How to check if an element contains a class using JavaScript?
Hi all! Another question in regards to my stat module from before. I am trying to use JavaScript to check if the container contains the class "dark-bg" and then do something else. I have tried .matches() and classList.has() it keeps giving me an error. Any help would be grealy appreciated!
https://codepen.io/rnkwill/pen/OJrzroj
17 Replies
element.classList.contains(class)
^^ tried that before and it gives me this error

Chris's answer is correct. Seems like that error is because you tried to access an element that doesn't exist.
Is your querySelector correct?
In your code "statContainer" is an array so you will need to do a loop or define a specific selector to check.
(I'm on the mobile so I can't really see what is happening)
I see. I had to step away but will try running a loop to check and will report back.
I updated the code pen after running a loop in the function but now it keeps firing and not sure what can be causing that now.
It keeps saying it has the class but only 1 of the containers has the class
Any ideas anyone?
statContainer
is a NodeList, so you can't use matches
on it, only on individual DOM elementsI reverted back to classList.contains but when I console log it returns the first node item in the list 3x.
My code pen has been updated. The test class function behaves as it should but once I try to run the if else statement in the computedGradientForPercentage function it breaks. So it has something to do with that but I can’t quite figure out why.
https://codepen.io/rnkwill/pen/OJrzroj
the class
stat-value
doesn't exist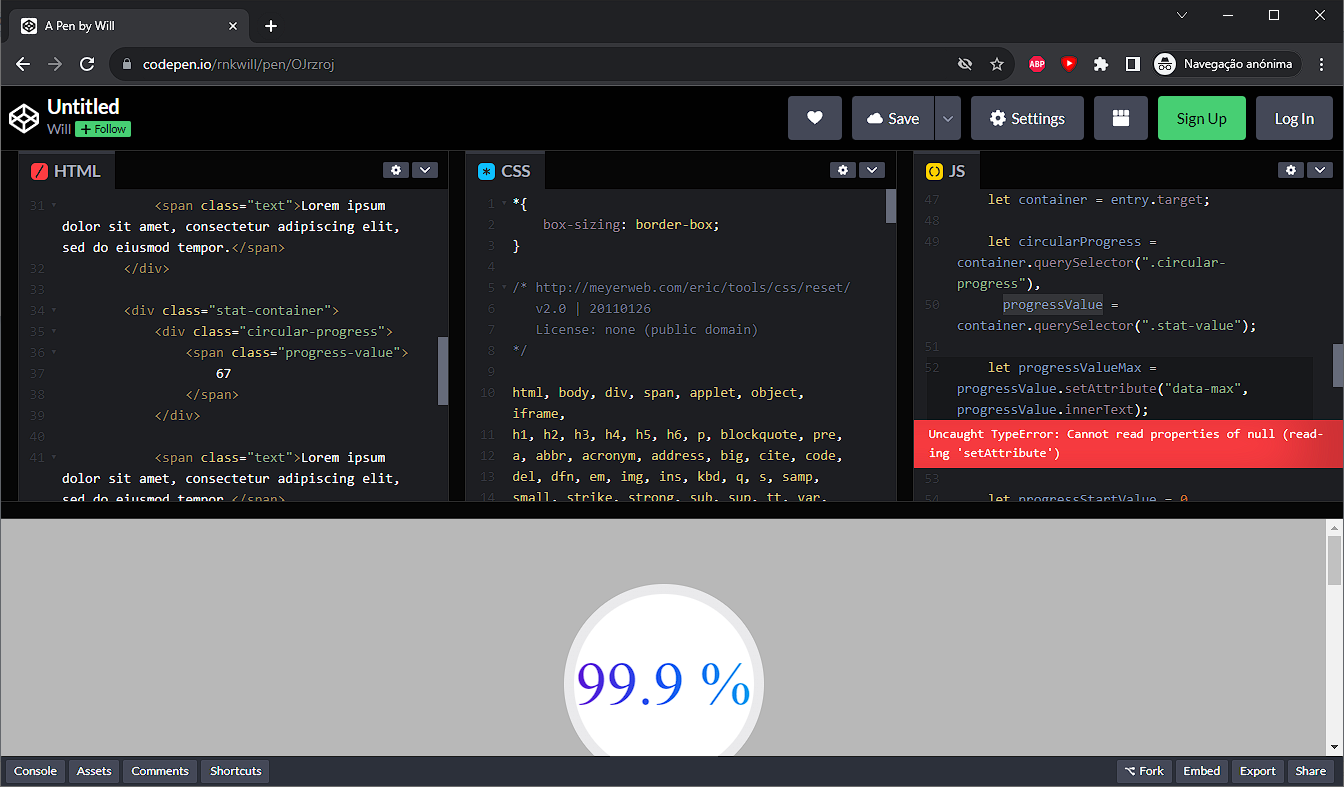
Ugh. Sorry I need to update the code pen some more. Been flipping through so many things. The code pen has been updated to reflect all recent updates! Thanks for calling that out @ἔρως
it seems to be working
i do see the numbers flipping
The number increase as they should. What needs to happen is if the div.stat-container has class dark-bg the circular progress bar turns white instead of grey. If see the first computedGradientForPercentage function it works as it should but if you comment that out and uncomment the second version of that function under it - it breaks.
ew
you're doing it in javascript
just use a css variable and update it
you're making a huge tangled mess with javascript and css
i think it's better if you refactor this to use css variables
you will have a lot more success
Your observer is already looping through each of the circles so you don't need the computeGradientForPercentage() to loop through them all again - this ends up overwriting things.
Try changing this line:
circularProgress.style.background = computeGradientForPercentage(progressStartValue);
to this so that it passes the current container within the "entries" loop:
circularProgress.style.background = computeGradientForPercentage(container,progressStartValue);
Then, update your computeGradientForPercentage()
function to accept the container argument and just check if it contains the "dark-bg" class something like this:
or, just rewrite this all to use css
and you update the variable
something like this:
then, you just do
element.style.setProperty('--percent', <value>)