Let vs var vs const
Can any one help me understand how these three different ways to create a variable really works within a function?
From what i already learn var is functions scoped let and const is block scope. Whats confusing to me is does this mean that if I create a variable using var I can use that variable anywhere inside the function and let/const can only be used within a specific block for example a if/switch statement?
Also how do you even know when its the right time to use a specific type of variable?
Thanks in advance
19 Replies
In short, any variable created with
let
or const
is only accessible within a scope, which is essentially any code within curly brackets. This includes if statements, for loops, functions, etc.
This means that you can have two variables with the same name, as long as they are not within the same scope. Not that you should intentionally do this, on the contrary, but it is technically possible.
As for when you choose which one to use, it's simple: use let
when you expect your variable to be reassigned; otherwise, use const
. Don't use var
. If you ever have the feeling that using var
may solve a particular problem that you are having, it's a clear sign that you need to reconsider the entire approach you are taking.Okay that was going to be my next question. From what i'm seeing and reading everything kind of advise against using var and I still cannot figure out why?
thats just my curiosity kicking in trying to understand why
One note regarding
let
and const
. If you declare an array or an object, you still can modify the value of the variable. For example, you can push items into an array, or add new properties to an object. That is not the same as reassigning the variable itself. Therefore you can declare arrays with const
because this only means you won't be reassigning the variable into something else, not that the array won't be modified somehow.There are, like, five different questions here :p
But I'll try to answer them as best as I can.
var
is either function scoped or globally scoped. So any var
declared in a function is scoped to that function and won't "leak" out. But any other place you declare a var
it will "leak" into the global scope: for loops, if statements, switch statements, etc. function
or nothing. It is for this reason that var
is frowned upon.
let
and const
are pretty much the same, with the only difference being that you can re-assign a let
but can't re-assign const
:
Both let
and const
are block scoped, so any place you have curly braces {}
the variables won't "leak" out. So a for loop, a while loop, an if statement, etc. that's why let
and const
are preferred.Here's a very basic example of the differences:
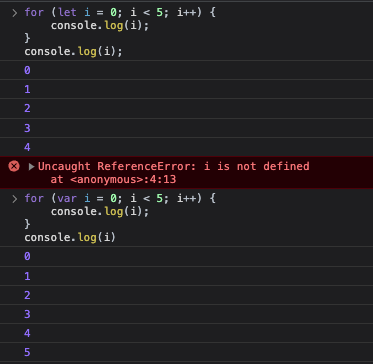
I just learn this like 20 mins ago lol
My personal opinion is that you should default to
const
, to prevent yourself from accidentally overwriting a variable. Change it to let
if you find yourself wanting to re-declare it. For example, if you're looping through something and it's updating a variableOne of the problems
const
and let
were supposed to solve was the risk of name collisions. You don't want to accidentally overwrite a variable from a library that you imported from npm or something.And yes, Joao is right:
const
only prevents you from re-assigning/re-declaring a variable. But "complex" (IE non primitives) can be modified. Primitives are:
* Null
* Undefined
* booleans
* numbers
* bigints
* strings
* symbols
Oh, yeah, that's a good point!
The following is valid JS:
But this will throw an error:
I've been noticing this tatic a lot. Even on som tutorials I watch and read const is the main but then gets changed to let if the variable is getting changed
Defaulting to
let
or const
is really a personal preference. I've seen valid arguments either way. Regardless of which you use, if you try to let a = something
or const a = something
more than once you'll get an error.That's another advantage. If you default to use
const
everywhere and I'm reading your code and I suddenly see a variable declared with let
, I immediately I'm aware that somewhere this will change values. So this syntax also signifies intent.This helps me out a lot. Thanks for the input guys I appreciate this
@dellannie Btw, in this code example from just above https://discord.com/channels/436251713830125568/1150169594036764682/1150174315002740817
Note that you can change a variable from one type to another e.g., from a number to an object, or string, or whatever. Try not to do that. It's fine to update the value from 21 to 42, but not from 42 to "John". It's possible but... meh, not so good in general.
okay
The only exception tothat would be if you need to somehow "destroy" the variable, so that would be assigning it to
null
. That's actually okay and quite useful actually, but yeah try to stick to one type per variable.okay. Havent got that far yet but all this stuff now I have a better understadning of how these variables work
Great, the better your understanding of the fundamentals the easier it'll be to learn everything else 🙂
Thats all I've been working on for the last few weeks trying to just understand how the fundamentals work.