How can i setup a ticket system with a parent category?
deploy-ticket.js code:
178 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!that setType is incorrect
how should it be?
its addChannelTypes
types being specified with that enum
that entire code is v13
you didnt happen to ask chatgpt did you?
i did T_T
yeah dont
its that obvious huh?
the comments + the fact that code is outdated
thing is that discord docs dont provide with examples on how to use it
well observed
use what?
buttons?
somehow i got 20 commands done with it and it works
yes
then you are using an outdated version
i wanna make a command called deploy-ticket wich will let me specify the this variables in this ticket model:
somehow it works perfectly π
then tag this post with the version you use
which is not v14
also if you're asking how to get data from a db, #other-js-ts for that
i use V14 tho
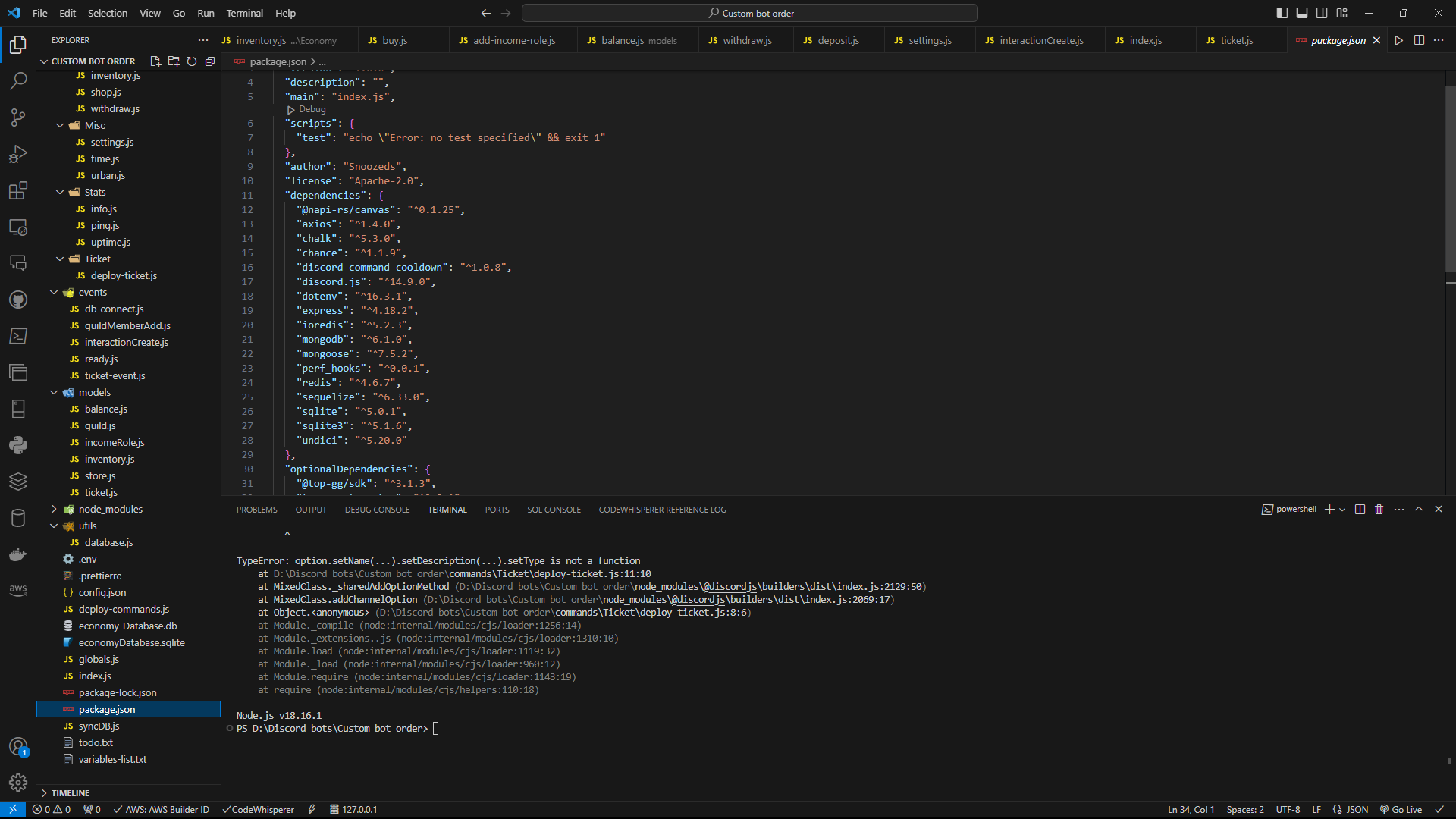
and waht does npm ls discord.js return
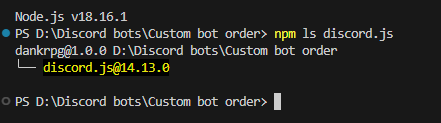
the code above shouldnt work
because its invalid
anyhow whats your question
how do i set it up so it asks for the channel category
ik how to make it ask for channel_id but not chanel category
use collectors and channel select menus
or use a slashcommand and make it so you only can input categorychannels
is there any template that has an example of how its used?
yeah im using slash commands
no
the guide explains how everything works
then you would need to adapt it to your use case
well choose what you want
because the code you showed uses both a slash command and selectmenus
yeah but i dont see any examples of how to use the GuildCategory
this is all it gives me
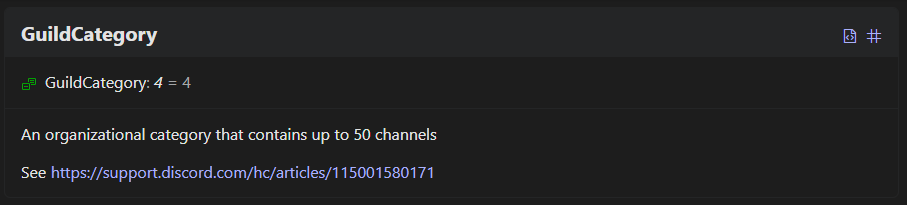
its an enum
you put it in the addChannelTypes
and then only Categorychannels will be populated into the results
like this
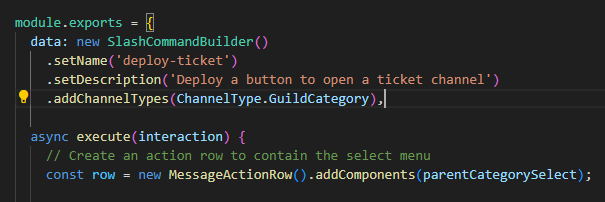
yes
thats one part fixed
the rest of your code still is v13
yayy
still not v14
Huh, so addChannelTypes is not a function
oh yeah you need to add a channeloption ofc
like this?
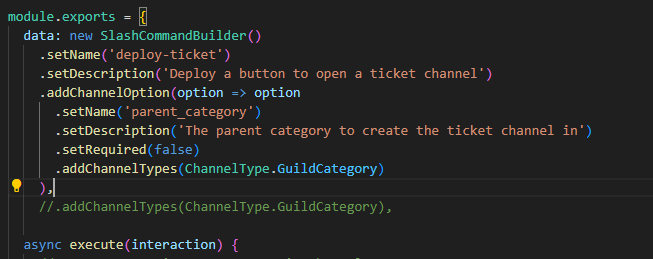
yep
somehow managed to get it working π
time to execute the command and see the bot die
called it xd

i got this now
and i get this now:
what do i do @wolvinnyπ
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
ohh
i need to import it from discord.js right?
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
like this right?
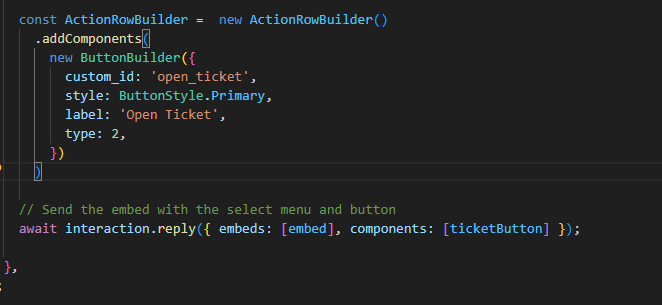
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
yeah ik
i usually do it but ijust forgot
YESSSS
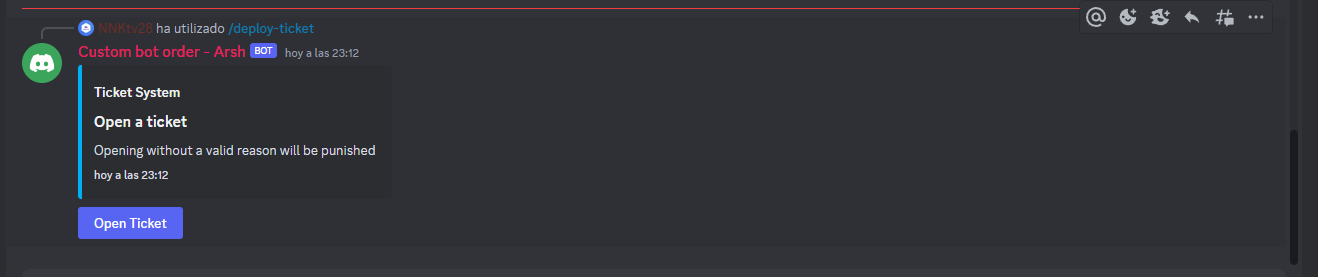
yall are the best
now i need to make it so that when i press the button, it opens a channel under a certain channel category
is there any way to specify that?
this is what i use to listen to when someone presses the button
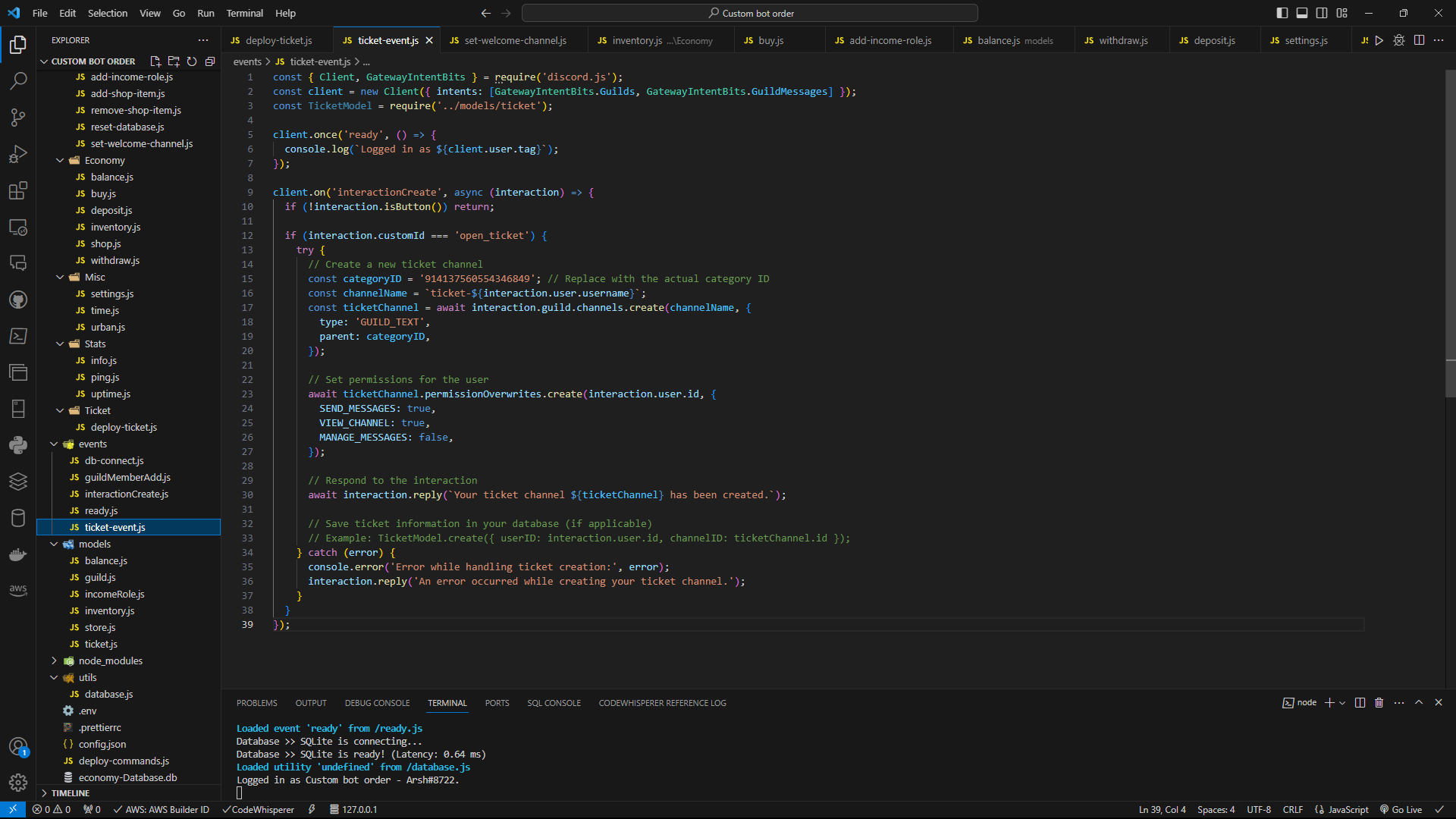
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
thats also v13
so just
dont ask chatgpt
and follow the guide
hehe i will π
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
π
thing is ive spend 3 days on this thing and i barely did any progress cause i try to watch tutorials and read docs but most times i dont understand anything at all
so easyest option is chatgpt
chatgpt is what made you end up here
so maybe consider following the guide
and if you dont understand the guide i recommend learning the basics of js first
i do know the basics of js but todays not my most brightest day π
i cant find the parent property, is it from discord.js V13?
i guess its like this
parent: TicketParentChannel,
yes
what happens if parent = Null?
will the channel create somewhere random?
it will create a channel without a parent
oh alright thats fine
probablt at the top of the guild
did i link it right with the button? or do i need to specify the buttons script and then call the button custom_id?
have you tried your code
yeah, gives interaction error and no console feedback
does your event fire at all
ah
why do you construct a new client there?
you have to construct 1 client
and log in with that one
use that to handle events and commands
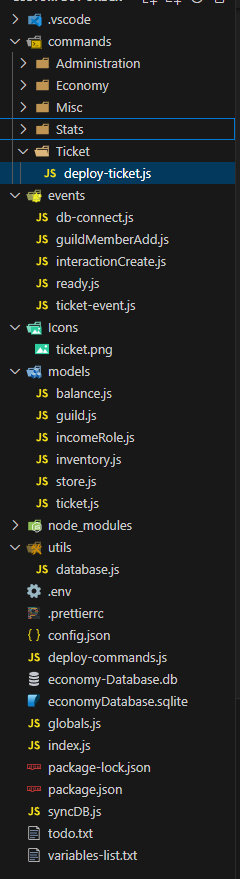
just you having those folders wont magically handle your commands / events
yeah ik
i load the events in the index.js file
and the commands too
then why did you construct a new client?
you have the events right there already
u mean i need to do this instead of
client.on('interactionCreate', async (interaction) => {
?Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
also
again
there is no ticketEvent
forgot the ""
and you never even defined that variable
wdym? where am i making a new client?
nvm just saw it
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
didnt even see that
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
half of it ig, most is copy paste from the other events and everything they have similar π
im reading the https://discordjs.guide/creating-your-bot/event-handling.html#individual-event-files and i see that they also added some stuff t o interactionCreate.js
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
yep
time to figure out what i need to add π
should i move to interactionCreate,js?
or make it a function and call it from interactionCreate.js?
if you check your interaction types correclty
you're better off making it a function
and make a button handler
how does that work t he button handler? is it another .js file in events folder?
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
so i made it a function
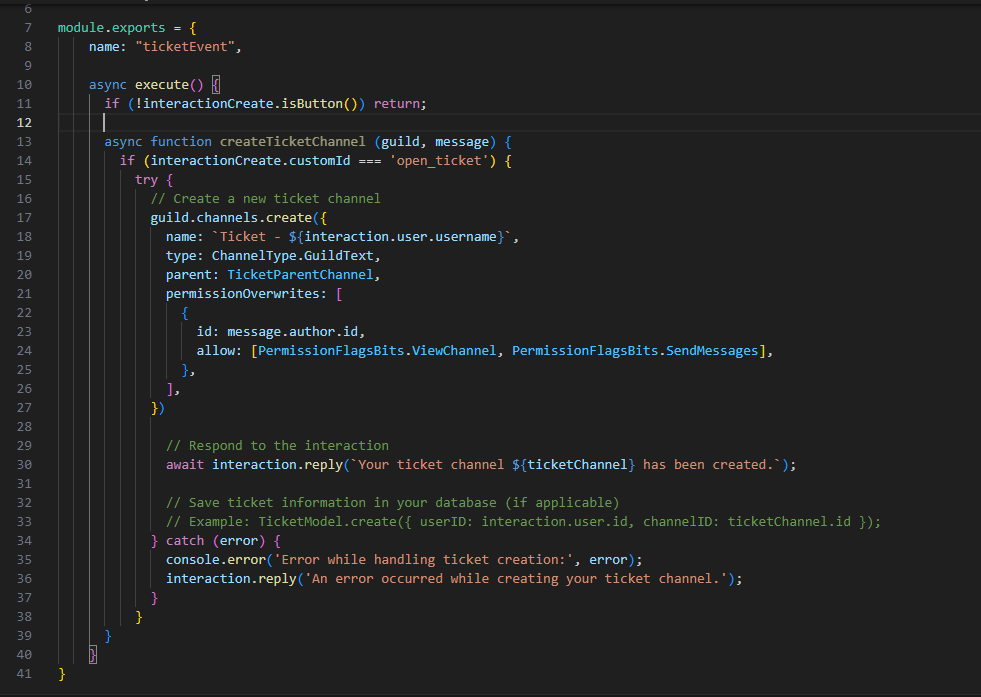
ig now i need to pass it to interactionCreate and call it from there?
you did not define interactionCreate
Nor do you properly export the function
this is basic js at this point
im on 2 monsters and 5 hours of sleep π
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
im studying web development so ik what no sleep is like π
is there any discord bot in v14 that i can just copy it from? cause im seing myself stuck with this for at least till next week π
Unknown Userβ’2y ago
Message Not Public
Sign In & Join Server To View
T_T
how is it that i can do it in python but not in JS π
can i have multiple module.export per .js file or just 1?
just one but you can export multiple things
not djs related however
oh alr
suggestions tempt me
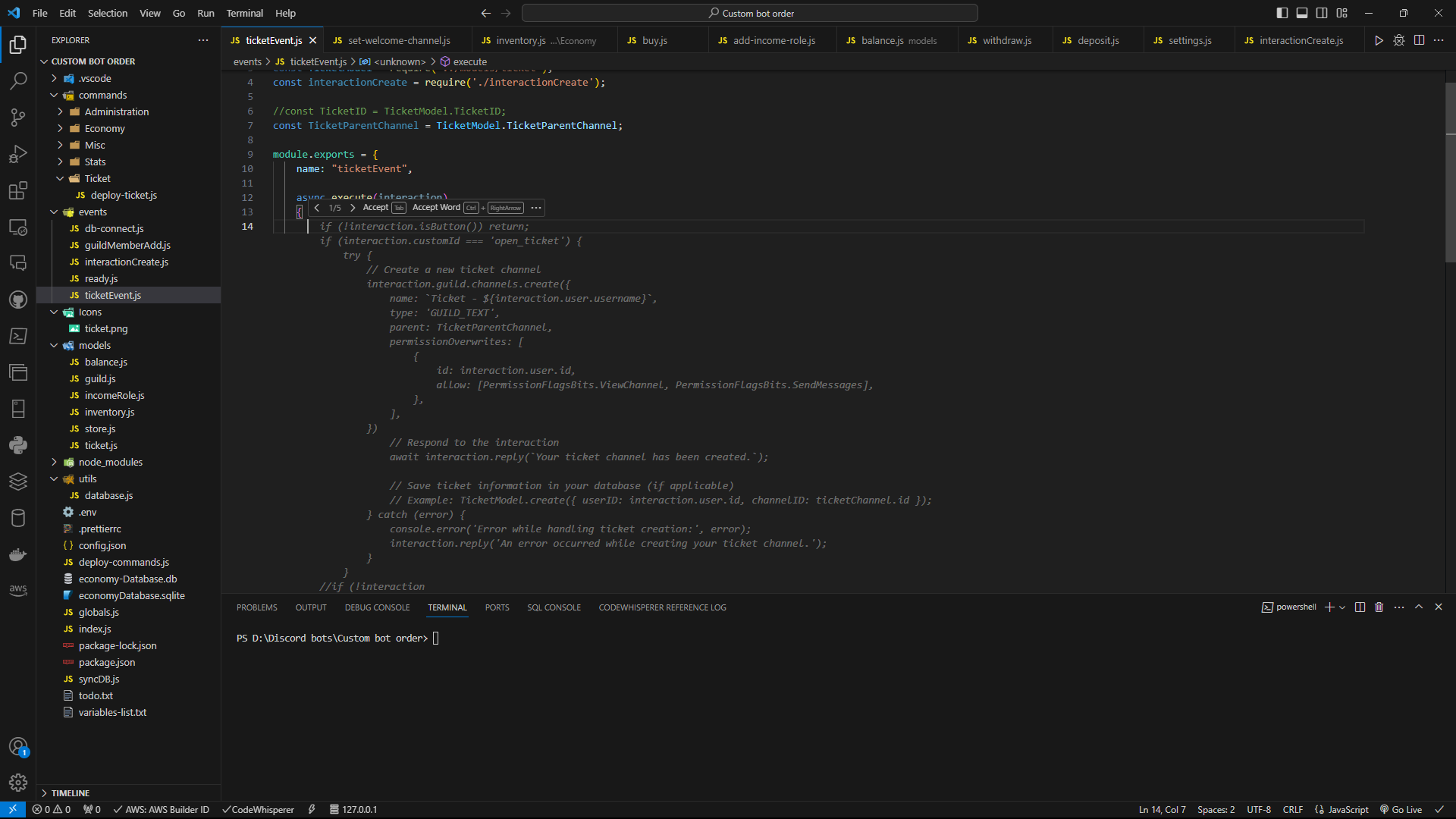
is this the right way? its in interactionCreate.js
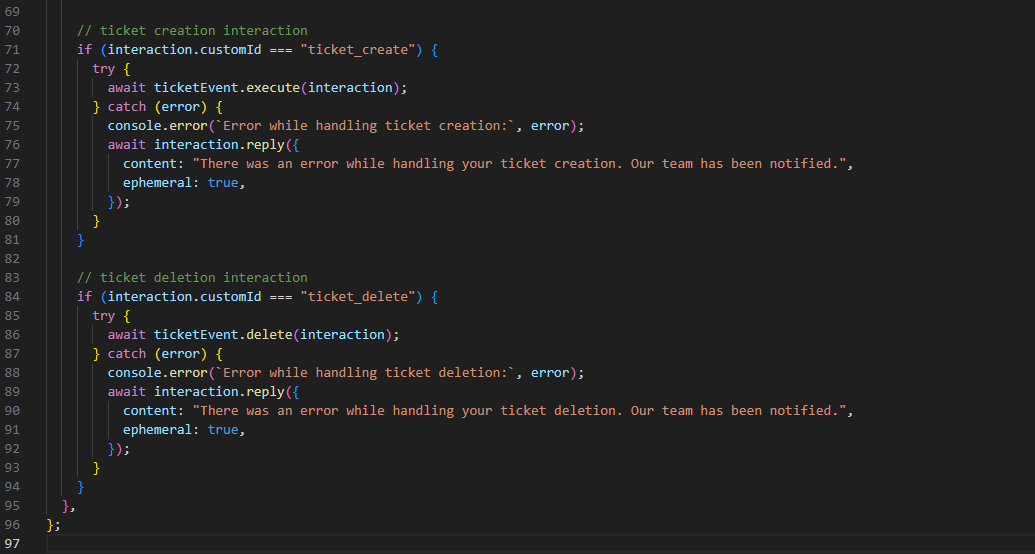
assuming you export those functions
also in the deploy-ticket command i dont see any option to specify a parent category even tho i defined it here:

then you didn't redeploy
i needed to redeploy? i thought that was only to register the slash command, not to modify it
indeed
i needed to redeploy
well yeah editing your command wont magically edit it in discord
i thought it was enough with reloading the bot
whats the difference between this at the start of the module and
module.exports = ticketEvent;
at the end of the module?what is ticketEvent defined as
also this isnt djs related anymore
So #useful-servers #other-js-ts #resources
and?
idk what im even doing at this point im just trying stuff i see tbh
you shouldn't do that
You should have a strong js knowledge before beginning djs
as per #rules 3
i never tryed doing such a hard bot, mostly i did was the typicall mod bot, but never got this far into it
im about to toss the entire ticket idea π
this is what i want to archive
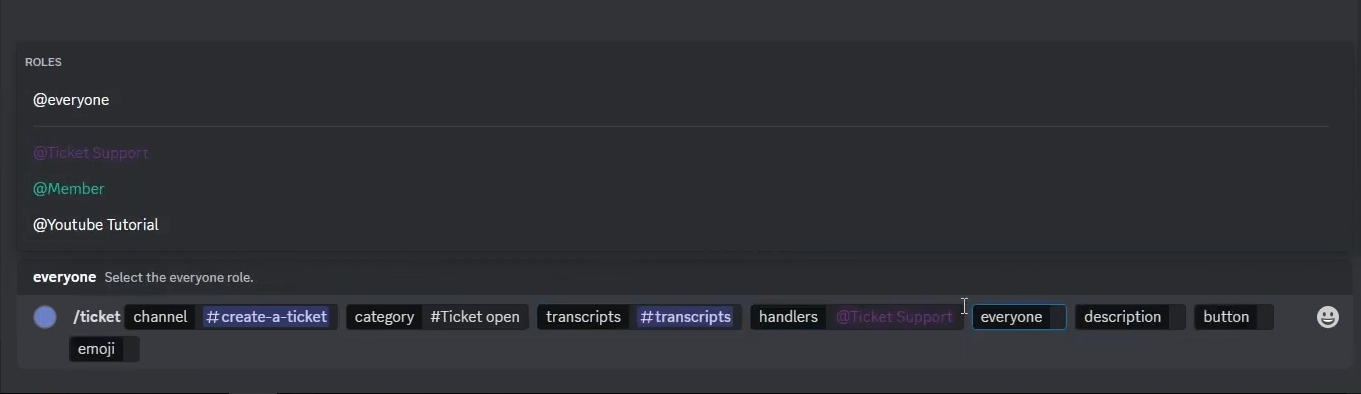
i did some progress π
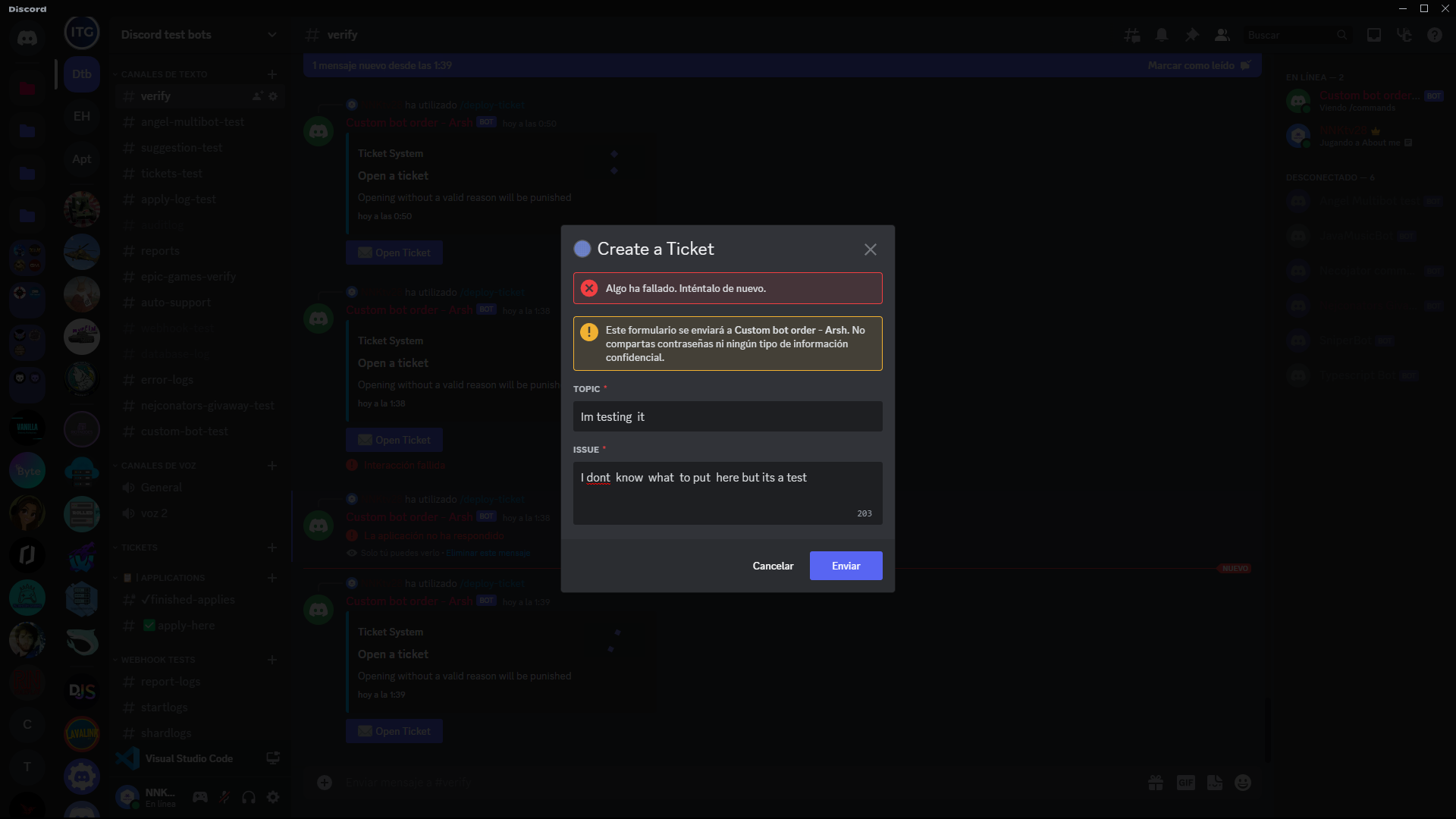
im not completely useless
i got it π
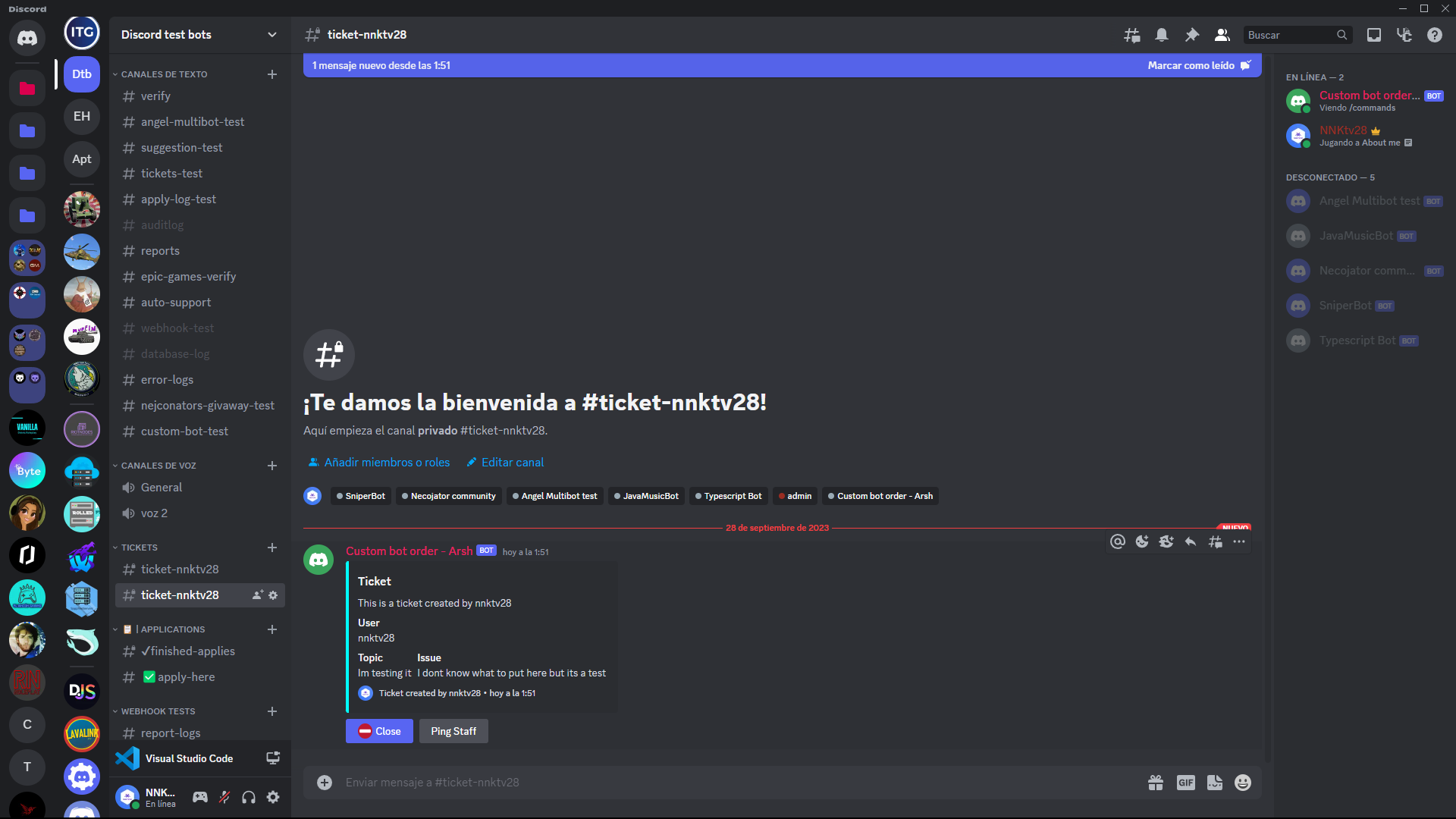
what u gona say now huh
stop, people are here to help you, if you were told your js knowledge was lacking its because it was, and it makes it super difficult for us to try and help you, too. Its nice that you are making progress.
I was just kidding mate π
Ik and i always apreciate everyone who helps me
I ended up bringing the interactions to the index.js file
so the ticket gets created and crashes the bot when i try to ping staff
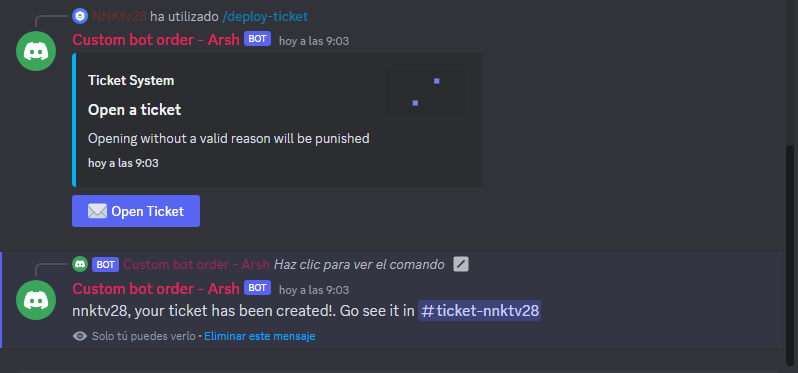
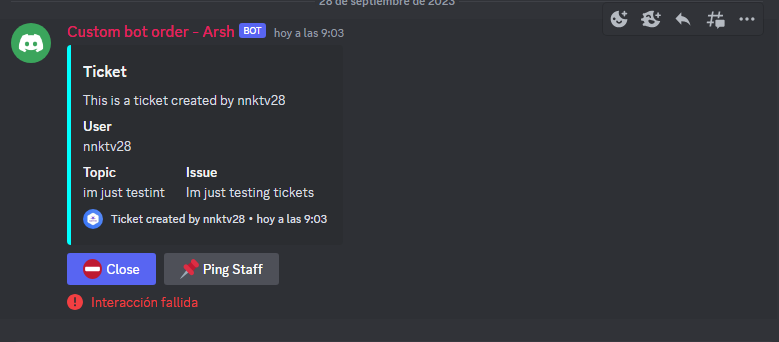
and i get this error:
you are trying to call map on a string
const StaffID = "1106128477528264774";
so i just remove the .map?
Nope dont work
how should it be?
you have one id which is a string and it seems like you want to format it as a mention, do that
its a string, you can preformat it
no need to go through a function
oh alr thxx
yess it worked
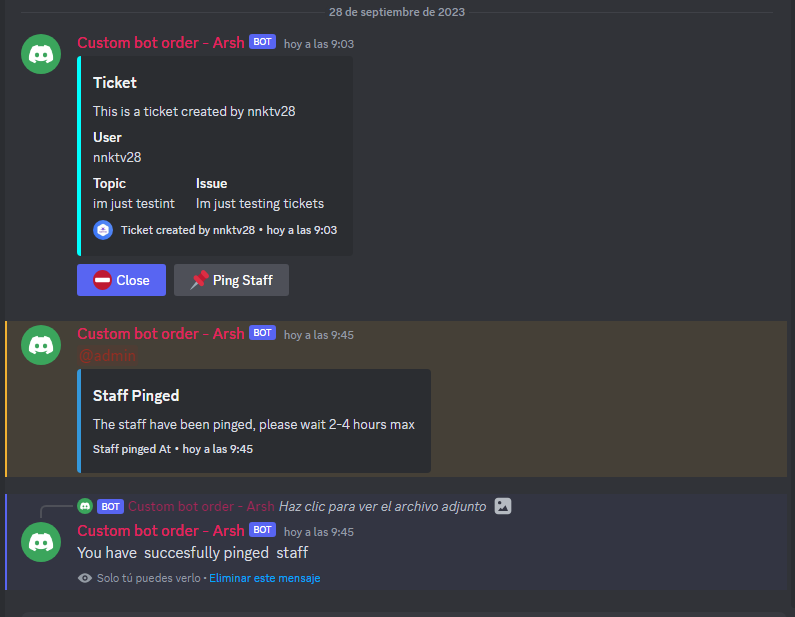
great now the delete button ticket broke and idk why:
And which code runs
Since you have 2x code that handles the same button
wait leme see cuz i actually dont know if its the on in interactionCreate is the old one
indeed it was the old one
so yea this is the one that runs it
There is no code to actually delete the channel
Nor do you reply to the interaction
so this does nothing?
interaction.channel.delete();
or should i specify the channel inside () like ('${channel}')
It deletes the channel the interaction happened in
yeah that the objective
delete the channel where the delete button was pressed
but since nothing happens at all i doubt that code is even being reached
yep its not reaching π
i'm pretty sure you just created a new client again
Because your other buttons work
So i dont see why you create a new event
dont think so, since im in the index.js i use Client.on
so i dont need to make a new client
You already have an interactionCreate event where you handle the other buttons
You need 1, not multiple listeners
so i can move the code in side this client.On
inside here?
yep there
and have only one client.on(Events.InteractionCreate, async interaction => {
oh alr thx
yeah makes sense now that i think about it
now not even staff ping button works π€£
first of all, <User>.reply doesnt exist
If you aren't getting any errors, try to place
console.log
checkpoints throughout your code to find out where execution stops.
- Once you do, log relevant values and if-conditions
- More sophisticated debugging methods are breakpoints and runtime inspections: learn moreim an idiot
the issue was that i needed to define (${'channel}')
interaction.channel.delete(
${channel}
);
like that
now it deletes the channel
π
although it does not like my ping button:
im not even gona ask about that cause il get 20 people shouting at me that its not discord.js related πCommon causes of
DiscordAPIError[10062]: Unknown interaction
:
- Initial response took more than 3 seconds β defer the response *.
- Wrong interaction object inside a collector.
- Two processes handling the same command (the first consumes the interaction, so it won't be valid for the other instance)
* Note: you cannot defer modal or autocomplete value responsesim pretty sure its because of my sqlite database that im probably calling the AdminRole variable wrong
even though i do get the bots response cause theres not any role stored yet
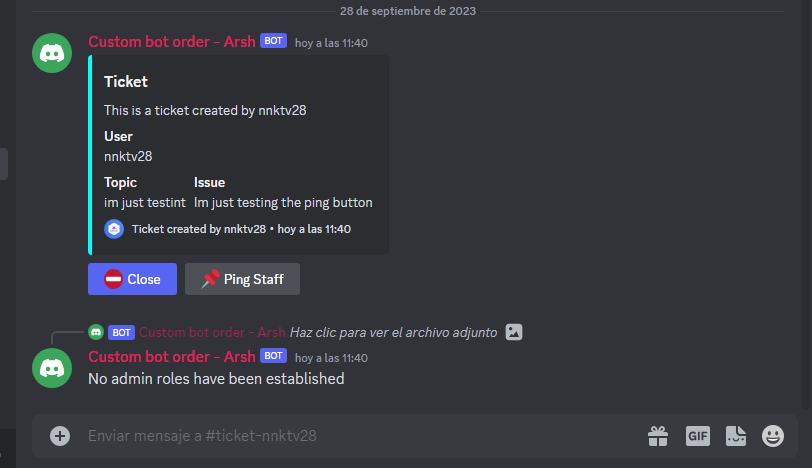
You shouldn't have to pass in the channel to delete
for some reason i did
seems like the delete button works after i open the ticket and the bot doesnt crash, once either i restart it or it crashes it doesnt work anymore
this describes the behavior one might expect when using either a collector or otherwise a nested
interactionCreate
listener
given that you're not using a collector, I'm assuming the latter, which means that the change you made to consolidate into 1 listener was completely reverted
wolvinny is also correct, you don't need to pass a param for <GuildChannel>.delete()
it accepts a string for the reason
for deletion, but it's not required or being used to determine what channel to delete
likely something else changed alongside your addition of this param so that it was simply perceived as this being the fix
it's hard to say exactly what given that you've thus far made several seemingly random changes without understanding themnow THATS what i call a great explanation, and a good deduction wich is half right cuz the specification of the channel was actually a desperate try
thats the code i did change
its how it is right now
also i did not revert
rule 1 of coding, something works then dont touch it, ima apply that rule to this
nobody said that you have to remove it
just that you shouldn't consider that to be what fixed the issue
that being said, are you not still using slash commands?
at the beginning of the thread you were, but if this is the one and only
interactionCreate
listener you have, where are you handling command interactions?i use slash commands since the start and deploy them usign node deploy-commands.js
yeah all the commands what they need is to just access a sqlite database
and thats all
for example this deposit.js:
this is what the rest of commands looks like
yes, and therefore somewhere you are receiving command interactions
I don't see any code for it here https://discord.com/channels/222078108977594368/1156684794059567275/1157034503181709402
therefore you have not consolidated all
interactionCreate
listeners into 1 as wolvinny recommendedthis is the interactionCreate.js code:
on the first line of this
execute
function you return if the interaction is not a command interaction
then later after handling commands, you attempt to handle what I assume is a button interaction
an interaction will never be both a command interaction and a button interaction
you'd want to rework the logic of this function so that it can accommodate both command and component interactions, then work from there
that way the components being handled in this separate listener here https://discord.com/channels/222078108977594368/1156684794059567275/1157034503181709402 can be consolidated into one interactionCreate
listenerohhh alright
so interactionCreate can only handle 1 interaction type?
no,
interactionCreate
handles all interactions
by returning on the first line if it's not a command interaction, you are only handling 1 typeohh okay
Heyyy i got progress
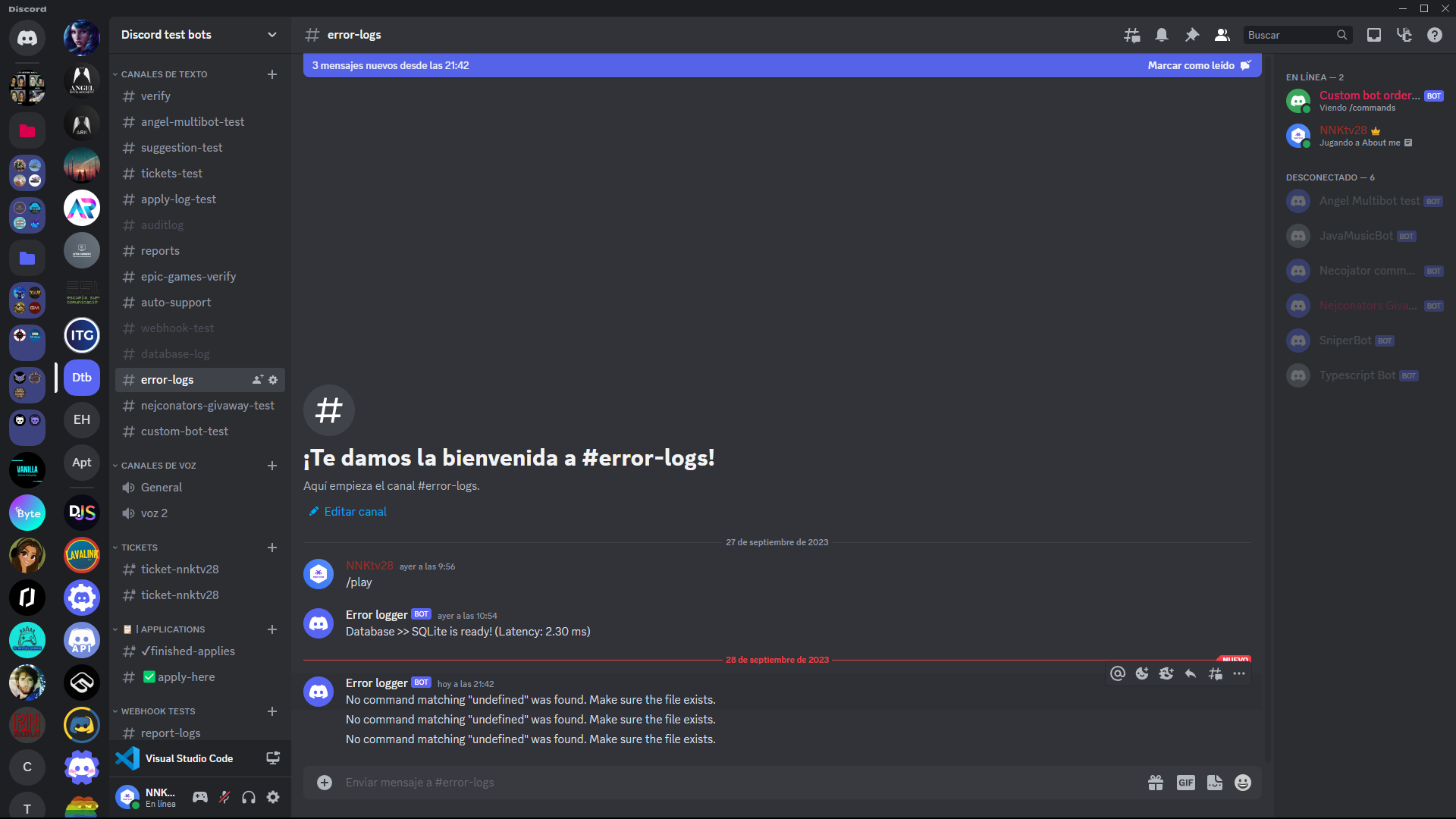
i commented the 1 line you told me and now the ping button works π
I didn't tell you to comment any lines, but ok
ik but i tryed it and it worked perfectly
cause tecnically the button interaction is not a command interaction so it made sense in my head to remove it
on the assumption the line you're referring to was your typeguard, I can only assume this is further straying from the advice you've been given
alright then my brain is not braining, ima head to bed and hope i can get it right tomorrow
cause i understood that the line was the one stopping the button interactions cause they werent command interactions
and due to that the button interaction wasnt going through
rest is important, so I leave this explanation for when you wake up:
this isn't just a "this is the problem code so you should remove it" situation
you do want to prevent component interactions from being handled as command interactions
however the same applies for the other way around: you don't want command interactions from being handled as component interactions
therefore typeguarding is still important, it just needs to be handled differently than simply returning on the first line
ima continue with other commands and leave the ticket thing for when i finish the commands cause i dont want to spend more time than i need to on a single command
i got 2 weeks to finish this bot or im screwed
pls dont delete this channel cause i will need it in the future withon 2 weeks
why take on a deadline if you cant handle it?
cause i can take it, and thats the only command thats giving me issues
the rest of the commands work fine
+ the ticket thing is optional and i wanted to add it as an extra for the client