply({
content: `You Selected ${query}`,
ephemeral: true,
});
},
};
const { SlashCommandBuilder } = require("discord.js");
const fs = require("fs");
function extractTextFromNotes(notes, result = []) {
notes.forEach((note) => {
if (note.type === "note") {
result.push(note.text);
} else if (note.children) {
extractTextFromNotes(note.children, result);
}
});
}
// Carrega o conteúdo do arquivo JSON uma vez
const jsonData = fs.readFileSync("./JSONS/rules.json", "utf8");
const jsonObject = JSON.parse(jsonData);
// Extrai os valores de "text" e armazena em um array
const choices = [];
extractTextFromNotes(jsonObject.rows, choices);
module.exports = {
data: new SlashCommandBuilder()
.setName("autocomplete")
.setDescription("autocomplete")
.addStringOption((option) =>
option
.setName("query")
.setDescription("Input a query")
.setRequired(true)
.setAutocomplete(true)
),
async autocomplete(interaction) {
const value = interaction.options.getString("query").toLowerCase();
// Filtra as opções de autocompletar a partir do array choices
const filtered = choices
.filter((choice) => choice.toLowerCase().includes(value))
.slice(0, 25);
if (!interaction.replied) return;
await interaction.reply(
filtered.map((choice) => ({ name: choice, value: choice }))
);
},
async execute(interaction) {
const query = interaction.options.getString("query");
await interaction.reply({
content: `You Selected ${query}`,
ephemeral: true,
});
},
};
const { SlashCommandBuilder } = require("discord.js");
const fs = require("fs");
function extractTextFromNotes(notes, result = []) {
notes.forEach((note) => {
if (note.type === "note") {
result.push(note.text);
} else if (note.children) {
extractTextFromNotes(note.children, result);
}
});
}
// Carrega o conteúdo do arquivo JSON uma vez
const jsonData = fs.readFileSync("./JSONS/rules.json", "utf8");
const jsonObject = JSON.parse(jsonData);
// Extrai os valores de "text" e armazena em um array
const choices = [];
extractTextFromNotes(jsonObject.rows, choices);
module.exports = {
data: new SlashCommandBuilder()
.setName("autocomplete")
.setDescription("autocomplete")
.addStringOption((option) =>
option
.setName("query")
.setDescription("Input a query")
.setRequired(true)
.setAutocomplete(true)
),
async autocomplete(interaction) {
const value = interaction.options.getString("query").toLowerCase();
// Filtra as opções de autocompletar a partir do array choices
const filtered = choices
.filter((choice) => choice.toLowerCase().includes(value))
.slice(0, 25);
if (!interaction.replied) return;
await interaction.reply(
filtered.map((choice) => ({ name: choice, value: choice }))
);
},
async execute(interaction) {
const query = interaction.options.getString("query");
await interaction.reply({
content: `You Selected ${query}`,
ephemeral: true,
});
},
};
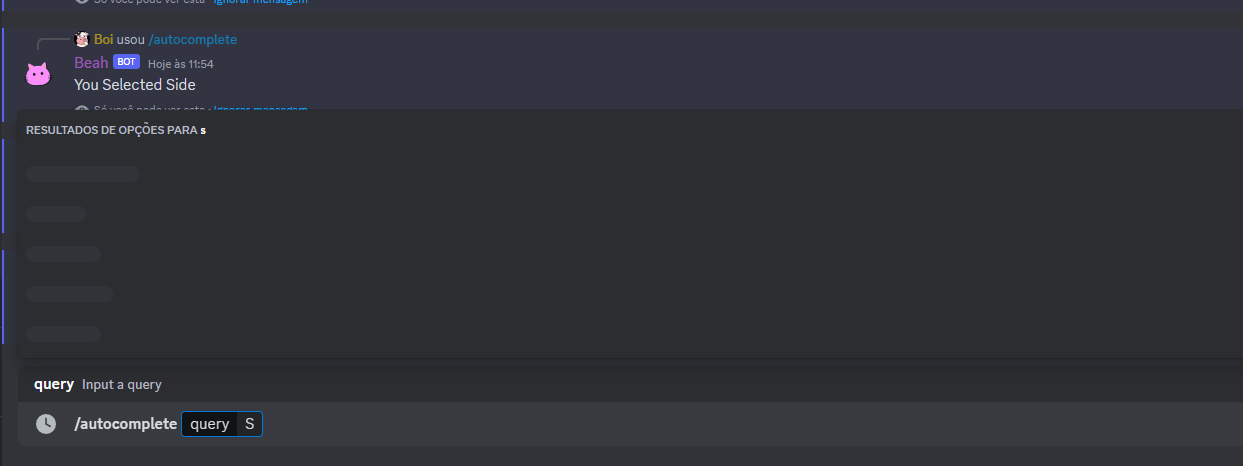
the path is ok too
const { SlashCommandBuilder } = require("discord.js");
const fs = require("fs");
function extractTextFromNotes(notes, result = []) {
notes.forEach((note) => {
if (note.type === "note") {
result.push(note.text);
} else if (note.children) {
extractTextFromNotes(note.children, result);
}
});
}
// Carrega o conteúdo do arquivo JSON uma vez
let jsonData;
try {
jsonData = fs.readFileSync("./JSONS/rules.json");
} catch (error) {
console.error("Erro ao ler o arquivo JSON:", error);
}
const jsonObject = JSON.parse(jsonData);
// Extrai os valores de "text" e armazena em um array
const choices = [];
extractTextFromNotes(jsonObject.rows, choices);
module.exports = {
data: new SlashCommandBuilder()
.setName("autocomplete")
.setDescription("autocomplete")
.addStringOption((option) =>
option
.setName("query")
.setDescription("Input a query")
.setRequired(true)
.setAutocomplete(true)
),
async autocomplete(interaction) {
const value = interaction.options.getString("query").toLowerCase();
console.log("Choices:", choices);
// Filtra as opções de autocompletar a partir do array choices
const filtered = choices
.filter((choice) => choice.toLowerCase().includes(value))
.slice(0, 25);
if (!interaction) return;
await interaction.respond(
filtered.map((choice) => ({ name: choice, value: choice }))
);
},
async execute(interaction) {
const query = interaction.options.getString("query");
await interaction.reply({
content: `You Selected ${query}`,
ephemeral: true,
});
},
};
const { SlashCommandBuilder } = require("discord.js");
const fs = require("fs");
function extractTextFromNotes(notes, result = []) {
notes.forEach((note) => {
if (note.type === "note") {
result.push(note.text);
} else if (note.children) {
extractTextFromNotes(note.children, result);
}
});
}
// Carrega o conteúdo do arquivo JSON uma vez
let jsonData;
try {
jsonData = fs.readFileSync("./JSONS/rules.json");
} catch (error) {
console.error("Erro ao ler o arquivo JSON:", error);
}
const jsonObject = JSON.parse(jsonData);
// Extrai os valores de "text" e armazena em um array
const choices = [];
extractTextFromNotes(jsonObject.rows, choices);
module.exports = {
data: new SlashCommandBuilder()
.setName("autocomplete")
.setDescription("autocomplete")
.addStringOption((option) =>
option
.setName("query")
.setDescription("Input a query")
.setRequired(true)
.setAutocomplete(true)
),
async autocomplete(interaction) {
const value = interaction.options.getString("query").toLowerCase();
console.log("Choices:", choices);
// Filtra as opções de autocompletar a partir do array choices
const filtered = choices
.filter((choice) => choice.toLowerCase().includes(value))
.slice(0, 25);
if (!interaction) return;
await interaction.respond(
filtered.map((choice) => ({ name: choice, value: choice }))
);
},
async execute(interaction) {
const query = interaction.options.getString("query");
await interaction.reply({
content: `You Selected ${query}`,
ephemeral: true,
});
},
};
🙂
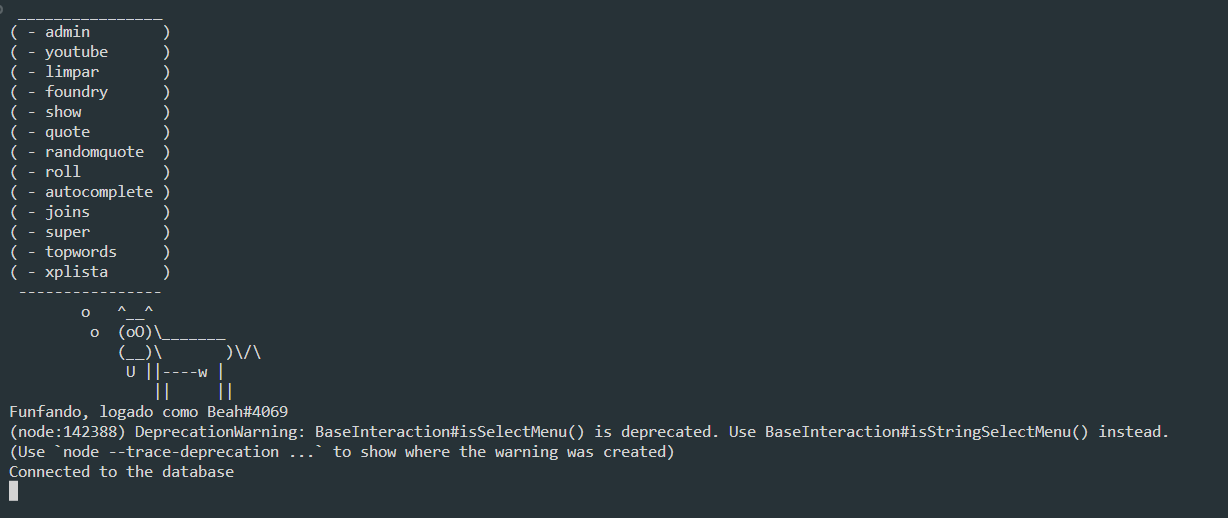
nothing!
yeah!
am i missing something?
yeah now i got first error
//index.js
client.on(Event.InteractionCreate, async (interaction) => {
if (interaction.isAutocomplete()) {
const command = interaction.client.commands.het(interaction.command.Name);
if (!command) {
return;
}
try {
await command.autocomplete(interaction);
} catch (err) {
return;
}
}
});
//index.js
client.on(Event.InteractionCreate, async (interaction) => {
if (interaction.isAutocomplete()) {
const command = interaction.client.commands.het(interaction.command.Name);
if (!command) {
return;
}
try {
await command.autocomplete(interaction);
} catch (err) {
return;
}
}
});
(node:176340) DeprecationWarning: BaseInteraction#isSelectMenu() is deprecated. Use BaseInteraction#isStringSelectMenu() instead.
(Use `node --trace-deprecation ...` to show where the warning was created)
Error executing autocomplete
DiscordAPIError[10062]: Unknown interaction
at handleErrors (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\@discordjs\rest\dist\index.js:687:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\@discordjs\rest\dist\index.js:786:23)
at async _REST.request (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\@discordjs\rest\dist\index.js:1218:22)
at async ChatInputCommandInteraction.reply (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:111:5)
at async Object.execute (C:\Users\cefas\OneDrive\Documentos\beah-bot\commands\rulestest.js:57:5)
at async Object.execute (C:\Users\cefas\OneDrive\Documentos\beah-bot\events\interactionCreate.js:33:7) {
requestBody: { files: [], json: { type: 4, data: [Object] } },
rawError: { message: 'Unknown interaction', code: 10062 },
code: 10062,
status: 404,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1145383132602839070/aW50ZXJhY3Rpb246MTE0NTM4MzEzMjYwMjgzOTA3MDpuZU1uTTJ3TklLOU9MakcyODJFNDg3ZGY3cUVDc2NoUXdobUZSekM2cVJjSWloODBtU3lETkFlb2FLYzRoUVp5SzI1MXN1dU9WVjhWZ1hEOUdwc1hPQnpOTXBFVldKR2lhVThlbUFsM3N5UlR3TDJ4Vnl1MHgyODlYblUzbk8wcg/callback'
}
(node:176340) DeprecationWarning: BaseInteraction#isSelectMenu() is deprecated. Use BaseInteraction#isStringSelectMenu() instead.
(Use `node --trace-deprecation ...` to show where the warning was created)
Error executing autocomplete
DiscordAPIError[10062]: Unknown interaction
at handleErrors (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\@discordjs\rest\dist\index.js:687:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\@discordjs\rest\dist\index.js:786:23)
at async _REST.request (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\@discordjs\rest\dist\index.js:1218:22)
at async ChatInputCommandInteraction.reply (C:\Users\cefas\OneDrive\Documentos\beah-bot\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:111:5)
at async Object.execute (C:\Users\cefas\OneDrive\Documentos\beah-bot\commands\rulestest.js:57:5)
at async Object.execute (C:\Users\cefas\OneDrive\Documentos\beah-bot\events\interactionCreate.js:33:7) {
requestBody: { files: [], json: { type: 4, data: [Object] } },
rawError: { message: 'Unknown interaction', code: 10062 },
code: 10062,
status: 404,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1145383132602839070/aW50ZXJhY3Rpb246MTE0NTM4MzEzMjYwMjgzOTA3MDpuZU1uTTJ3TklLOU9MakcyODJFNDg3ZGY3cUVDc2NoUXdobUZSekM2cVJjSWloODBtU3lETkFlb2FLYzRoUVp5SzI1MXN1dU9WVjhWZ1hEOUdwc1hPQnpOTXBFVldKR2lhVThlbUFsM3N5UlR3TDJ4Vnl1MHgyODlYblUzbk8wcg/callback'
}
hunnn it was a reply to chat
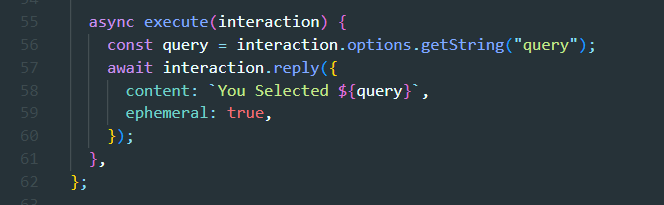
Dude. thank you very much but i really have no much idea what i'm doing here. it's my fisrt rodeo coding a bot to discord. I'll share the enritre project in github to someone take a look for me.
Thanks for all the help.
@qjuh Just a reply, I Did it, half hour reading documentantiion and it worked!