JS logic question
Why is the answer to this
20 30 10 20 200
and not 10 20 20 30 200
?
Would anyone mind explaining it to me?47 Replies
because
abc
has local variables that receive 10 and 20, respectively
and the function is the first thing to run
when it exits the function, the variables in that scope are unchanged, and that's why it goes back to 10, 20 and then 200So,
z = abc(x,y);
basically means that the function is being called, and not declared, right?right, because the function is defined before
and the function receives 2 arguments
if you remove the 2 arguments, the result will be 20, 30, 20, 30, 200
I still can't understand it fully 😭
well, inside the function
abc
, rename x
to tomato
and y
to potato
The naming is not what confuses me, is the calling order.
the calling order has no influence
everything inside the function is something different
It does 20 and 30, which are the logs of abc, then it does 10 and 20? but then it gives the 200
you're mixing the local variables in the function with the variables outside
they just happen to have the same name as the variables outside, but you can use any valid name and nothing will change
This is the correct answer based on the test I took
20 30 10 20 200
The correct orderyes
because javascript runs from top to bottom
Is this incorrect?
z = abc(x,y); // now it's declared, but not read
<-- no, this is the function being calledAnd what should be the outpuf of Z?
20, 30, 200?
and
z
receives the value returned by the function
200
but z
is the last thing to be shownconsole.log(x) -> console.log(y) -> console.log(z) in this case, this would be the order, correct?
yes
it goes from top to bottom
I mean, the way I understand it, is that it first calls Z, and it just returns the 2 logs inside the function, then you log X, then you log Y, then you log Z, which Z returns 200 (and I don't understand why it doesn't return the 2 logs inside)
no, z isn't a function
z is a variable
z = abc(x,y);
<-- the =
means "assignment"then why the heck does it show the 2 logs inside Z first
it doesn't
z contains a number, not a variable
z can't do anything
aha, and it goes back to my question
why is
20 30 10 20 200
the correct answerthat line should be written like this:
var z = abc(x,y);
and not
10 20 20 30 200
because `abc´ is called
but you just said it was an assingment 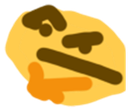
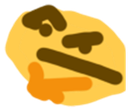
execution order: 🟥 🟦 🟪 🟧
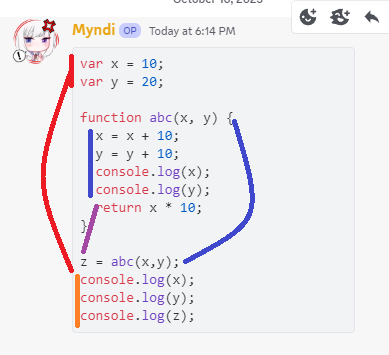
z = abc(x,y);
is 3 things in 1:
1- calls abc
2- defines a global variable z
3- assigns the result of calling abc(x,y)
into z
okay, the function by itself does nothing until being called, when Z is declared it also calls and runs the function, right?
no
z doesn't do anything
hang on
so, a long time ago, i wrote my own language, and i think the code will help you understand what's going on
the result of it is exactly what you explained, but the "code" is written in pure english
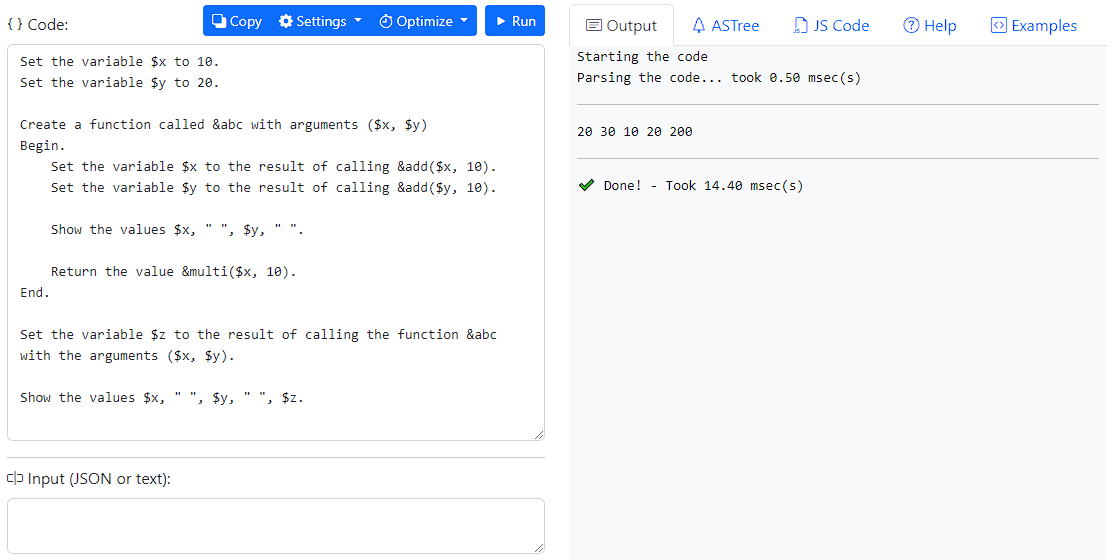
What I don't get is why the console log from inside the function is logged, before the function is called. That's what I don't understand
I get everything else
that's why I asked my order vs the answer's order
Set the variable $z to the result of calling the function &abc with the arguments ($x, $y).
<-- this
it calls the function THEN assigns the returned value
but the function is called first
and part of the function is to produce outputYeah, I assume you could just type abc(x,y)
but in this case you type abc(x,y) = z (to write it in another way)
I guess what throws me off is the return at the end
more like
abc(x, y) -> z
it returns 200, ends the function, but nothing happens with it
yup
because it's not being told to exist anywhere else
and when you assign it to Z, Z assumes the value of 200
but ignores the console.logs?
it doesn't ignore
or they get sent to the void?
think of a function as a completely new program
what happens with the console.log(z)
and that program receives stuff, and shows stuff
I know it's 200 because of the return, but what happpenedto the logs?
they are above the return, therefore, they are already executed
javascript runs from top to bottom
from the 1st line towards the last line
if the first line is
send cookies
, then it will send cookies
before doing anything elsethank you for your help ^_^
you're welcome