i need one interaction on select Replace the interaction 1
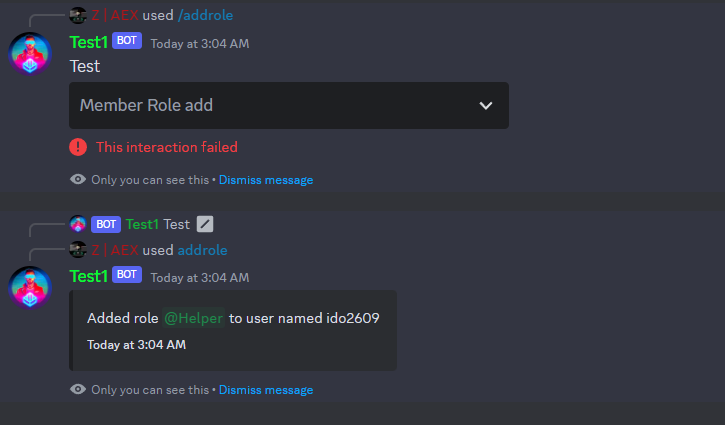
2 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!<Interaction>.followUp()
just sends a follow up message to an interaction
since you're not responding to the component interaction anywhere, I'd suggest using <ComponentInteraction>.update()
which properly responds to the interaction (so that you don't get the "This interaction failed" message) and updates the message the component is on