MongoDB cant get an item to be added to a shop schema
hi, so basically im very lost, im trying to make it so a bot can have a custom shop with itemName, itemPrice and itemDescription, my shopItemModel.js: the add-shop-item.js code:
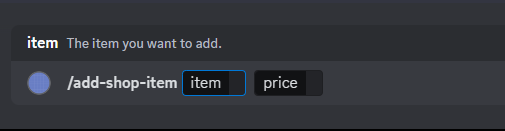
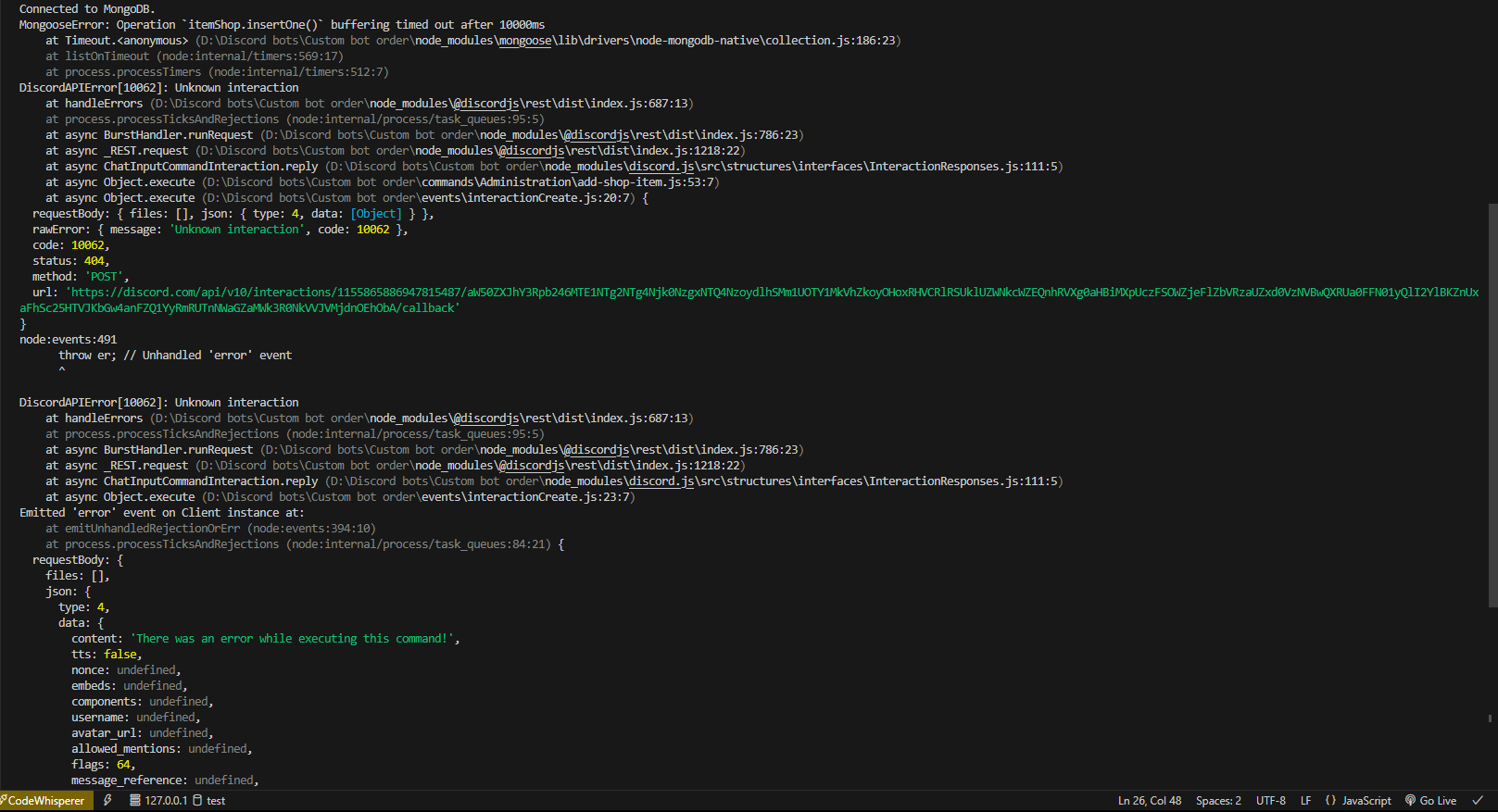
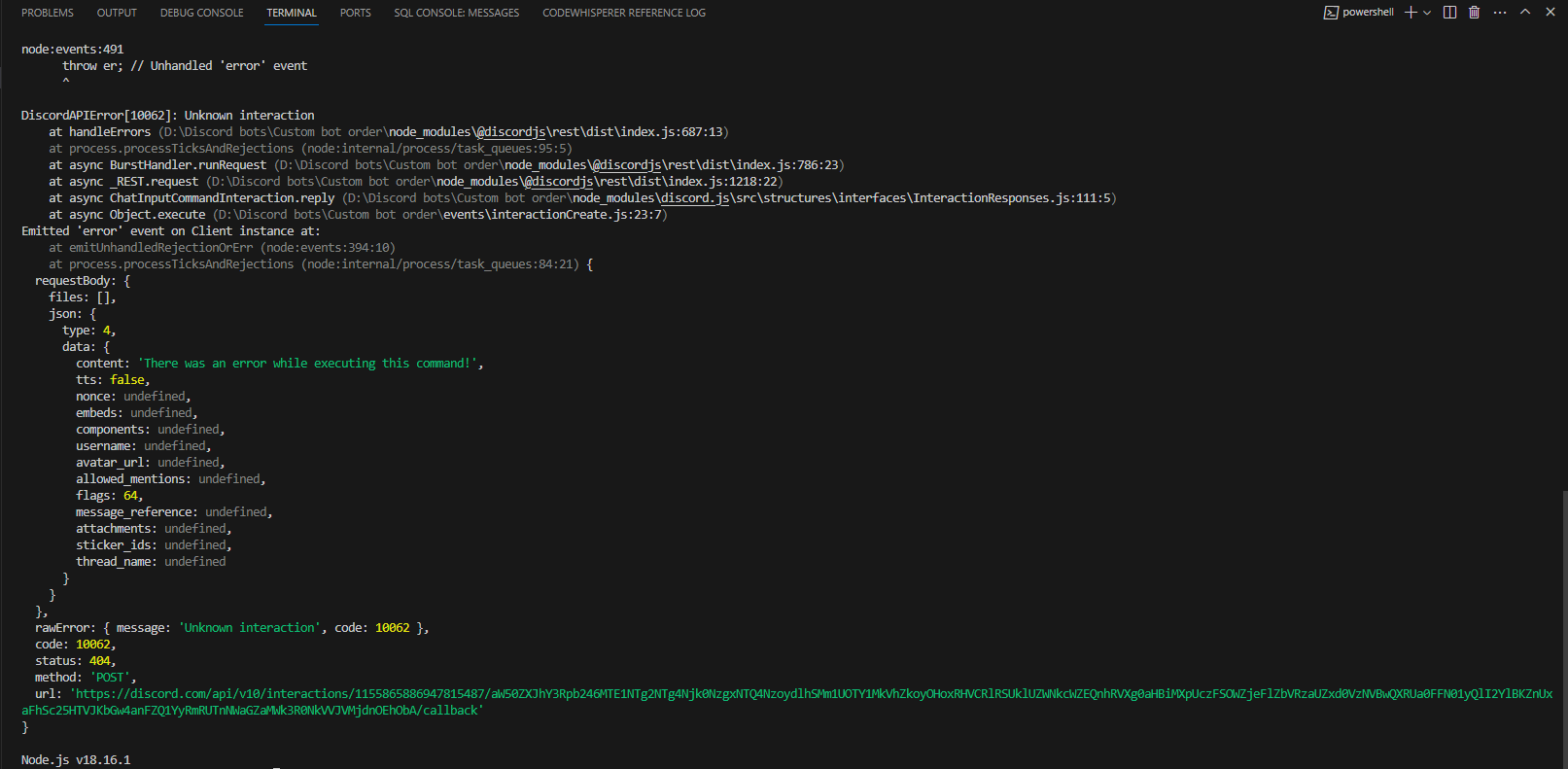
3 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffit is
its not djs related anyway