21 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!If I had to venture a guess on the issue, it’s likely the database query. But a little more info would help. How long is it taking to complete? Is there any error? “I’ve got a problem” isn’t giving us much to go off of.
no errors and warnings. Request to local db takes 3-8 ms.
I tracked that 99% of the execution time is taken up by this section.
@SpecialSauce
and the running time of the script is very random, then 300ms, as in all my other similar scripts, then 15000ms
*don't ping. Anyone
Then try
editReply
or check if its a network problem since the runtimes are different.
and do as Qjuh said by awaiting the deferUpdatethat isn't a reply
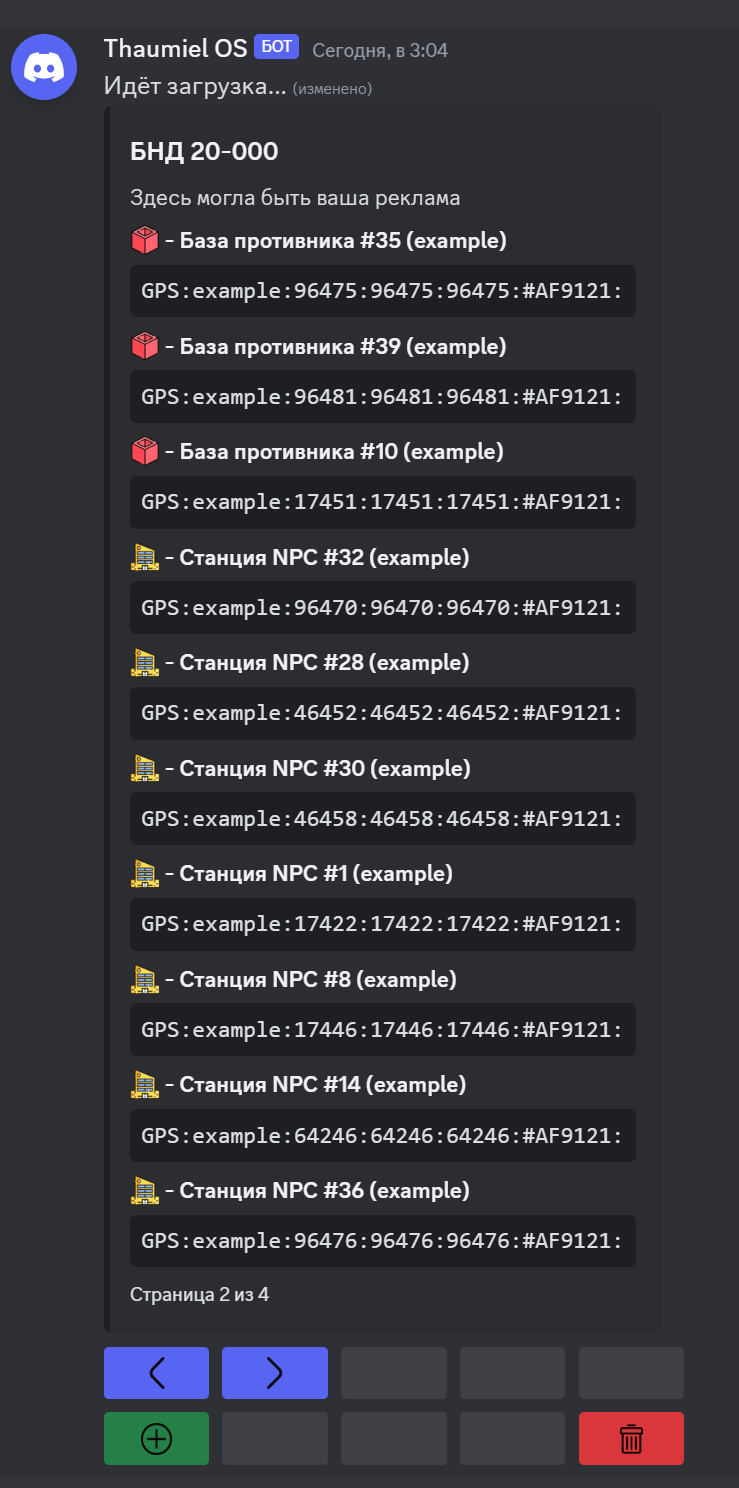
that is common message
Well, have you tried awaiting the
deferUpdate
? If that doesn't work then I dont believe this issue is related to d.jsSounds like a possible rate limit
Please add the following code to your code base outside of any other event listeners and provide the full log output relevant to your issue.
- Note: if you initialize your Client as
bot
or other identifiers you need to use these instead of client
- If the output is too long to post consider using a bin instead: gist | paste.gg | sourceb.in | hastebinIt may be a better option to respond to the interaction as recommended by Qjuh. Those endpoints probably have different rate limits.
Pastebin
log - Pastebin.com
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
it is advisable to avoid this, since it will require a complete restructuring of the database and script
What would need to be changed besides interaction.message.edit > interaction.editReply?
That message isn't a respons of interaction. That one is a static message
interaction.editReply throws an error, while I am using it
What error?
oh lol I used
interaction.message.editReply()
😄
now It works and works fast (maximum 500 ms)Just be aware of the possible race condition of not awaiting the deferUpdate() you could respond sooner than when that completes
Id probably just await it
but why in other my scripts (I use await there) this problem doesn't cause?
Pastebin
code - Pastebin.com
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
it works well
and I use that:
Thanks for help)
maybe