Cannot read properties of null.
const { SlashCommandBuilder, PermissionsBitField, EmbedBuilder } = require('discord.js');
const warningSchema = require('../../Schemas/warnSchema');
module.exports = {
data: new SlashCommandBuilder()
.setName('warn')
.setDescription('Warns a user.')
.addUserOption(option => option
.setName('user')
.setDescription('Username of who you want to warn.')
.setRequired(true)
)
.addStringOption( option => option
.setName('reason')
.setDescription('Reason for warning the User.')
.setRequired(false)
),
async execute(interaction) {
if(
!interaction.member.permissions.has(PermissionsBitField.Flags.KickMembers)
) return await interaction.reply(
{content: "Sorry! You don't have the sufficient permissions required to run this command!", ephemeral: true}
)
const {options, guildId, user} = interaction;
const target = options.getUser('user');
const reason = options.getString('reason') || "No reason provided!"
const userTAG = `${target.username}`
warningSchema.findOne({GuildID: guildId, UserID: target.id, Username: target.tag}, async(err, data) => {
if(err) throw err;
if(data) {
data = new warningSchema({
GuildID: guildId,
UserID: target.id,
Username: userTAG,
Content: [
{
Executor: target.tag,
ExecutorID: user.id,
Reasom: reason
}
],
});
} else {
const warnContent = {
Executor: target.tag,
ExecutorID: user.id,
Reasom: reason
};
data.Content.push(warnContent)
}
data.save()
});
const embed = new EmbedBuilder()
.setColor('#5077B4')
.setTitle('New Warning.')
.setDescription(`:white_check_mark: You have recieved a warning in ${interaction.guild.name} || ${reason}`)
const embed2 = new EmbedBuilder()
.setColor('#5077B4')
.setTitle('New Warning.')
.setDescription(`:white_check_mark: Succesfully warned ${target.tag} || ${reason}`)
target.send({ embeds: [embed] }).catch(err => {
return;
})
interaction.reply({ embeds: [embed2] })
}
}
const { SlashCommandBuilder, PermissionsBitField, EmbedBuilder } = require('discord.js');
const warningSchema = require('../../Schemas/warnSchema');
module.exports = {
data: new SlashCommandBuilder()
.setName('warn')
.setDescription('Warns a user.')
.addUserOption(option => option
.setName('user')
.setDescription('Username of who you want to warn.')
.setRequired(true)
)
.addStringOption( option => option
.setName('reason')
.setDescription('Reason for warning the User.')
.setRequired(false)
),
async execute(interaction) {
if(
!interaction.member.permissions.has(PermissionsBitField.Flags.KickMembers)
) return await interaction.reply(
{content: "Sorry! You don't have the sufficient permissions required to run this command!", ephemeral: true}
)
const {options, guildId, user} = interaction;
const target = options.getUser('user');
const reason = options.getString('reason') || "No reason provided!"
const userTAG = `${target.username}`
warningSchema.findOne({GuildID: guildId, UserID: target.id, Username: target.tag}, async(err, data) => {
if(err) throw err;
if(data) {
data = new warningSchema({
GuildID: guildId,
UserID: target.id,
Username: userTAG,
Content: [
{
Executor: target.tag,
ExecutorID: user.id,
Reasom: reason
}
],
});
} else {
const warnContent = {
Executor: target.tag,
ExecutorID: user.id,
Reasom: reason
};
data.Content.push(warnContent)
}
data.save()
});
const embed = new EmbedBuilder()
.setColor('#5077B4')
.setTitle('New Warning.')
.setDescription(`:white_check_mark: You have recieved a warning in ${interaction.guild.name} || ${reason}`)
const embed2 = new EmbedBuilder()
.setColor('#5077B4')
.setTitle('New Warning.')
.setDescription(`:white_check_mark: Succesfully warned ${target.tag} || ${reason}`)
target.send({ embeds: [embed] }).catch(err => {
return;
})
interaction.reply({ embeds: [embed2] })
}
}
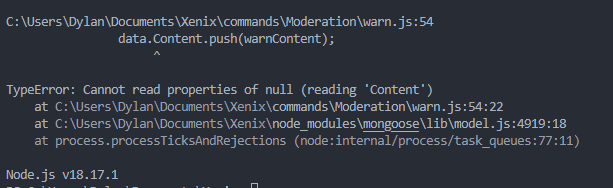
3 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPError is occurring at
(line 54)
data.Content.push(warnContent)
data.Content.push(warnContent)
Unknown User•10mo ago
Message Not Public
Sign In & Join Server To View