Can't get 'uses' property on fetched invites
Trying to get all the invites from the guild
Invite objects with the 'uses' counter are expected to be received, but none of them have it at all
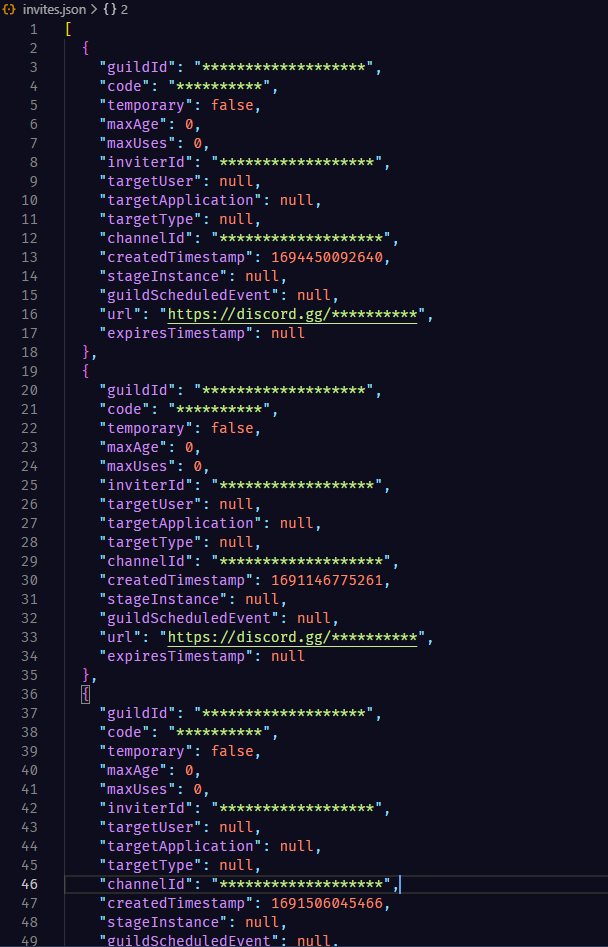
18 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffCan you log
inv
? (Also thats not how cache works)It is already on the screenshot, since it writed to the file for debugging purposes
Show your Client constructor
Its better to use
GatewayIntentBits
enumplanned
just copied from one old project, so already all intents on the hand
but this will not affect the topic of the question in any way
as temporary solution now I use
no I cant. there are no uses property in retrieved objects
they are in Invite class, but they are not in Invite objects, that I get from guild.invites.fetch()
check attachment
so only rest method is the solution?
but invites, that I get from rest request have both uses and maxUses properties even if maxUses is 0
sorry for answering so late
Is rest defined as
client.rest
?rest defined in client/index.ts as
Can you try using client.rest instead of rest?
same
zero difference between client.rest and REST()
if I log it without stringifying it appears in console as [object Object]
got it
oh, there it is
the thing is, I couldn't access uses previously, so I decided to console.log(JSON.stringify(invFetch))
and as uses wasn't there I thought that they are not exist in fetched invites
I've already forgotten and not commited the way I tried
@Qjuh tyvm, looks like I need to learn more about js objects 🤓